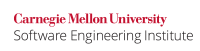
Avoid excessive stack allocations, particularly in situations where the growth of the stack can be controlled or influenced by an attacker. See INT04-C. Enforce limits on integer values originating from tainted sources for more information on preventing attacker-controlled integers from exhausting memory.
Noncompliant Code Example
The C standard includes Standard includes support for variable - length arrays (VLAs) [ISO/IEC 9899:2011]. If the array length is derived from an untrusted data source, an attacker can cause the process to perform an excessive allocation on the stack.
This noncompliant code example temporarily stores data read from a source file into a buffer. The buffer is allocated on the stack as a variable-length array a VLA of size bufsize
. If bufsize
can be controlled by a malicious user, this code can be exploited to cause a denial-of-service attack.:
Code Block | ||||
---|---|---|---|---|
| ||||
int copy_file(FILE *src, FILE *dst, size_t bufsize) { char buf[bufsize]; while (fgets(buf, bufsize, src)) { if (fputs(buf, dst) == EOF) { /* Handle error */ } } return 0; } |
The BSD extension function alloca()
behaves in a similar fashion to variable-length arraysVLAs; its use is not recommended [Loosemore 2007].
...
This compliant solution replaces the variable-length array the VLA with a call to malloc()
. If malloc()
fails, the return value can be checked to prevent the program from terminating abnormally.
Code Block | ||||
---|---|---|---|---|
| ||||
int copy_file(FILE *src, FILE *dst, size_t bufsize) { if (bufsize == 0) { /* Handle error */ } char *buf = (char *)malloc(bufsize); if (!buf) { /* return -1;Handle error */ } while (fgets(buf, bufsize, src)) { if (fputs(buf, dst) == EOF) { /* Handle error */ } } /* ... */ free(buf); return 0; } |
...
Recursion can also lead to large stack allocations. Recursive functions must ensure that they they do not exhaust the stack due to excessive stack as a result of excessive recursions.
This noncompliant implementation of the Fibonacci function uses recursion.:
Code Block | ||||
---|---|---|---|---|
| ||||
unsigned long fib1(unsigned int n) { if (n == 0) { return 0; } else if (n == 1 || n == 2) { return 1; } else { return fib1(n-1) + fib1(n-2); } } |
The required stack space needed grows exponentially The amount of stack space needed grows linearly with respect to the parameter n
. Large values of n
have been shown to cause abnormal program termination.
Compliant Solution
This implementation of the Fibonacci functions eliminates the use of recursion.:
Code Block | ||||
---|---|---|---|---|
| ||||
unsigned long fib2(unsigned int n) { if (n == 0) { return 0; } else if (n == 1 || n == 2) { return 1; } unsigned long prev = 1; unsigned long cur = 1; unsigned int i; for (i = 3; i <= n; i++) { unsigned long tmp = cur; cur = cur + prev; prev = tmp; } return cur; } |
...
Program stacks are frequently used for convenient temporary storage because allocated memory is automatically freed when the function returns. Generally, the operating system will grow grows the stack as needed. However, growing the stack can fail due to a fail because of a lack of memory or collision a collision with other allocated areas of the address space (depending on the architecture). When the stack is exhausted, the operating system can terminate the program abnormally. This behavior can be exploited, and an attacker can cause a denial-of-service attack in situations where the attacker can attack if he or she can control or influence the amount of stack memory allocated.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MEM05-C |
Medium |
Likely |
Medium |
P12 |
L1 |
Automated Detection
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
CodeSonar |
| IO.TAINT.SIZE MISC.MEM.SIZE.BAD | Tainted Allocation Size Unreasonable Size Argument | ||||||
| STACK_USE | Can help detect single stack allocations that are dangerously large, although it will not detect excessive stack use resulting from recursion | |||||||
Helix QAC |
| C1051, C1520, C3670 | |||||||
Klocwork |
| MISRA.FUNC. |
1520
3670
Related Vulnerabilities
RECUR | |||||||||
LDRA tool suite |
| 44 S | Enhanced Enforcement | ||||||
Parasoft C/C++test |
| CERT_C-MEM05-a | Do not use recursion | ||||||
PC-lint Plus |
| 9035, 9070 | Partially supported: reports use of variable length arrays and recursion | ||||||
Polyspace Bug Finder |
| Checks for:
Rec. partially covered. | |||||||
PVS-Studio |
| V505 |
Related Vulnerabilities
Stack overflow has been implicated in Toyota unintended acceleration cases, where Camry and other Toyota vehicles accelerated unexpectedly. Michael Barr testified at the trial that a stack overflow could corrupt the critical variables of the operating system, because they were located in memory adjacent to the top of the stack [Samek 2014].
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
...
...
...
TR 24772:2013 | Recursion [GDL] |
MISRA C:2012 | Rule 17.2 (required) |
ISO/IEC TR 24772 "GDL Recursion"
...
Bibliography
...
...
...
Samek 2014] | Are We Shooting Ourselves in the Foot with Stack Overflow? Monday, February 17th, 2014 by Miro Samek |
[Seacord 2013] | Chapter 4, "Dynamic Memory Management |
...
" |
...