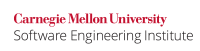
The C programming language provides several ways to allocate memory, such as std::malloc()
, std::calloc()
, and std::realloc()
, which can be used by a C++ program. However, the C programming language defines only a single way to free the allocated memory: std::free()
. See MEM31-C. Free dynamically allocated memory when no longer needed and MEM34-C. Only free memory allocated dynamically for rules specifically regarding C allocation and deallocation requirements.
The C++ programming language adds additional ways to allocate memory, such as the operators new
, new[]
, and placement new
, and allocator objects. Unlike C, C++ provides multiple ways to free dynamically allocated memory, such as the operators delete
, delete[]()
, and deallocation functions on allocator objects.
Do not call a deallocation function on anything other than nullptr
, or a pointer returned by the corresponding allocation function described by the following.
Allocator | Deallocator |
---|---|
global operator new()/new | global operator delete ()/delete |
global operator new[]()/new[] | global operator delete[]()/delete[] |
class-specific operator new()/new |
class-specific operator delete ()/delete |
class-specific operator new[]()/new[] |
class-specific operator delete[]()/delete[] |
placement operator new () | N/A |
allocator<T>::allocate() |
|
std::malloc() , std::calloc() ,
std::realloc() |
std::free() |
std::get_temporary_buffer() | std::return_temporary_buffer() |
Passing a pointer value to an inappropriate deallocation function can result in undefined behavior.
The C++ Standard, [expr.delete], paragraph 2 [ISO/IEC 14882-2014], in part, states the following:
In the first alternative (delete object), the value of the operand of
delete
may be a null pointer value, a pointer to a non-array object created by a previous new-expression, or a pointer to a subobject (1.8) representing a base class of such an object (Clause 10). If not, the behavior is undefined. In the second alternative (delete array), the value of the operand ofdelete
may be a null pointer value or a pointer value that resulted from a previous array new-expression. If not, the behavior is undefined.
Deallocating a pointer that is not allocated dynamically (including non-dynamic pointers returned from calls to placement new()
) is undefined behavior because the pointer value was not obtained by an allocation function. Deallocating a pointer that has already been passed to a deallocation function is undefined behavior because the pointer value no longer points to memory that has been dynamically allocated.
When an operator such as new
is called, it results in a call to an overloadable operator of the same name, such as operator new()
. These overloadable functions can be called directly but carry the same restrictions as their operator counterparts. That is, calling operator delete()
and passing a pointer parameter has the same constraints as calling the delete
operator on that pointer. Further, the overloads are subject to scope resolution, so it is possible (but not permissible) to call a class-specific operator to allocate an object but a global operator to deallocate the object.
See MEM53-CPP. Explicitly construct and destruct objects when manually managing object lifetime for information on lifetime management of objects when using memory management functions other than the new
and delete
operators.
Noncompliant Code Example (placement new()
)
In this noncompliant code example, the local variable space
is passed as the expression to the placement new
operator. The resulting pointer of that call is then passed to ::operator delete()
, resulting in undefined behavior due to ::operator delete()
attempting to free memory that was not returned by ::operator new()
.
#include <iostream> struct S { S() { std::cout << "S::S()" << std::endl; } ~S() { std::cout << "S::~S()" << std::endl; } }; void f() { alignas(struct S) char space[sizeof(struct S)]; S *s1 = new (&space) S; // ... delete s1; }
Compliant Solution (placement new()
)
This compliant solution removes the call to ::operator delete()
, instead explicitly calling s1
's destructor. This is one of the few times when explicitly invoking a destructor is warranted.
#include <iostream> struct S { S() { std::cout << "S::S()" << std::endl; } ~S() { std::cout << "S::~S()" << std::endl; } }; void f() { alignas(struct S) char space[sizeof(struct S)]; S *s1 = new (&space) S; // ... s1->~S(); }
Noncompliant Code Example (Uninitialized delete
)
In this noncompliant code example, two allocations are attempted within the same try
block, and if either fails, the catch
handler attempts to free resources that have been allocated. However, because the pointer variables have not been initialized to a known value, a failure to allocate memory for i1
may result in passing ::operator delete()
a value (in i2
) that was not previously returned by a call to ::operator new()
, resulting in undefined behavior.
