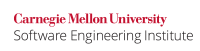
...
In general, holding a lock on any data structure that contains a boxed value can be dangerous.
Noncompliant Code Example
Wiki Markup |
---|
This noncompliant code example uses a {{Boolean}} field to synchronize. However, there can only be two possible values ({{true}} and {{false}}) that a {{Boolean}} can assume. Consequently, any other code that synchronizes on the same value can cause unresponsiveness and deadlocks \[[Findbugs 08|AA. Java References#Findbugs 08]\]. |
Code Block | ||
---|---|---|
| ||
private Boolean initialized = Boolean.FALSE;
synchronized(initialized) {
if (!initialized) {
// perform initialization
initialized = Boolean.TRUE;
}
}
|
Compliant Solution
In the absence of an existing object to lock on, using a raw object to synchronize suffices.
...
Note that the instance of the raw object should not be changed from within the synchronized block. For example, creating and storing the reference of a new object into the lock
field. To prevent such modifications, declare the lock
field final
.
Noncompliant Code Example
Synchronizing on getClass()
rather than a class literal can also be counterproductive. Whenever the implementing class is subclassed, the subclass locks on a completely different Class
object.
...
Finally, it is more important to recognize the entities with whom synchronization is required rather than indiscreetly scavenging for variables or objects to synchronize on.
Noncompliant Code Example
Wiki Markup |
---|
When using synchronization wrappers, the synchronization object must be the {{Collection}} object. The synchronization is necessary to enforce atomicity ([CON38-J. Ensure atomicity of thread-safe code]). This noncompliant code example demonstrates inappropriate synchronization resulting from locking on a {{Collection}} view instead of the Collection itself \[[Tutorials 08|AA. Java References#Tutorials 08]\]. |
...
Code Block | ||
---|---|---|
| ||
// ... synchronized(m) { // Synchronize on m, not s for(Integer k : s) { /* do something */ } } |
Noncompliant Code Example
This noncompliant code example incorrectly uses a ReentrantLock
as the lock object.
...