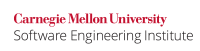
...
Code Block | ||
---|---|---|
| ||
#ifndef MY_HEADER_H #define MY_HEADER_H /* contents of <my_header.h> */ #endif /* MY_HEADER_H */ |
Noncompliant Code Example (Global Variable)
In this example, a variable beginning with an underscore is defined with implicit global scope.
Code Block | ||
---|---|---|
| ||
size_t _limit = 100; unsigned int getValue(unsigned int count){ size_t i; unsigned int result = 0; for(i = 0; i < _limit; i++){ result++; if(i == count){ break; } } } |
...
Noncompliant Code Example (Static Variable)
In this compliant solutionexample, the variable is declared as static and, hence, has file scope.
This code might have been safe if the C file containing it includes no header files. However, it requires stdlib.h
in order to define size_t
. And loading any standard header files will introduce a potential name clash. Consequently it is not safe to prepend any identifier with an underline, even if its usage is confined to a single file.
Code Block | ||
---|---|---|
| ||
static size_t _limit = 100; unsigned int getValue(unsigned int count){ size_t i; unsigned int result = 0; for(i = 0; i < _limit; i++){ result++; if(i == count){ break; } } } |
Compliant
...
Solution
...
(Global Variable)
In this compliant solution, the variable name does not begin with an underscore and, hence, is not reserved.
Code Block | ||
---|---|---|
| ||
size_t limit = 100; unsigned int getValue(unsigned int count){ size_t i; unsigned int result = 0; for(i = 0; i < limit; i++){ result++; if(i == count){ break; } } } |
Noncompliant Code Example
Identifiers with external linkage include setjmp
, errno
, math_errhandling
, and va_end
.
In the example, errno
is defined. The errno
value set by the function open()
would not be accessible to the program because its definition is suppressed. For information regarding redefining errno
, see ERR31-C. Don't redefine errno.
...