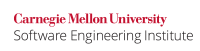
The interfaces of the Java Collections Framework [JCF 2014] use generically typed, parameterized methods, such as add(E e)
and put(K key, V value)
, to insert objects into the collection or map, but they have other methods, such as contains()
, remove()
, and get()
, that accept an argument of type Object
rather than a parameterized type. Consequently, these methods accept an object of any type. The collections framework interfaces were designed in this manner to maximize backwards compatibility, but this design can also lead to coding errors. Programmers must ensure that arguments passed to methods such as Map<K,V>
get()
, Collection<E>
contains()
, and remove()
have the same type as the parameterized type of the corresponding class instance.
Noncompliant Code Example
After adding and removing 10 elements, the HashSet
in this noncompliant code example still contains 10
elements and not the expected 0. Java's type checking requires that only values of type Short
can be inserted into s. Consequently, the programmer has added a cast to short
so that the code will compile. However, the Collections<E>.remove()
method accepts an argument of type Object
rather than of type E
, allowing a programmer to attempt to remove an object of any type. In this noncompliant code example, the programmer has neglected to also cast the variable i
before passing it to the remove()
method, which is autoboxed into an object of type Integer
rather than one of type Short
. The HashSet
contains only values of type Short
; the code attempts to remove objects of type Integer
. Consequently, the remove()
method has no effect.
import java.util.HashSet; public class ShortSet { public static void main(String[] args) { HashSet<Short> s = new HashSet<Short>(); for (int i = 0; i < 10; i++) { s.add((short)i); // Cast required so that the code compiles s.remove(i); // Tries to remove an Integer } System.out.println(s.size()); } }
This noncompliant code example also violates EXP00-J. Do not ignore values returned by methods because the remove()
method returns a Boolean value indicating its success.
Compliant Solution
Objects removed from a collection must share the type of the collection elements. Numeric promotion and autoboxing can produce unexpected object types. This compliant solution uses an explicit cast to short
that matches the intended boxed type.
import java.util.HashSet; public class ShortSet { public static void main(String[] args) { HashSet<Short> s = new HashSet<Short>(); for (int i = 0; i < 10; i++) { s.add((short)i); // Remove a Short if (s.remove((short)i) == false) { System.err.println("Error removing " + i); } } System.out.println(s.size()); } }
Exceptions
EXP04-J-EX1: The collections framework equals()
method also takes an argument of type Object
, but it is acceptable to pass an object of a different type from that of the underlying collection/map to the equals()
method. Doing so cannot cause any confusion because the contract of the equals()
method stipulates that objects of different classes will never be equivalent (see MET08-J. Preserve the equality contract when overriding the equals() method for more information).
EXP04-J-EX2: Some Java programs, particularly legacy programs, may iterate through a collection of variously typed objects with the expectation that only those objects with the same type as the collection parameter will be operated on. An exception is allowed when there is no expectation that the operation is not a no-op.
Risk Assessment
Passing arguments to certain Java Collection Framework methods that are of a different type from that of the class instance can cause silent failures, resulting in unintended object retention, memory leaks, or incorrect program operation [Techtalk 2007].
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
EXP04-J | Low | Probable | Low | P6 | L2 |
Automated Detection
Detection of invocations of Collection.remove()
whose operand fails to match the type of the elements of the underlying collection is straightforward. It is possible, although unlikely, that some of these invocations could be intended. The remainder are heuristically likely to be in error. Automated detection for other APIs could be possible.
Tool | Version | Checker | Description |
---|---|---|---|
PVS-Studio | 7.36 | V6066 | |
SonarQube | 9.9 | S2175 | Inappropriate "Collection" calls should not be made |
Bibliography
Chapter 5, "Inheritance" | |
[JCF 2014] | The Java Collections Framework |
[JLS 2015] | |
[Seacord 2015] | IDS17-J. Prevent XML External Entity Attacks LiveLesson |
"The Joy of Sets" |