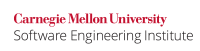
...
Non-Compliant Coding Example
Using the longjmp
function inside a signal handler is particularly dangerous, as it could call any part of your code.
Code Block | ||
---|---|---|
| ||
#include <setjmp.h> #include <signal.h> static jmp_buf env; void int_handler() { longjmp(env, 1); } int main() { char *foo; signal(SIGINT, int_handler); if(setjmp(env) == 0) { foo = malloc(15); foo = "Nothing yet."; } else { foo = "Signal caught."; } /* main loop which displays foo */ return 0; } |
Compliant Solution
Signal handlers should be as minimal as possible, only unconditionally setting a flag where appropriate, and returning.
Code Block | ||
---|---|---|
| ||
#include <setjmp.h>
#include <signal.h>
static jmp_buf env;
int interrupted = 0;
void int_handler() {
interrupted = 1;
}
int main() {
char *foo;
signal(SIGINT, int_handler);
foo = malloc(15);
foo = "Nothing yet.";
/* main loop which displays foo */
if(interrupt == 1) {
foo = "Signal caught.";
}
return 0;
}
|
Risk Assessment
Depending on the code, this could lead to any number of attacks, many of which could give root access. This is extremely dangerous. For an overview of actual issuessome software vulnerabilities, see Zalewski's signal article.
...