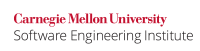
...
Code Block | ||
---|---|---|
| ||
/* ... */ int fd = open(file_name, O_CREAT | O_WRONLY, file_access_permissions); if (fd == -1){ /* Handle Error */ } /* ... */ |
Non-Compliant Code Example (argument validation)
Using user-defined functions to calculate the amount of memory to allocate is a common practice that may sometimes be necessary. However, if the function used to calculate the size parameter is flawed, then an incorrect size argument will be supplied to the allocation routine.
This following non-compliant code reads user-supplied data from standard input, returning the number of characters read.
Code Block | ||
---|---|---|
| ||
#include <stdlib.h>
#include <stdio.h>
enum { MAXLINE = 1000 };
size_t calc() {
char line[MAXLINE], c;
size_t size = 0;
while ( (c = getchar()) != EOF && c != '\n') {
line[size] = c;
size++;
if (size >= MAXLINE)
break;
}
return size;
}
int main(void) {
char * line = malloc(calc());
printf("%d\n", size);
}
|
However, if no characters are entered, calc()
will return 0
. Because there is no validation on the result of calc()
, a malloc(0)
could occur, which could lead to a buffer overflow.
Compliant Solution (argument validation)
In this compliant solution, the result of calc()
is not supplied directly to malloc()
. Instead, the result of calc()
is stored in the variable size
and checked for the exceptional condition of being 0
. This modification reduces the complexity of the line of code that calls malloc()
and adds an additional layer of validation, thus reducing the chances of error.
Code Block | ||
---|---|---|
| ||
#include <stdlib.h>
#include <stdio.h>
enum { MAXLINE = 1000 };
size_t calc() {
char line[MAXLINE], c;
size_t size = 0;
while ( (c = getchar()) != EOF && c != '\n') {
line[size] = c;
size++;
if (size >= MAXLINE)
break;
}
return size;
}
int main(void) {
size_t size = calc();
if (size > 0) {
char * line = malloc(size)
printf("%d\n", size);
}
}
|
Risk Assessment
Calling a function with incorrect arguments can result in unexpected or unintended program behavior.
...