#include <new> void f() { int *i1, *i2; try { i1 = new int; i2 = new int; } catch (std::bad_alloc &) { delete i1; delete i2; } }
Compliant Solution (Uninitialized delete
)
This compliant solution initializes both pointer values to nullptr
, which is a valid value to pass to ::operator delete().
#include <new> void f() { int *i1 = nullptr, *i2 = nullptr; try { i1 = new int; i2 = new int; } catch (std::bad_alloc &) { delete i1; delete i2; } }
Noncompliant Code Example (Double-Free)
Once a pointer is passed to the proper deallocation function, that pointer value is invalidated. Passing the pointer to a deallocation function a second time when the pointer value has not been returned by a subsequent call to an allocation function results in an attempt to free memory that has not been allocated dynamically. The underlying data structures that manage the heap can become corrupted in a way that can introduce security vulnerabilities into a program. These types of issues are called double-free vulnerabilities. In practice, double-free vulnerabilities can be exploited to execute arbitrary code.
In this noncompliant code example, the class C
is given ownership of a P *
, which is subsequently deleted by the class destructor. The C++ Standard, [class.copy], paragraph 7 [ISO/IEC 14882-2014], states the following:
If the class definition does not explicitly declare a copy constructor, one is declared implicitly. If the class definition declares a move constructor or move assignment operator, the implicitly declared copy constructor is defined as deleted; otherwise, it is defined as defaulted (8.4). The latter case is deprecated if the class has a user-declared copy assignment operator or a user-declared destructor.
Despite the presence of a user-declared destructor, C
will have an implicitly defaulted copy constructor defined for it, and this defaulted copy constructor will copy the pointer value stored in p
, resulting in a double-free: the first free happens when g()
exits and the second free happens when h()
exits.
struct P {}; class C { P *p; public: C(P *p) : p(p) {} ~C() { delete p; } void f() {} }; void g(C c) { c.f(); } void h() { P *p = new P; C c(p); g(c); }
Compliant Solution (Double-Free)
In this compliant solution, the copy constructor and copy assignment operator for C
are explicitly deleted. This deletion would result in an ill-formed program with the definition of g()
from the preceding noncompliant code example due to use of the deleted copy constructor. Consequently, g()
was modified to accept its parameter by reference, removing the double-free.
struct P {}; class C { P *p; public: C(P *p) : p(p) {} C(const C&) = delete; ~C() { delete p; } void operator=(const C&) = delete; void f() {} }; void g(C &c) { c.f(); } void h() { P *p = new P; C c(p); g(c); }
Noncompliant Code Example (array new[]
)
In the following noncompliant code example, an array is allocated with array new[]
but is deallocated with a scalar delete
call instead of an array delete[]
call, resulting in undefined behavior.
void f() { int *array = new int[10]; // ... delete array; }
Compliant Solution (array new[]
)
In the compliant solution, the code is fixed by replacing the call to delete
with a call to delete []
to adhere to the correct pairing of memory allocation and deallocation functions.
void f() { int *array = new int[10]; // ... delete[] array; }
Noncompliant Code Example (malloc()
)
In this noncompliant code example, the call to malloc()
is mixed with a call to delete
.
#include <cstdlib> void f() { int *i = static_cast<int *>(std::malloc(sizeof(int))); // ... delete i; }
This code does not violate MEM53-CPP. Explicitly construct and destruct objects when manually managing object lifetime because it complies with the MEM53-CPP-EX1 exception.
Implementation Details
Some implementations of ::operator new()
result in calling std::malloc()
. On such implementations, the ::operator delete()
function is required to call std::free()
to deallocate the pointer, and the noncompliant code example would behave in a well-defined manner. However, this is an implementation detail and should not be relied on—implementations are under no obligation to use underlying C memory management functions to implement C++ memory management operators.
