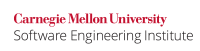
...
Code Block | ||||
---|---|---|---|---|
| ||||
void func(unsigned int ui_a, unsigned int ui_b) {
unsigned int usum = ui_a + ui_b;
/* ... */
} |
Compliant Solution (Precondition Test)
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <limits.h>
void func(unsigned int ui_a, unsigned int ui_b) {
unsigned int usum;
if (UINT_MAX - ui_a < ui_b) {
/* Handle error condition */
} else {
usum = ui_a + ui_b;
}
/* ... */
} |
Compliant Solution (Postcondition Test)
...
Subtraction is between two operands of arithmetic type, between two pointers to qualified or unqualified versions of compatible object types, or between a pointer to an object type and an integer type. (This rule only applies to subtraction between two operands of arithmetic type. See ARR36-C. Do not subtract or compare two pointers that do not refer to the same array, ARR37-C. Do not add or subtract an integer to a pointer to a non-array object, and ARR30-C. Do not form or use out of bounds pointers or array subscripts for information about pointer subtraction.)
Decrementing is equivalent to subtracting 1.
...
Code Block | ||||
---|---|---|---|---|
| ||||
void func(unsigned int ui_a, unsigned int ui_b) {
unsigned int udiff = ui_a - ui_b;
/* ... */
} |
Compliant Solution (Precondition Test)
...
Code Block | ||||
---|---|---|---|---|
| ||||
void func(unsigned int ui_a, unsigned int ui_b) {
unsigned int udiff;
if (ui_a < ui_b){
/* Handle error condition */
} else {
udiff = ui_a - ui_b;
}
/* ... */
} |
Compliant Solution (Postcondition Test)
...
Code Block | ||||
---|---|---|---|---|
| ||||
void func(unsigned int ui_a, unsigned int ui_b) {
unsigned int udiff = ui_a - ui_b;
if (udiff > ui_a) {
/* Handle error condition */
}
/* ... */
} |
Anchor | ||||
---|---|---|---|---|
|
...
The C Standard defines arithmetic on atomic integer types as read-modify-write operations with the same representation as nonatomic non-atomic integer types. As a result, wrapping of atomic unsigned integers is identical to nonatomic non-atomic unsigned integers and should also be prevented or detected.
This section includes an example only for the addition of atomic integer types. For other operations, you can use tests similar to the precondition tests for nonatomic non-atomic integer types.
Noncompliant Code Example
...
INT30-EX1. Unsigned integers can exhibit modulo behavior (wrapping) only when this behavior is necessary for the proper execution of the program. It is recommended that the variable declaration be clearly commented as supporting modulo behavior and that each operation on that integer also be clearly commented as supporting modulo behavior.
...
- Operations on two compile-time constants
- Operations on a variable and 0 (except division by 0, of course)
- Subtracting any variable from its type's maximum; for instanceexample, any
unsigned int
may safely be subtracted fromUINT_MAX
- Multiplying any variable by 1
- Division, as long as the divisor is nonzero
- Right-shifting any type maximum by any number smaller than the type size; for instance,
UINT_MAX >> x
is valid as long as0 <= x < 32
(assuming that the size ofunsigned int
is 32 bits) - Left-shifting 1 by any number smaller than the type size
...