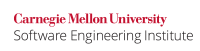
According to the Java Language Specification, §8.3.1.4, "volatile
Fields" [JLS 2013],
A field may be declared
volatile
, in which case the Java Memory Model ensures that all threads see a consistent value for the variable (§17.4).
This safe publication guarantee applies only to primitive fields and object references. Programmers commonly use imprecise terminology and speak about "member objects." For the purposes of this visibility guarantee, the actual member is the object reference; the objects referred to (aka referents) by volatile object references are beyond the scope of the safe publication guarantee. Consequently, declaring an object reference to be volatile is insufficient to guarantee that changes to the members of the referent are published to other threads. A thread may fail to observe a recent write from another thread to a member field of such an object referent.
Furthermore, when the referent is mutable and lacks thread safety, other threads might see a partially constructed object or an object in a (temporarily) inconsistent state [Goetz 2007]. However, when the referent is immutable, declaring the reference volatile suffices to guarantee safe publication of the members of the referent. Programmers cannot use the volatile
keyword to guarantee safe publication of mutable objects. Use of the volatile
keyword can only guarantee safe publication of primitive fields, object references, or fields of immutable object referents.
Confusing a volatile object with the volatility of its member objects is a similar error to the one described in OBJ50-J. Never confuse the immutability of a reference with that of the referenced object.
Noncompliant Code Example (Arrays)
This noncompliant code example declares a volatile reference to an array object.
final class Foo { private volatile int[] arr = new int[20]; public int getFirst() { return arr[0]; } public void setFirst(int n) { arr[0] = n; } // ... }
Values assigned to an array element by one thread—for example, by calling setFirst()
—might not be visible to another thread calling getFirst()
because the volatile
keyword guarantees safe publication only for the array reference; it makes no guarantee regarding the actual data contained within the array.
This problem arises when the thread that calls setFirst()
and the thread that calls getFirst()
lack a happens-before relationship. A happens-before relationship exists between a thread that writes to a volatile variable and a thread that subsequently reads it. However, setFirst()
and getFirst()
read only from a volatile variable—the volatile reference to the array. Neither method writes to the volatile variable.
Compliant Solution (AtomicIntegerArray
)
To ensure that the writes to array elements are atomic and that the resulting values are visible to other threads, this compliant solution uses the AtomicIntegerArray
class defined in java.util.concurrent.atomic
.
final class Foo { private final AtomicIntegerArray atomicArray = new AtomicIntegerArray(20); public int getFirst() { return atomicArray.get(0); } public void setFirst(int n) { atomicArray.set(0, 10); } // ... }
AtomicIntegerArray
guarantees a happens-before relationship between a thread that calls atomicArray.set()
and a thread that subsequently calls atomicArray.get()
.
Compliant Solution (Synchronization)
To ensure visibility, accessor methods may synchronize access while performing operations on nonvolatile elements of an array, whether the array is referred to by a volatile or a nonvolatile reference. Note that the code is thread-safe even though the array reference is not volatile.
final class Foo { private int[] arr = new int[20]; public synchronized int getFirst() { return arr[0]; } public synchronized void setFirst(int n) { arr[0] = n; } }
Synchronization establishes a happens-before relationship between threads that synchronize on the same lock. In this case, the thread that calls setFirst()
and the thread that subsequently calls getFirst()
on the same object instance both synchronize on that instance, so safe publication is guaranteed.
Noncompliant Code Example (Mutable Object)
This noncompliant code example declares the Map
instance field volatile. The instance of the Map
object is mutable because of its put()
method.
final class Foo { private volatile Map<String, String> map; public Foo() { map = new HashMap<String, String>(); // Load some useful values into map } public String get(String s) { return map.get(s); } public void put(String key, String value) { // Validate the values before inserting if (!value.matches("[\\w]*")) { throw new IllegalArgumentException(); } map.put(key, value); } }
Interleaved calls to get()
and put()
may result in the retrieval of internally inconsistent values from the Map
object because put()
modifies its state. Declaring the object reference volatile is insufficient to eliminate this data race.
