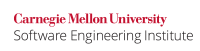
...
Code Block | ||
---|---|---|
| ||
private Integer lock = new Integer(0); private void doSomething() { synchronized(lock) { /* ... */ } } public void setLock(Integer lockvalue) { lock = lockValue; } |
This is because the thread that holds a lock on the nonfinal field object can modify the field's value, allowing another thread that is blocked on the unmodified value to resume, at the same time, contending for the lock with a third thread that is blocked on the modified value. It is insecure to synchronize on a mutable field because this is equivalent to synchronizing on the field's contents. This is a lock mutability problem as opposed to the lock sharing issue specific to string literals and boxed primitives.
...
Code Block | ||
---|---|---|
| ||
private final Integer lock = new Integer(0); private void doSomething() { synchronized(lock) { /* ... */ } } // setValue() is disallowed |
As long as the lock object is final
, it is acceptable for the referenced object to be mutable. In this compliant solution, the Integer
object happens to be immutable by definition.
...