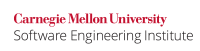
Thread startup can be misleading because the code can appear to be performing its function correctly when it is actually being executed by the wrong thread.
Invoking the Thread.start()
method instructs the Java runtime to start executing the thread's run()
method using the started thread. Invoking a Thread
object's run()
method directly is incorrect. When a Thread
object's run()
method is invoked directly, the statements in the run()
method are executed by the current thread rather than by the newly created thread. Furthermore, if the Thread
object was constructed by instantiating a subclass of Thread
that fails to override the run()
method rather than constructed from a Runnable
object, any calls to the subclass's run()
method would invoke Thread.run()
, which does nothing. Consequently, programs must not directly invoke a Thread
object's run()
method.
Noncompliant Code Example
This noncompliant code example explicitly invokes run()
in the context of the current thread:
public final class Foo implements Runnable { @Override public void run() { // ... } public static void main(String[] args) { Foo foo = new Foo(); new Thread(foo).run(); } }
The newly created thread is never started because of the incorrect assumption that run()
starts the new thread. Consequently, the statements in the run()
method are executed by the current thread rather than by the new thread.
Compliant Solution
This compliant solution correctly uses the start()
method to tell the Java runtime to start a new thread:
public final class Foo implements Runnable { @Override public void run() { // ... } public static void main(String[] args) { Foo foo = new Foo(); new Thread(foo).start(); } }
Exceptions
THI00-J-EX0: The run()
method may be directly invoked during unit testing. Note that this method cannot be used to test a class for multithreaded use.
Given a Thread
object that was constructed with a runnable argument, when invoking the Thread.run()
method, the Thread
object may be cast to Runnable
to eliminate analyzer diagnostics:
public void sampleRunTest() { Thread thread = new Thread(new Runnable() { @Override public void run() { // ... } }); ((Runnable) thread).run(); // THI00-J-EX0: Does not start a new thread }
Casting a thread to Runnable
before calling the run()
method documents that the explicit call to Thread.run()
is intentional. Adding an explanatory comment alongside the invocation is highly recommended.
THI00-J-EX1: Runtime system code involved in starting new threads is permitted to invoke a Thread
object's run()
method directly; this is an obvious necessity for a working Java runtime system. Note that the likelihood that this exception applies to user-written code is vanishingly small.
Risk Assessment
Failure to start threads correctly can cause unexpected behavior.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
THI00-J | Low | Probable | Medium | P4 | L3 |
Automated Detection
Automated detection of direct invocations of Thread.run()
methods is straightforward. Sound automated determination of which specific invocations are permitted may be infeasible. Heuristic approaches may be useful.
Tool | Version | Checker | Description |
---|---|---|---|
CodeSonar | 8.1p0 | JAVA.CONCURRENCY.LOCK.SCTB | Synchronous Call to Thread Body (Java) |
Coverity | 7.5 | DC.THREADING.thread_run | Implemented |
Parasoft Jtest | 2023.1 | CERT.THI00.IRUN | Do not call the 'run()' method directly on classes extending 'java.lang.Thread' or implementing 'java.lang.Runnable' |
PVS-Studio | 7.30 | V6064 | |
SonarQube | 9.9 | S1217 | Thread.run() should not be called directly |
Related Guidelines
Android Implementation Details
Android provides a couple of solutions for threading. The Android Developers Blog's article "Painless Threading" discusses those solutions.
6 Comments
David Svoboda
This is a good rule, so far, simple, yet realistic. (I think I've made this mistake in the past.)
I wonder if we should be stronger with this rule. In particular, perhaps this rule should never invoke the run() method on a Thread object. (A side note is that if a thread was not constructed from a Runnable object, than Thread.run() does nothing.) If you have a Thread object and you must run it in this thread, and you know it is a Runnable, then cast it to a Runnable b/f calling run().
Dhruv Mohindra
This change is in now as an exception. Thanks.
David Svoboda
IIRC there used to be two exceptions to this rule: one saying "unit testing is ok" and one saying "cast a Thread object to a Runnable first". They seem to have been merged into one exception...why?
Dhruv Mohindra
Because it is assumed that the run method should be used for unit testing its functionality only. Casting it to Runnable just documents such as exception and does not serve as a valid exception on its own.
Pennie Walters
Automated Detection is not done yet. It's marked as TODO.
Dean Sutherland
Obvious heuristic for checking this rule:
Thread
object is cast to aRunnable
first.@COSCGExcept("THI02-EX2")
to suppress errors for the vanishingly few instances where the call is legitimate.