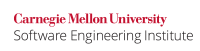
...
Code Block | ||
---|---|---|
| ||
String file = /* Provided by user */; InputStream in = null; try { in = new FileInputStream(file); // ... } finally { try { if (in !=null) { in.close();} } catch (IOException x) { // Handle error } } |
Noncompliant Code Example
...
This noncompliant code example uses the try
-with-resources statement from (introduced in Java SE 7) to open the file. The try
-with-resources statement guarantees the file's successful closure if an exception is thrown, but this code is subject to the same vulnerabilities as the previous example.
Code Block | ||
---|---|---|
| ||
String filename = /* Provided by user */; Path path = new File(filename).toPath(); try (InputStream in = Files.newInputStream(path)) { // Read file } catch (IOException x) { // Handle error } |
Noncompliant Code Example (
...
isRegularFile()
)
This noncompliant code example first checks that the file is a regular file (using the NIO.2 API) before opening it:
...
This test can still be circumvented by a symbolic link. By default, the readAttributes()
method follows symbolic links and reads the file attributes of the final target of the link. The result is that the program may reference a file other than the one intended.
Noncompliant Code Example (
...
NOFOLLOW_LINKS
)
This noncompliant code example checks the file by calling the readAttributes()
method with the NOFOLLOW_LINKS
link option to prevent the method from following symbolic links. This approach allows the detection of symbolic links because the isRegularFile()
check is carried out on the symbolic link file and not on the final target of the link.
...
This code is still vulnerable to a time-of-check, time-of-use (TOCTOU) race condition. For example, an attacker can replace the regular file with a file link or device file after the code has completed its checks but before it opens the file.
Noncompliant Code Example (POSIX
...
: Check-Use-Check)
This noncompliant code example performs the necessary checks and then opens the file. After opening the file, it performs a second check to make sure that the file has not been moved and that the file opened is the same file that was checked. This approach reduces the chance that an attacker has changed the file between checking and then opening the file. In both checks, the file's fileKey
attribute is examined. The fileKey
attribute serves as a unique key for identifying files and is more reliable than the path name as on indicator of the file's identity.
...
- The TOCTOU race condition still exists between the first check and open. During this race window, an attacker can replace the regular file with a symbolic link or other nonregular file. The second check detects this race condition but does not eliminate it.
- An attacker could subvert this code by letting the check operate on a regular file, substituting the nonregular file for the open, and then resubstituting the regular file to circumvent the second check. This vulnerability exists because Java lacks a mechanism to obtain file attributes from a file by any means other than the file name, and the binding of the file name to a file object is reasserted every time the file name is used in an operation. Consequently, an attacker can still swap a file for a nefarious file, such as a symbolic link.
- A system with hard links allows an attacker to construct a malicious file that is a hard link to a protected file. Hard links cannot be reliably detected by a program and can foil canonicalization attempts, which are prescribed by FIO16-J. Canonicalize path names before validating them.
Compliant Solution (POSIX
...
: Secure Directory)
Because of the potential for race conditions and the inherent accessibility of shared directories, files must be operated on only in secure directories. Because programs may run with reduced privileges and lack the facilities to construct a secure directory, a program may need to throw an exception if it determines that a given path name is not in a secure directory.
...