Compliant Solution (malloc()
)
In this compliant solution, the pointer allocated by std::malloc()
is deallocated by a call to std::free()
instead of delete.
#include <cstdlib> void f() { int *i = static_cast<int *>(std::malloc(sizeof(int))); // ... std::free(i); }
Noncompliant Code Example ( new
)
In this noncompliant code example, std::free()
is called to deallocate memory that was allocated by new
. A common side effect of the undefined behavior caused by using the incorrect deallocation function is that destructors will not be called for the object being deallocated by std::free().
#include <cstdlib> struct S { ~S(); }; void f() { S *s = new S(); // ... std::free(s); }
Additionally, this code violates MEM53-CPP. Explicitly construct and destruct objects when manually managing object lifetime.
Compliant Solution (new
)
In this compliant solution, the pointer allocated by new
is deallocated by calling delete
instead of std::free().
struct S { ~S(); }; void f() { S *s = new S(); // ... delete s; }
Noncompliant Code Example (Class new
)
In this noncompliant code example, the global new
operator is overridden by a class-specific implementation of operator new()
. When new
is called, the class-specific override is selected, so S::operator new()
is called. However, because the object is destroyed with a scoped ::delete
operator, the global operator delete()
function is called instead of the class-specific implementation S::operator delete()
, resulting in undefined behavior.
#include <cstdlib> #include <new> struct S { static void *operator new(std::size_t size) noexcept(true) { return std::malloc(size); } static void operator delete(void *ptr) noexcept(true) { std::free(ptr); } }; void f() { S *s = new S; ::delete s; }
Compliant Solution (class new
)
In this compliant solution, the scoped ::delete
call is replaced by a nonscoped delete
call, resulting in S::operator delete()
being called.
#include <cstdlib> #include <new> struct S { static void *operator new(std::size_t size) noexcept(true) { return std::malloc(size); } static void operator delete(void *ptr) noexcept(true) { std::free(ptr); } }; void f() { S *s = new S; delete s; }
Noncompliant Code Example (std::unique_ptr
)
In this noncompliant code example, a std::unique_ptr
is declared to hold a pointer to an object, but is direct-initialized with an array of objects. When the std::unique_ptr
is destroyed, its default deleter calls delete
instead of delete[]
, resulting in undefined behavior.
#include <memory> struct S {}; void f() { std::unique_ptr<S> s{new S[10]}; }
Compliant Solution (std::unique_ptr
)
In this compliant solution, the std::unique_ptr
is declared to hold an array of objects instead of a pointer to an object. Additionally, std::make_unique()
is used to initialize the smart pointer.
#include <memory> struct S {}; void f() { std::unique_ptr<S[]> s = std::make_unique<S[]>(10); }
Use of std::make_unique()
instead of direct initialization will emit a diagnostic if the resulting std::unique_ptr
is not of the correct type. Had it been used in the noncompliant code example, the result would have been an ill-formed program instead of undefined behavior. It is best to use std::make_unique()
instead of manual initialization by other means.
Noncompliant Code Example (std::shared_ptr
)
In this noncompliant code example, a std::shared_ptr
is declared to hold a pointer to an object, but is direct-initialized with an array of objects. As with std::unique_ptr
, when the std::shared_ptr
is destroyed, its default deleter calls delete
instead of delete[]
, resulting in undefined behavior.
#include <memory> struct S {}; void f() { std::shared_ptr<S> s{new S[10]}; }
Compliant Solution (std::shared_ptr
)
Unlike the compliant solution for std::unique_ptr
, where std::make_unique()
is called to create a unique pointer to an array, it is ill-formed to call std::make_shared()
with an array type. Instead, this compliant solution manually specifies a custom deleter for the shared pointer type, ensuring that the underlying array is properly deleted.
