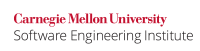
...
In general, it is not safe to invoke I/O functions from within signal handlers. Programmers should ensure a function is included in the list of an implementation's asynchronous-safe functions for all implementations the code will run on before using them in signal handlers.
Noncompliant Code Example
In this noncompliant example, the C standard library functions fprintf()
and free()
are called from the signal handler via the function log_message()
. Neither function is asynchronous-safe.
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <signal.h> #include <stdlib.h> enum { MAXLINE = 1024 }; volatile sig_atomic_t eflag = 0; void handler(int signum) { eflag = 1; } void log_message(char *info1, char *info2) { static char *buf = NULL; static size_t bufsize; char buf0[MAXLINE]; if (buf == NULL) { buf = buf0; bufsize = sizeof(buf0); } /* * Try to fit a message into buf, else reallocate * it on the heap and then log the message. */ if (buf == buf0) { buf = NULL; } } int main(void) { if (signal(SIGINT, handler) == SIG_ERR) { /* Handle error */ } char *info1; char *info2; /* info1 and info2 are set by user input here */ while (!eflag) { /* Main loop program code */ log_message(info1, info2); /* More program code */ } log_message(info1, info2); return 0; } |
Noncompliant Code Example (raise()
)
In this noncompliant code example, the int_handler()
function is used to carry out tasks specific to SIGINT
and then raises SIGTERM
. However, there is a nested call to the raise()
function, which is undefined behavior.
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <signal.h> #include <stdlib.h> void term_handler(int signum) { /* SIGTERM handler */ } void int_handler(int signum) { /* SIGINT handler */ /* Pass control to the SIGTERM handler */ term_handler(SIGTERM); } int main(void) { if (signal(SIGTERM, term_handler) == SIG_ERR) { /* Handle error */ } if (signal(SIGINT, int_handler) == SIG_ERR) { /* Handle error */ } /* Program code */ if (raise(SIGINT) != 0) { /* Handle error */ } /* More code */ return EXIT_SUCCESS; } |
Implementation Details
POSIX
The following table from the POSIX standard [IEEE Std 1003.1:2013] defines a set of functions that are asynchronous-signal-safe. Applications may invoke these functions, without restriction, from a signal handler.
Asynchronous-Signal-Safe Functions
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
...
See also undefined behavior 131.
OpenBSD
The OpenBSD signal()
manual page lists a few additional functions that are asynchronous-safe in OpenBSD but "probably not on other systems" [OpenBSD], including snprintf()
, vsnprintf()
, and syslog_r()
(but only when the syslog_data struct
is initialized as a local variable).
...
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Compass/ROSE | Can detect violations of the rule for single-file programs | ||||||||
| 88 D, 89 D | Partially implemented | |||||||
|
|
|
Related Vulnerabilities
For an overview of software vulnerabilities resulting from improper signal handling, see Michal Zalewski's paper "Delivering Signals for Fun and Profit" [Zalewski 2001].
...
[C99 Rationale 2003] | Subclause 5.2.3, "Signals and Interrupts" Subclause 7.14.1.1, "The signal Function" |
[Dowd 2006] | Chapter 13, "Synchronization and State" |
[Greenman 1997] | |
[IEEE Std 1003.1:2013] | XSH, System Interfaces, longjmp XSH, System Interfaces, raise |
[ISO/IEC 9899:2011] | 7.14.1.1, "The signal Function" |
[OpenBSD] | signal() Man Page |
[VU #834865] | |
[Zalewski 2001] | "Delivering Signals for Fun and Profit" |
...
adjust column widths