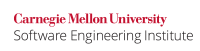
...
Code Block | ||
---|---|---|
| ||
public static boolean isSecureDir(Path file) {
if (!Files.isDirectory( file)) {
return false;
}
if (!file.isAbsolute()) {
file = file.toAbsolutePath();
}
// If any parent dirs (from root on down) are not secure, dir is not secure
for (int i = 1; i <= file.getNameCount(); i++) {
Path partialPath = Paths.get( file.getRoot().toString(), file.subpath( 0, i).toString());
try {
if (Files.isSymbolicLink( partialPath)) {
if (!isSecureDir( Files.readSymbolicLink( partialPath))) {
// Symbolic link, linked-to dir not secure
return false;
}
} else {
FileSystem fileSystem = partialPath.getFileSystem();
UserPrincipalLookupService upls = fileSystem.getUserPrincipalLookupService();
UserPrincipal root = upls.lookupPrincipalByName("root");
UserPrincipal user = upls.lookupPrincipalByName( System.getProperty("user.name"));
UserPrincipal owner = Files.getOwner( partialPath);
if (!owner.equals( user) && !owner.equals( root)) {
// dir owned by someone else, not secure
return false;
}
PosixFileAttributes attr = Files.readAttributes( partialPath, PosixFileAttributes.class);
Set<PosixFilePermission> perms = attr.permissions();
if (perms.contains( PosixFilePermission.GROUP_WRITE) ||
perms.contains( PosixFilePermission.OTHERS_WRITE)) {
// someone else can write files, not secure
return false;
}
}
} catch (IOException x) {
x.printStackTrace(System.out);
return false;
}
}
return true;
}
|
...