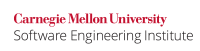
...
Code Block | ||
---|---|---|
| ||
#include <signal.h> #include <stdio.h> #include <stdlib.h> enum { MAXLINE = 1024 }; char *info = NULL; void log_message(void) { fprintf(stderr, info); } void handler(int signum) { log_message(); free(info); info = NULL; } int main(void) { if (signal(SIGINT, handler) == SIG_ERR) { /* Handle error */ } info = (char*)malloc(MAXLINE); if (info == NULL) { /* Handle Error */ } while (1) { /* Main loop program code */ log_message(); /* More program code */ } return 0; } |
This program's signal handler has four problems. The first is that it is unsafe to call the fprintf()
function from within a signal handler because the handler may be called when global data (such as stderr
) is in an inconsistent state. In general, it is not safe to invoke I/O functions within a signal handler.
...
The third problem is if SIGINT
occurs after the call to free()
, resulting in the memory referenced by info
being freed twice. This is a violation of rules MEM31-C. Free dynamically allocated memory exactly once and SIG31-C. Do not access or modify shared objects in signal handlers.
The fourth and final problem is that the signal handler reads the variable info
, which is not declared to be of type volatile sig_atomic_t
. This is a violation of rule SIG31-C. Do not access or modify shared objects in signal handlers.
Furthermore, there are problems in the {[main()}} function as well, such as the possibility that the signal handler might get invoked during the call to malloc()
in main()
.
Implementation Details
POSIX
...