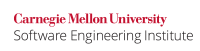
Lambda expressions may capture objects with automatic storage duration from the set of enclosing scopes (called the reaching scope) for use in the lambda's function body. These captures may be either explicit, by specifying the object to capture in the lambda's capture-list, or implicit, by using a capture-default and referring to the object within the lambda's function body. When capturing an object explicitly or implicitly, the capture-default indicates that the object is either captured by copy (using =)
or captured by reference (using &
). When an object is captured by copy, the lambda object will contain an unnamed nonstatic data member that is initialized to the value of the object being captured. This nonstatic data member's lifetime is that of the lambda object's lifetime. However, when an object is captured by reference, the lifetime of the referent is not tied to the lifetime of the lambda object.
Because entities captured are objects with automatic storage duration (or this
), a general guideline is that functions returning a lambda object (including returning via a reference parameter), or storing a lambda object in a member variable or global, should not capture an entity by reference because the lambda object often outlives the captured reference object.
When a lambda object outlives one of its reference-captured objects, execution of the lambda object's function call operator results in undefined behavior once that reference-captured object is accessed. Therefore, a lambda object must not outlive any of its reference-captured objects. This is a specific instance of EXP54-CPP. Do not access an object outside of its lifetime.
Noncompliant Code Example
In this noncompliant code example, the function g()
returns a lambda, which implicitly captures the automatic local variable i
by reference. When that lambda is returned from the call, the reference it captured will refer to a variable whose lifetime has ended. As a result, when the lambda is executed in f()
, the use of the dangling reference in the lambda results in undefined behavior.
auto g() { int i = 12; return [&] { i = 100; return i; }; } void f() { int j = g()(); }
Compliant Solution
In this compliant solution, the lambda does not capture i
by reference but instead captures it by copy. Consequently, the lambda contains an implicit nonstatic data member whose lifetime is that of the lambda.
auto g() { int i = 12; return [=] () mutable { i = 100; return i; }; } void f() { int j = g()(); }
Noncompliant Code Example
In this noncompliant code example, a lambda reference captures a local variable from an outer lambda. However, this inner lambda outlives the lifetime of the outer lambda and any automatic local variables it defines, resulting in undefined behavior when an inner lambda object is executed within f()
.
auto g(int val) { auto outer = [val] { int i = val; auto inner = [&] { i += 30; return i; }; return inner; }; return outer(); } void f() { auto fn = g(12); int j = fn(); }
Compliant Solution
In this compliant solution, the inner lambda captures i
by copy instead of by reference.
auto g(int val) { auto outer = [val] { int i = val; auto inner = [i] { return i + 30; }; return inner; }; return outer(); } void f() { auto fn = g(12); int j = fn(); }
Risk Assessment
Referencing an object outside of its lifetime can result in an attacker being able to run arbitrary code.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
EXP61-CPP | High | Probable | High | P6 | L2 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Astrée | 22.10 | invalid_pointer_dereference | |
Helix QAC | 2024.1 | DF4706, DF4707, DF4708 | |
Klocwork | 2024.1 | LOCRET.RET | |
Parasoft C/C++test | 2023.1 | CERT_CPP-EXP61-a | Never return lambdas that capture local objects by reference |
Polyspace Bug Finder | R2023b | CERT C++: EXP61-CPP | Checks for situations where object escapes scope through lambda expressions (rule fully covered) |
PVS-Studio | 7.30 | V1047 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
Bibliography
[ISO/IEC 14882-2014] | Subclause 3.8, "Object Lifetime" Subclause 5.1.2, "Lambda Expressions" |