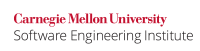
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> #include <stdlib.h> #include <string.h> char *get_filled_buffer(void) { char temp[32]; char *ret = NULL; char *end = ret; while (fgets(temp, sizeof(temp), stdin)) { size_t len = strlen(temp); const size_t full_size = end - ret + len; char *r_temp = realloc(ret, full_size + 1); /* NTBS */ if (r_temp == NULL) { break; } ret = r_temp; strcat(ret, temp); end = ret + full_size; if (feof(stdin) || temp[len-1] == '\n') { return ret; } } free(ret); return NULL; } |
Compliant Solution (POSIX getline()
)
The getline()
function was originally a GNU extension, but is now standard in POSIX.1-2008. It also fills a string with characters from an input stream. In this case, the program passes it a NULL pointer for a string, indicating that getline()
should allocate sufficient space for the string and the caller frees it later.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h>
void func(void) {
char* buf = NULL;
size_t dummy = 0;
if (getline(&buf, &dummy, stdin) == -1) {
/* handle error */
}
printf("The user input %s\n", buf);
free(buf);
} |
Risk Assessment
Incorrectly assuming a newline character is read by fgets()
or fgetws()
can result in data truncation.
...