#include <memory> struct S {}; void f() { std::shared_ptr<S> s{new S[10], [](const S *ptr) { delete [] ptr; }}; }
Risk Assessment
Passing a pointer value to a deallocation function that was not previously obtained by the matching allocation function results in undefined behavior, which can lead to exploitable vulnerabilities.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MEM51-CPP | High | Likely | Medium | P18 | L1 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Astrée | 22.10 | invalid_dynamic_memory_allocation dangling_pointer_use | |
Axivion Bauhaus Suite | 7.2.0 | CertC++-MEM51 | |
Clang | 3.9 | clang-analyzer-cplusplus.NewDeleteLeaks -Wmismatched-new-delete | Checked by clang-tidy , but does not catch all violations of this rule |
CodeSonar | 9.0p0 | ALLOC.DF | Double free Type mismatch Leak |
Helix QAC | 2025.1 | C++2110, C++2111, C++2112, C++2113, C++2118, C++3337, C++3339, C++4262, C++4263, C++4264 | |
Klocwork | 2025.1 | CL.FFM.ASSIGN CL.FFM.COPY CL.FMM CL.SHALLOW.ASSIGN CL.SHALLOW.COPY FMM.MIGHT FMM.MUST FNH.MIGHT FNH.MUST FUM.GEN.MIGHT FUM.GEN.MUST UNINIT.CTOR.MIGHT UNINIT.CTOR.MUST UNINIT.HEAP.MIGHT UNINIT.HEAP.MUST | |
LDRA tool suite | 9.7.1
| 232 S, 236 S, 239 S, 407 S, 469 S, 470 S, 483 S, 484 S, 485 S, 64 D, 112 D | Partially implemented |
Parasoft C/C++test | 2024.2 | CERT_CPP-MEM51-a | Use the same form in corresponding calls to new/malloc and delete/free |
Parasoft Insure++ | Runtime detection | ||
Polyspace Bug Finder | R2024b | CERT C++: MEM51-CPP | Checks for:
Rule partially covered. |
PVS-Studio | 7.37 | V515, V554, V611, V701, V748, V773, V1066 | |
SonarQube C/C++ Plugin | 4.10 | S1232 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
SEI CERT C++ Coding Standard | MEM53-CPP. Explicitly construct and destruct objects when manually managing object lifetime |
SEI CERT C Coding Standard | MEM31-C. Free dynamically allocated memory when no longer needed |
MITRE CWE | CWE 590, Free of Memory Not on the Heap |
Bibliography
[Dowd 2007] | "Attacking delete and delete [] in C++" | ||
[Henricson 1997] | Rule 8.1, "delete should only be used with new" Rule 8.2, " delete [] should only be used with new []" | ||
[ISO/IEC 14882-2014] | Subclause 5.3.5, "Delete" | ||
[Meyers 2005] | Item 16, "Use the Same Form in Corresponding Uses of new and delete " | ||
[Seacord 2013] | Chapter 4, "Dynamic Memory Management" | ||
[Viega 2005] | "Doubly Freeing Memory" |
7 Comments
Joseph C. Sible
I dislike the way this page addresses placement new. Two main problems with it:
David Svoboda
I agree with these criticisms. I fixed them in the 'placement new' code examples.
Aaron Ballman
One thing I noticed as a drive by from these changes is that we're only accidentally correct with the placement new CS (and probably others, but I did not check). It is not accounting for the alignment requirements for that type. I think the declaration should be more like:
alignas(S) char space[sizeof(S)];
David Svoboda
First of all, I just changed both 'placement new' code examples to use a char array for placement new rather than an
S
object. That may affect the alignment...It's been a while since I studied alignment, but my recollection is that an unaligned stack declaration would conform to alignment of the most restrictive type (at least in C). The concern would be if we used an unaligned address like
&(space + 1)
. But using&space
should always be safe wrt alignment.Joseph C. Sible
I think that's worth double-checking. I've never heard of such a guarantee.
David Svoboda
C17, 6.7.5 also addresses alignment, using _Alignas. The 'strictest' alignment I suggested applies to pointers returned by malloc(), not to stack variables.
Aaron Ballman
Stack alignment is based on the declared type + any explicit alignment specifiers. See C17 6.2.8p1 and p6. The alignment of char is the weakest alignment and the issue is that alignment requirements for
struct S
may be stronger.