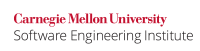
According to [12to The Java Language Specification (JLS), §12.4, "Initialization of Classes and Interfaces" |http://java.sun.com/docs/books/jls/third_edition/html/execution.html#12.4] of the _Java Language Specification_ \[ [JLS 2005|AA. Bibliography#JLS 05]\]]: Wiki Markup
Initialization of a class consists of executing its
static
initializers and the initializers forstatic
fields (class variables) declared in the class.
Therefore, the presence of a static field triggers the initialization of a class. However, the initializer of a static field could depend on the initialization of another class, possibly creating an initialization cycle.
The JLS also states in §8 In other words, the presence of a {{static}} field triggers the initialization of a class. However, a static field could depend on the initialization of a class, possibly creating an initialization cycle. The _Java Language Specification_ also states in [8.3.2.1, "Initializers for Class Variables" |http://java.sun.com/docs/books/jls/third_edition/html/classes.html#8.3.2.1] \ [[JLS 2005|AA. Bibliography#JLS 05]\]]: Wiki Markup
At ...at run time,
static
variables that arefinal
and that are initialized with compile-time constant values are initialized first.
This statement can be misleading because it is inapplicable does not apply to instances that use values of static final fields that are initialized at a later stage. Declaring a field to be static final is insufficient to guarantee that it is fully initialized before being read.
Programs in general should—and security-sensitive programs must—eliminate all class initialization cycles.
Noncompliant Code Example (
...
Intraclass Cycle)
This noncompliant code example contains an intra-class intraclass initialization cycle. :
Code Block | ||
---|---|---|
| ||
public class Cycle { private final int balance; private static final Cycle c = new Cycle(); private static final int deposit = (int) (Math.random() * 100); // Random deposit public Cycle() { balance = deposit - 10; // Subtract processing fee } public static void main(String[] args) { System.out.println("The account balance is: " + c.balance); } } |
The Cycle()
class declares a private static final
class variable, which is initialized to a new instance of the Cycle()
class. Static initializers are guaranteed to be invoked once before the first use of a static class member or the first invocation of a constructor.
The programmer's intent is to calculate the account balance by subtracting the processing fee from the deposited amount. However, the initialization of the c
class variable happens before the runtime initialization of the deposit
field is initialized because it appears lexically before the initialization of the deposit
field. Consequently, the value of deposit
seen by the constructor, when invoked during the static initialization of c
, is the initial value of deposit
(0) rather than the random value. As a result, the balance is always computed to be -10
.
Wiki Markup |
---|
The _Java Language Specification_ permits implementations to ignore the possibility of such recursive attempts \[[Bloch 2005|AA. Bibliography#Bloch 05]\]. |
...
Step 3 of the detailed initialized procedure described in JLS §12.4.2 [JLS 2014] permits implementations to ignore the possibility of such recursive initialization cycles.
Compliant Solution (Intraclass Cycle)
This compliant solution changes the initialization order of the class Cycle
so that the fields are initialized without creating any dependency cycles. Specifically, the initialization of c
is placed lexically after the initialization of deposit
so that it occurs temporally after deposit
is fully initialized.
Code Block | ||
---|---|---|
| ||
public class Cycle { private final int balance; private static final int deposit = (int) (Math.random() * 100); // Random deposit private static final Cycle c = new Cycle(); // Inserted after initialization of required fields public Cycle() { balance = deposit - 10; // Subtract processing fee } public static void main(String[] args) { System.out.println("The account balance is: " + c.balance); } } |
Such initialization cycles become insidious when many fields are involved; , so it is important to ensure that the control flow lacks such cycles.
Although this compliant solution prevents the initialization cycle, it depends on declaration order and is consequently fragile; later maintainers of the software may be unaware that the declaration order must be maintained to preserve correctness. Consequently, such dependencies must be clearly documented in the code.
Noncompliant Code Example (
...
Interclass Cycle)
This noncompliant code example declares two classes with static variables whose values depend on each other. The cycle is obvious when the classes are seen together (like they are as here) , but this can easily be missed when the classes are viewed but is easy to miss when viewing the classes separately.
Code Block | ||
---|---|---|
| ||
class A { public static public final int a = B.b + 1; // ... } | ||
Code Block | ||
| ||
class B { public static public final int b = A.a + 1; // ... } |
The initialization order of the classes can vary and, consequently, cause causing computation of different values for A.a
and B.b
. When class A
is initialized first, A.a
will have the value 2, and B.b
will have the value 1. These values will be reversed when class B
is initialized first.
Compliant Solution (
...
Interclass Cycle)
This compliant solution breaks the inter-class interclass cycle by eliminating one of the dependencies.the dependency of A
on B
:
Code Block | ||
---|---|---|
| ||
class A { public static public final int a = 2; // ... } // class B unchanged: { public static final int b = A.a + 1; // ... } |
With the cycle broken, the initial values will always be A.a = 2
and B.b = 3
regardless of initialization order.
Noncompliant Code Example
The programmer in this noncompliant code example attempts to initialize a static variable in one class using a static method in a second class, but that method in turn relies on a static method in the first class:
Code Block | ||||
---|---|---|---|---|
| ||||
class A {
public static int a = B.b();
public static int c() { return 1; }
}
class B {
public static int b() { return A.c(); }
}
|
This code correctly initializes A.a
to 1, using the Oracle JVM, regardless of initialization order.whether A
or B
is loaded first. However, the JLS does not guarantee A.a
to be properly initialized. Furthermore, the initialization cycle makes this system harder to maintain and more likely to break in surprising ways when modified.
Compliant Solution
This compliant solution moves the c()
method into class B
, breaking the cycle:
Code Block | ||||
---|---|---|---|---|
| ||||
class A {
public static int a = B.b();
}
class B {
public static int b() { return B.c(); }
public static int c() { return 1; }
}
|
Risk Assessment
Initialization cycles may lead to unexpected results.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|
DCL00-J |
Low |
Unlikely |
Medium | P2 | L3 |
Automated Detection
...
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
CodeSonar |
| JAVA.STRUCT.UA JAVA.STRUCT.UA.DEFAULT | Useless Assignment (Java) Useless Assignment to Default (Java) | ||||||
Parasoft Jtest |
| CERT.DCL00.ACD | Ensure that files do not contain cyclical dependencies | ||||||
PVS-Studio |
| V6050 | |||||||
SonarQube |
| Classes should not access their own subclasses during initialization |
Related Guidelines
...
...
...
Initialization of Variables [LAV] | |
CWE-665, Improper Initialization |
Bibliography
Puzzle 49, "Larger than Life" | |
[JLS 2005] |
Bibliography
...
...
...
...
...
§12.4, "Initialization of Classes and Interfaces" | |
[Seacord 2015] | DCL00-J. Prevent class initialization cycles LiveLesson |
...
Wiki Markup |
---|
\[[MITRE 2009|AA. Bibliography#MITRE 09]\]
[CWE ID 665|http://cwe.mitre.org/data/definitions/665.html] "Improper Initialization" |
—
DCL11-J. Do not derive a value associated with an enum from its ordinal 01. Declarations and Initialization (DCL) DCL13-J. Avoid cyclic dependencies between packages