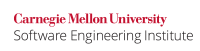
...
Code Block | ||
---|---|---|
| ||
int main(int argc, char** argv) { try { Object object; // do useful work return 0; // object gets destroyed here } catch (...) { throw; } } |
Non-Compliant Code Example (throw()
Declaration)
A function that declares exception specifications must include every exception that might be thrown during its invocation. If an exception is thrown that is not included in its specification, control automatically reverts to std::unexpected()
, which does not return.
In the following code example, the function f()
claims to throw exception1
but actually throws exception2
. Consequently control flow is diverted to std::unexpected
, and the toplevel catch
clause may not be invoked. (It is not invoked on Linux with G++ 4.3).
Code Block | ||
---|---|---|
| ||
using namespace std;
class exception1 : public exception {};
class exception2 : public exception {};
void f(void) throw( exception1) {
// ...
throw (exception2());
}
int main() {
try {
f();
return 0;
} catch (...) {
cerr << "F called" << endl;
}
return -1;
}
|
Compliant Solution (throw()
Declaration)
The following code example declares the same exception it actually throws
Code Block | ||
---|---|---|
| ||
using namespace std;
class exception1 : public exception {};
class exception2 : public exception {};
void f(void) throw( exception1) {
// ...
throw (exception1());
}
int main() {
try {
f();
return 0;
} catch (...) {
cerr << "F called" << endl;
}
return -1;
}
|
Risk Assessment
Failing to handle exceptions can lead to resources not being freed, closed, etc.
...