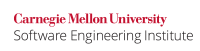
...
The Point
class is declared final
to prevent subclasses from overriding the clone()
method. This enables the class to be suitably used without any inadvertent modifications of the original object.
Noncompliant Code Example (Arrays)
This noncompliant code example uses a public static final
array, items
:
Code Block | ||
---|---|---|
| ||
public static final String[] items = {/* ... */};
|
Clients can trivially modify the contents of the array even though declaring the array reference to be final
prevents modification of the reference itself.
Compliant Solution (Index Getter)
This compliant solution makes the array private
and provides public methods to get individual items and array size.
Code Block | ||
---|---|---|
| ||
private static final String[] items = {/* ... */};
public static final String getItem(int index) {
return items[index];
}
public static final int getItemCount() {
return items.length;
}
|
Providing direct access to the array objects themselves is safe because String
is immutable.
Compliant Solution (Clone the Array)
This compliant solution defines a private
array and a public
method that returns a copy of the array:
Code Block | ||
---|---|---|
| ||
private static final String[] items = {/* ... */};
public static final String[] getItems() {
return items.clone();
}
|
Because a copy of the array is returned, the original array values cannot be modified by a client. Note that a manual deep copy could be required when dealing with arrays of objects. This generally happens when the objects do not export a clone()
method. Refer to OBJ06-J. Defensively copy mutable inputs and mutable internal components for more information.
As before, this method provides direct access to the array objects themselves, but this is safe because String
is immutable. If the array contained mutable objects, the getItems()
method could return an array of cloned objects instead.
Compliant Solution (Unmodifiable Wrappers)
This compliant solution declares a private
array from which a public
immutable list is constructed:
Code Block | ||
---|---|---|
| ||
private static final String[] items = { ... };
public static final List<String> itemsList =
Collections.unmodifiableList(Arrays.asList(items));
|
Neither the original array values nor the public
list can be modified by a client. For more details about unmodifiable wrappers, refer to OBJ56-J. Provide sensitive mutable classes with unmodifiable wrappers. This solution can also be used when the array contains mutable objects.
Applicability
Incorrectly assuming that final
references cause the contents of the referenced object to remain mutable can result in an attacker modifying an object believed to be immutable.
...