Noncompliant Code Example (Volatile-Read, Synchronized-Write)
This noncompliant code example attempts to use the volatile-read, synchronized-write technique described in Java Theory and Practice [Goetz 2007]. The map
field is declared volatile to synchronize its reads and writes. The put()
method is also synchronized to ensure that its statements are executed atomically.
final class Foo { private volatile Map<String, String> map; public Foo() { map = new HashMap<String, String>(); // Load some useful values into map } public String get(String s) { return map.get(s); } public synchronized void put(String key, String value) { // Validate the values before inserting if (!value.matches("[\\w]*")) { throw new IllegalArgumentException(); } map.put(key, value); } }
The volatile-read, synchronized-write technique uses synchronization to preserve atomicity of compound operations, such as increment, and provides faster access times for atomic reads. However, it fails for mutable objects because the safe publication guarantee provided by volatile
extends only to the field itself (the primitive value or object reference); the referent is excluded from the guarantee, as are the referent's members. In effect, the write and a subsequent read of the map
lack a happens-before relationship.
This technique is also discussed in VNA02-J. Ensure that compound operations on shared variables are atomic.
Compliant Solution (Synchronized)
This compliant solution uses method synchronization to guarantee visibility:
final class Foo { private final Map<String, String> map; public Foo() { map = new HashMap<String, String>(); // Load some useful values into map } public synchronized String get(String s) { return map.get(s); } public synchronized void put(String key, String value) { // Validate the values before inserting if (!value.matches("[\\w]*")) { throw new IllegalArgumentException(); } map.put(key, value); } }
It is unnecessary to declare the map
field volatile because the accessor methods are synchronized. The field is declared final
to prevent publication of its reference when the referent is in a partially initialized state (see TSM03-J. Do not publish partially initialized objects for more information).
Noncompliant Code Example (Mutable Subobject)
In this noncompliant code example, the volatile format
field stores a reference to a mutable object, java.text.DateFormat
:
final class DateHandler { private static volatile DateFormat format = DateFormat.getDateInstance(DateFormat.MEDIUM); public static java.util.Date parse(String str) throws ParseException { return format.parse(str); } }
Because DateFormat
is not thread-safe [API 2013], the value for Date
returned by the parse()
method may not correspond to the str
argument:
Compliant Solution (Instance per Call/Defensive Copying)
This compliant solution creates and returns a new DateFormat
instance for each invocation of the parse()
method [API 2013]:
final class DateHandler { public static java.util.Date parse(String str) throws ParseException { return DateFormat.getDateInstance( DateFormat.MEDIUM).parse(str); } }
Compliant Solution (Synchronization)
This compliant solution makes DateHandler
thread-safe by synchronizing statements within the parse()
method [API 2013]:
final class DateHandler { private static DateFormat format = DateFormat.getDateInstance(DateFormat.MEDIUM); public static java.util.Date parse(String str) throws ParseException { synchronized (format) { return format.parse(str); } } }
Compliant Solution (ThreadLocal
Storage)
This compliant solution uses a ThreadLocal
object to create a separate DateFormat
instance per thread:
final class DateHandler { private static final ThreadLocal<DateFormat> format = new ThreadLocal<DateFormat>() { @Override protected DateFormat initialValue() { return DateFormat.getDateInstance(DateFormat.MEDIUM); } }; // ... }
Applicability
Incorrectly assuming that declaring a field volatile guarantees safe publication of a referenced object's members can cause threads to observe stale or inconsistent values.
Technically, strict immutability of the referent is a stronger condition than is fundamentally required for safe publication. When it can be determined that a referent is thread-safe by design, the field that holds its reference may be declared volatile. However, this approach to using volatile
decreases maintainability and should be avoided.
Bibliography
6 Comments
James Ahlborn
The NCCEs which use Properties are bad examples because the Properties class is synchronized (so all the examples are actually equivalent to the compliant example).
David Svoboda
Agreed. I s/Properties/Map/ in the relevant code examples.
Andrey Aniskovets
Does this code safe (java 8)?
David Svoboda
That depends on your definition of 'safe'. Your code isn't subject to the problems of the noncompliant code examples shown here. You could still have a race condition on two threads that call setValueFrom String, since it itself is not synchornized. That said, your enumValue will always point to a consistent object.
Soumya mahata
Here in this following example we are trying to use volatile keyword over a singleton class. Though we have used synchronized at the class level we are getting sonar bug (minor) report as non-compliant code.
Can someone please take a look and tell us if this can be improved to avoid the issue or is it a false-negative scenario ?
Thanks.
David Svoboda
This code does appear to comply with this rule, and also with LCK10-J. Use a correct form of the double-checked locking idiom.
There could still be problems outside this function, perhaps if multiple threads rely on fields within dbProvider.