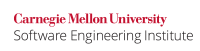
Functions should provide a consistent and easy to use interface for error checking. Complex interfaces are sometimes ignored by programmers, resulting in code that is not error checked. Inconsistent interfaces are frequently misused and difficult to use, resulting in lower quality code and higher development costs.
Noncompliant Code Example
The standard strncpy()
function does not guarantee that the resulting string is null terminated [[ISO/IEC 9899:1999]]. If there is no null character in the first n
characters of the source
array, the result may not be null terminated.
char *source; char a[NTBS_SIZE]; /* ... */ if (source) { errno_t err = strncpy(a, source, 5); if (err != 0) { /* Handle error */ } } else { /* handle NULL string condition */ }
Compliant Solution (strncpy_s()
)
The strncpy_s()
function copies up to n
characters from the source array to a destination array [[TR 24731]]. If no null character was copied from the source array, then the n
th position in the destination array is set to a null character, guaranteeing that the resulting string is null-terminated.
char *source; char a[NTBS_SIZE]; /* ... */ if (source) { errno_t err = strncpy_s(a, sizeof(a), source, 5); if (err != 0) { /* Handle error */ } } else { /* handle NULL string condition */ }
Risk Assessment
Failure to do so can result in type errors in the program.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
API07-C |
medium |
unlikely |
medium |
P2 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C++ Secure Coding Standard as API07-CPP. Enforce type safety.
References
[[ISO/IEC 9899:1999]] Section 7.21, "String handling <string.h>"
[[ISO/IEC PDTR 24772]] "CJM String Termination"
[[ISO/IEC TR 24731-1:2007]] Section 6.7.1.4, "The strncpy_s function"
01. Preprocessor (PRE) 02. Declarations and Initialization (DCL)