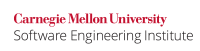
According to C99, Section 6.2.5, "Types":
A computation involving unsigned operands can never overflow, because a result that cannot be represented by the resulting unsigned integer type is reduced modulo the number that is one greater than the largest value that can be represented by the resulting type.
This behavior is more informally referred to as unsigned integer wrapping. Unsigned integer operations can wrap if the resulting value cannot be represented by the underlying representation of the integer. The following table indicates which operators can result in wrapping
...
:
Operator | Wrap |
| Operator | Wrap |
| Operator | Wrap |
| Operator | Wrap |
---|---|---|---|---|---|---|---|---|---|---|
yes |
| yes |
| << | yes |
| < | no | ||
yes |
| yes |
| >> | no |
| > | no | ||
yes |
| /= | no |
| & | no |
| >= | no | |
/ | no |
| %= | no |
| | | no |
| <= | no |
% | no |
| yes |
| ^ | no |
| == | no | |
++ | yes |
| >>= | no |
| ~ | no |
| != | no |
-- | yes |
| &= | no |
| ! | no |
| && | no |
= | no |
| |= | no |
| un + | no |
| || | no |
yes |
| ^= | no |
| un - | yes |
| ?: | no |
...
The following sections examine specific operations that are susceptible to unsigned integer wrap. When operating on small integer types (smaller than int
), integer promotions are applied. The usual arithmetic conversions may also be applied to (implicitly) convert operands to equivalent types before arithmetic operations are performed. Make sure you understand integer conversion rules before trying to implement secure arithmetic operations. (see See guideline INT02-C. Understand integer conversion rules.).
Integer values must not be allowed to wrap if they are used in any of the following ways:
...
Addition is between two operands of arithmetic type or between a pointer to an object type and an integer type. (see See guidelines ARR37-C. Do not add or subtract an integer to a pointer to a non-array object and ARR38-C. Do not add or subtract an integer to a pointer if the resulting value does not refer to a valid array element for rules about adding a pointer to an integer.) . Incrementing is equivalent to adding one.
...
Code Block | ||
---|---|---|
| ||
unsigned int ui1, ui2, usum; /* Initialize ui1 and ui2 */ usum = ui1 + ui2; |
Compliant Solution (Pre-
...
Condition Test)
This compliant solution performs a pre-condition test of the operands of the addition to guarantee there is no possibility of unsigned wrap.
Code Block | ||
---|---|---|
| ||
unsigned int ui1, ui2, usum; /* Initialize ui1 and ui2 */ if (UINT_MAX - ui1 < ui2) { /* handle error condition */ } else { usum = ui1 + ui2; } |
Compliant Solution (Post-
...
Condition Test)
This compliant solution performs a post-condition test to ensure that the result of the unsigned addition operation usum
is not less than either of the operands.
...
Subtraction is between two operands of arithmetic type, two pointers to qualified or unqualified versions of compatible object types, or between a pointer to an object type and an integer type. See guidelines ARR36-C. Do not subtract or compare two pointers that do not refer to the same array, ARR37-C. Do not add or subtract an integer to a pointer to a non-array object, and ARR38-C. Do not add or subtract an integer to a pointer if the resulting value does not refer to a valid array element for rules about pointer subtraction. Decrementing is equivalent to subtracting one.
...
Code Block | ||
---|---|---|
| ||
unsigned int ui1, ui2, udiff; /* Initialize ui1 and ui2 */ udiff = ui1 - ui2; |
Compliant Solution (Pre-
...
Condition Test)
This compliant solution performs a pre-condition test of the unsigned operands of the subtraction operation to guarantee there is no possibility of unsigned wrap.
Code Block | ||
---|---|---|
| ||
unsigned int ui1, ui2, udiff; /* Initialize ui1 and ui2 */ if (ui1 < ui2){ /* handle error condition */ } else { udiff = ui1 - ui2; } |
Compliant Solution (Post-
...
Condition Test)
This compliant solution performs a post-condition test that the result of the unsigned subtraction operation udiff
is not greater than the minuend.
...
