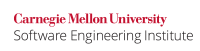
...
Code Block | ||
---|---|---|
| ||
static final int BUFFER = 512; // ... // external data source: filename FileInputStream fis = new FileInputStream(filename); ZipInputStream zis = new ZipInputStream(new BufferedInputStream(fis)); ZipEntry entry; while ((entry = zis.getNextEntry()) != null) { System.out.println("Extracting: " + entry); int count; byte data[] = new byte[BUFFER]; // write the files to the disk String name = validateFilename(entry.getName()); FileOutputStream fos = new FileOutputStream(entry.getName(name)); BufferedOutputStream dest = new BufferedOutputStream(fos, BUFFER); while ((count = zis.read(data, 0, BUFFER)) != -1) { dest.write(data, 0, count); } dest.flush(); dest.close(); zis.closeEntry(); } zis.close(); |
...
Code Block | ||
---|---|---|
| ||
static final int BUFFER = 512;
static final int TOOBIG = 0x6400000; // 100MB
// ...
// external data source: filename
FileInputStream fis = new FileInputStream(filename);
ZipInputStream zis = new ZipInputStream(new BufferedInputStream(fis));
ZipEntry entry;
try{
while ((entry = zis.getNextEntry()) != null) {
System.out.println("Extracting: " + entry);
int count;
byte data[] = new byte[BUFFER]; // write the files to the disk, but ensure that the file is not insanely big
int total = 0;
String name = validateFilename(entry.getName());
FileOutputStream fos = new FileOutputStream(name);
 BufferedOutputStream dest = new BufferedOutputStream(fos, BUFFER);
 while (total <= TOOBIG && (count = zis.read(data, 0, BUFFER)) != -1) {
   dest.write(data, 0, count);
   total += count;
 }
 dest.flush();
 dest.close();
zis.closeEntry();
if (total > TOOBIG){
throw new IllegalStateException("File being unzipped is huge."); }
}
} finally {
zis.close();
}
|
...
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="74bcba099b30a910-dba1b02f-4c1b40ec-ade1b7ea-f7bf8a0298cc71dfd0ebdac6"><ac:plain-text-body><![CDATA[ | [[Mahmoud 2002 | AA. References#Mahmoud 02]] | [Compressing and Decompressing Data Using Java APIs | http://java.sun.com/developer/technicalArticles/Programming/compression/] | ]]></ac:plain-text-body></ac:structured-macro> |
...