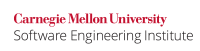
...
Code Block |
---|
int rename(char const *old_file, char const *new_file); |
If the file referenced by new_file
exists prior to calling rename()
, the behavior is implementation-defined. On POSIX systems, the file is removed. On Windows systems, the rename()
fails.
...
Occasionally, a programmer might want to implements a behavior other than the implementation-defined behavior for rename()
on that platform. If the desired behavior is to ensure that any file referenced by new_file
is removed, Windows programmers must write additional code.
...
If the intent of the programmer is to remove the file referenced by new_file
if it exists prior to calling rename()
, this code example is non-compliant on Windows platforms because it will fail, returns a nonzero value, and sets errno
to EACCES
.
Code Block | ||
---|---|---|
| ||
char const *old_file = /* ... */; char const *new_file = /* ... */; if (rename(old_file, new_file) != 0) { /* Handle Error */ } |
...
On Windows systems, it is necessary to remove the file referenced by new_file
before calling rename()
, if you want the file to be overwritten and the rename()
operation to succeed.
Code Block | ||
---|---|---|
| ||
char const *old_file = /* ... */; char const *new_file = /* ... */; if (remove(new_file) != 0) { /* Handle error condition */ } if (rename(old_file, new_file) != 0) { /* Handle error condition */ } |
...
On POSIX systems, if the file referenced by new_file
exists prior to calling rename()
, the file is removed.
Code Block | ||
---|---|---|
| ||
char const *old_file = /* ... */; char const *new_file = /* ... */; if (rename(old_file, new_file) != 0) { /* Handle error condition */ } |
...
If the desired behavior is to ensure that any file referenced by new_file
is not removed, POSIX programmers must write additional code.
...
If the intent of the programmer is to not remove the file referenced by new_file
if it exists prior to calling rename()
, this code example is non-compliant because on POSIX systems the file is removed.
Code Block | ||
---|---|---|
| ||
char const *old_file = /* ... */; char const *new_file = /* ... */; if (rename(old_file, new_file) != 0) { /* Handle Error */ } |
...
Wiki Markup |
---|
If the intent of the programmer is to not remove the file referenced by {{new_file}} if it exists prior to calling {{rename()}}, the POSIX {{access()}} function which can check for the existence of a file explicitly \[[Open Group 04|AA. C References#Open Group 04]\]. This compliant solution only renames the file referenced by {{old_file}} if the file referenced by {{new_file}} does not exist. |
Code Block | ||
---|---|---|
| ||
char const *old_file = /* ... */; char const *new_file = /* ... */; if (access(new_file, F_OK) != 0) { if (rename(old_file, new_file) != 0) { /* Handle error condition */ } } else { /* Handle error condition */ } |
...
Consequently, it is unnecessary to explicitly check for the existence of the file referenced by new_file
before calling rename()
.
Code Block | ||
---|---|---|
| ||
char const *old_file = /* ... */; char const *new_file = /* ... */; if (rename(old_file, new_file) != 0) { /* Handle Error */ } |
...
This compliant solution ensures that any file referenced by new_file
is portably removed.
Code Block | ||
---|---|---|
| ||
char const *old_file = /* ... */; char const *new_file = /* ... */; if (remove(new_file) != 0) { /* Handle error condition */ } if (rename(old_file, new_file) != 0) { /* Handle error condition */ } |
...
This compliant solution only renames the file referenced by old_file
if the file referenced by new_file
does not exist.
Code Block | ||
---|---|---|
| ||
char const *old_file = /* ... */; char const *new_file = /* ... */; if (access(new_file, F_OK) != 0) { if (rename(old_file, new_file) != 0) { /* Handle error condition */ } } else { /* Handle error condition */ } |
...