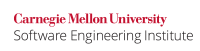
...
Code Block | ||
---|---|---|
| ||
private Object myState = null; // Sets some internal state in the library void setState(Object state) { if (state == null) { // Handle null state } // Defensive copy here when state is mutable if (isInvalidState(state)) { // Handle invalid state } myState = state; } // Performs some action using the state passed earlier void useState() { if (myState == null) { // Handle no state (e.g., null) condition } // ... } |
Exceptions
MET00-J-EX0: Argument validation inside a method may be omitted when the stated contract of a method requires that the caller must validate arguments passed to the method. In this case, the validation must be performed by the caller for all invocations of the method.
MET00-J-EX1: Argument validation may be omitted for arguments whose type adequately constrains the state of the argument. This constraint should be clearly documented in the code.
...
Code Block | ||
---|---|---|
| ||
public int product(int x, int y) { long result = (long) x * y; if (result < Integer.MIN_VALUE || result > Integer.MAX_VALUE) { // Handle error } return (int) result; } |
MET00-J-EX2: Complete validation of all arguments of all methods may introduce added cost and complexity that exceeds its value for all but the most critical code. In such cases, consider argument validation at API boundaries, especially those that may involve interaction with untrusted code.
...