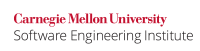
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h> #include <string.h> enum { N1 = 4096 }; void *func(size_t n2) { typedef int A[n2][N1]; A *array = malloc(sizeof(A)); if (!array) { /* Handle error */ return NULL; } for (size_t i = 0; i != n2; ++i) { memset(array[i], 0, N1 * sizeof(int)); } return array; } |
Furthermore, this code also violates ARR39-C. Do not add or subtract a scaled integer to a pointer, where array
is a pointer to the two-dimensional array, where it should really be a pointer to the latter dimension instead. This means that the memset()
call does out-of-bounds writes on all of its invocations except the first.
Compliant Solution (sizeof
)
This compliant solution prevents sizeof
wrapping by detecting the condition before it occurs and avoiding the subsequent computation when the condition is detected. The code also uses an additional typedef to fix the type of array
so that memset()
never writes past the two-dimensional array.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdint.h> #include <stdlib.h> #include <string.h> enum { N1 = 4096 }; void *func(size_t n2) { if (n2 > SIZE_MAX / (N1 * sizeof(int))) { /* Prevent sizeof wrapping */ return NULL; } typedef int A1[N1]; typedef A1 A[n2][N1]; AA1 *array = (A1*) malloc(sizeof(A)); if (!array) { /* Handle error */ return NULL; } for (size_t i = 0; i != n2; ++i) { memset(array[i], 0, N1 * sizeof(int)); } return array; } |
...