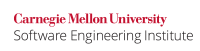
...
If no matching handler is found in a program, the function
std::terminate()
is called; whether or not the stack is unwound before this call tostd::terminate()
is implementation-defined (15.5.1).
...
In this code example, all exceptions are caught, allowing normal termination, even in the face of unexpected errors (albeit with an exit status indicating that an error occurred).
Code Block | ||
---|---|---|
| ||
int main(int argc, char** argv) { Object object; boolint errorexit_status = falseEXIT_SUCCESS; try { // do useful work } catch (...) { errorexit_status = trueEXIT_FAILURE; } return error ? -1 : 0exit_status; // object gets destroyed here } |
...
An alternative is to wrap all of main()
's functionality inside a try-catch block and catch and handle exceptions by exiting with a status indicating an error to the invoking process.
Code Block | ||
---|---|---|
| ||
int main(int argc, char** argv) { try { Object object; // do useful work return 0; // object gets destroyed here } catch (...) { throwexit(EXIT_FAILURE); } } |
Non-Compliant Code Example (throw()
Declaration)
A function that declares exception specifications must include every list all unrelated exception classes that might be thrown during its invocation. If an exception is thrown that is not included related to any of those listed in its exception specification, control automatically reverts to std::unexpected()
, which does not return.
...
Code Block | ||
---|---|---|
| ||
using namespace std; class exception1 : public exception {}; class exception2 : public exception {}; void f(void) throw( exception1) { // ... throw (exception2()); } int main() { try { f(); return 0; } catch (...) { cerr << "F called" << endl; } return -1EXIT_FAILURE; } |
Compliant Solution (throw()
Declaration)
...
Code Block | ||
---|---|---|
| ||
using namespace std; class exception1 : public exception {}; class exception2 : public exception {}; void f(void) throw( exception1) { // ... throw (exception1()); } int main() { try { f(); return 0; } catch (...) { cerr << "F called" << endl; } return -1EXIT_FAILURE; } |
Risk Assessment
Failing to handle exceptions can lead to resources not being freed, closed, etc.
...