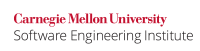
The ISO/IEC 9899-1999 C standard function fopen()
is typically used to open an existing file or create a new one. However, fopen()
does not indicate if an existing file has been opened for writing or a new file has been created. This may lead to a program overwriting or accessing an unintended file.
Non-Compliant Code Example
In this example, an attempt is made to check whether a file exists before opening it for writing by trying to open the file for reading.
... FILE *fp = fopen("foo.txt","r"); if( !fp ) { /* file does not exist */ FILE *new_fp = fopen("foo.txt","w"); ... fclose(new_fp); } else { /* file exists */ fclose(fp); } ...
However, this code suffers from a Time of Check, Time of Use (or TOCTOU) vulnerability (see [[Seacord 05]] Section 7.2). On a shared multitasking system there is a window of opportunity between the first call of fopen()
and the second call for a malicious attacker to, for example, create a link with the given filename to an existing file, so that the existing file is overwritten by the second call of fopen()
and the subsequent writing to the file.
Compliant Solution 1
The fopen()
function does not indicate if an existing file has been opened for writing or a new file has been created. However, the open()
function as defined in the Open Group Base Specifications Issue 6 [[Open Group 04]] is available on many platforms and provides such a mechanism. If the O_CREAT
and O_EXCL
flags are used together, the open()
function fails when the file specified by file_name
already exists.
... int fd = open(file_name, O_CREAT | O_EXCL | O_WRONLY, new_file_mode); if (fd == -1) { /* Handle Error */ } ...
Compliant Solution 2
The function fdopen()
[[Open Group 04]] can be used in conjunction with open()
to determine if a file is opened or created, and then associate a stream with the file descriptor.
... FILE *fp; int fd; fd = open(file_name, O_CREAT | O_EXCL | O_WRONLY, new_file_mode); if (fd == -1) { /* Handle Error */ } fp = fdopen(fd,"w"); if (fp == NULL) { /* Handle Error */ } ...
Risk Assessment
The ability to determine if an existing file has been opened, or a new file has been created provides greater assurance that the file accessed is the one that was intended.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO03-A |
3 (high) |
2 (probable) |
1 (high) |
P6 |
L2 |
References
- Seacord 05 Chapter 7, File I/O
- ISO/IEC 9899-1999 Sections 7.19.3, Files
- ISO/IEC 9899-1999 Sections 7.19.4, Operations on Files
- Open Group 04