Wiki Markup |
---|
The Mozilla Scalable Vector Graphics (SVG) viewer contains a heap buffer overflow vulnerability resulting from an unsigned integer wrap during the multiplication of the {{signed int}} value {{pen->num_vertices}} and the {{size_t}} value {{sizeof(cairo_pen_vertex_t)}} \[[VU#551436|AA. Bibliography#VU551436]\]. The {{signed int}} operand is converted to {{size_t}} prior to the multiplication operation (see [INT02-C. Understand integer conversion rules|INT02-C. Understand integer conversion rules]), so that the multiplication takes place between two {{size_t}} integers, which are unsigned. (See guideline [INT02-C. Understand integer conversion rules].) |
Code Block | ||
---|---|---|
| ||
pen->num_vertices = _cairo_pen_vertices_needed(
gstate->tolerance, radius, &gstate->ctm
);
pen->vertices = malloc(
pen->num_vertices * sizeof(cairo_pen_vertex_t)
);
|
...
This compliant solution tests the operands of the multiplication to guarantee that there is no unsigned integer wrap.
Code Block | ||
---|---|---|
| ||
pen->num_vertices = _cairo_pen_vertices_needed(
gstate->tolerance, radius, &gstate->ctm
);
if (pen->num_vertices > SIZE_MAX/sizeof(cairo_pen_vertex_t)) {
/* handle error condition */
}
pen->vertices = malloc(
pen->num_vertices * sizeof(cairo_pen_vertex_t)
);
|
...
- Operations on two compile-time constants
- Operations on a variable and 0 (except divison division by 0, of course)
- Subtracting any variable from its type's maximum. For instance, any
unsigned int
may safely be subtracted fromUINT_MAX
. - Multiplying any variable by 1
- Division, as long as the divisor is nonzero.
- Right-shifting any type maximum by any number smaller than the type size. For instance,
UINT_MAX >> x
is valid as long asx < sizeof(unsigned int)
. - Left-shifting 1 by any number smaller than the type size.
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
INT30-C | high | likely | high | P9 | L2 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
|
...
|
|
|
...
|
|
|
|
...
|
...
Related Vulnerabilities
Wiki Markup |
---|
[CVE-2009-1385|http://web.nvd.nist.gov/view/vuln/detail?vulnId=CVE-2009-1385] results from a violation of this rule. The value performs an unchecked subtraction on the {{length}} of a buffer, and then adds that many bytes of data to another buffer \[[xorl 2009|http://xorl.wordpress.com/2009/06/10/cve-2009-1385-linux-kernel-e1000-integer-underflow/]\]. This can cause a buffer overflow, which allows an attacker to execute arbitrary code. |
Wiki Markup |
---|
A Linux kernel vmsplice [exploit|BB. Definitions#exploit], described at \[[Wojtczuk 082008|AA. Bibliography#Wojtczuk 08]\], documents a vulnerability and exploit arising from a buffer overflow (caused by unsigned integer wrapping). |
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
Related Guidelines
This rule appears in the C++ Secure Coding Standard as : INT30-CPP. Ensure that unsigned integer operations do not wrap.
Bibliography
Wiki Markup |
---|
\[[Dowd 062006|AA. Bibliography#Dowd 06]\] Chapter 6, "C Language Issues" (Arithmetic Boundary Conditions, pp. 211-223) \[[ISO/IEC 9899:1999|AA. Bibliography#ISO/IEC 9899-1999]\] Section 6.2.5, "Types," Section 6.5, "Expressions," and Section 7.10, "Sizes of integer types {{<limits.h>}}" \[[ISO/IEC PDTR 24772|AA. Bibliography#ISO/IEC PDTR 24772]\] "XYY Wrap-around Error" \[[MITRE 072007|AA. Bibliography#MITRE 07]\] [CWE ID 190|http://cwe.mitre.org/data/definitions/190.html], "Integer Overflow (Wrap or Wraparound)" \[[Seacord 052005|AA. Bibliography#Seacord 05]\] Chapter 5, "Integers" \[[Viega 052005|AA. Bibliography#Viega 05]\] Section 5.2.7, "Integer overflow" \[[VU#551436|AA. Bibliography#VU551436]\] \[[Warren 022002|AA. Bibliography#Warren 02]\] Chapter 2, "Basics" \[[Wojtczuk 082008|AA. Bibliography#Wojtczuk 08]\] \[[xorl 2009|AA. Bibliography#xorl 2009]\] ["CVE-2009-1385: Linux kernel E1000 Integer Underflow"|http://xorl.wordpress.com/2009/06/10/cve-2009-1385-linux-kernel-e1000-integer-underflow/] |
...