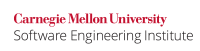
Declaring multiple variables in a single declaration could cause confusion about the types of variables and their initial values. In particular, do not declare any of the following in a single declaration:
- Variables of different types
- A mixture of initialized and uninitialized variables
In general, you should declare each variable on its own line with an explanatory comment regarding its role. While not required for conformance with this guideline, this practice is also recommended in the Code Conventions for the Java Programming Language, §6.1, "Number Per Line" [Conventions 2009].
This guideline applies to
- Local variable declaration statements [JLS 2013, §14.4]
- Field declarations [JLS 2013, §8.3]
- Field (constant) declarations [JLS 2013, §9.3]
Noncompliant Code Example (Initialization)
This noncompliant code example might lead a programmer or reviewer to mistakenly believe that both i
and j
are initialized to 1. In fact, only j
is initialized, while i
remains uninitialized:
int i, j = 1;
Compliant Solution (Initialization)
In this compliant solution, it is readily apparent that both i
and j
are initialized to 1:
int i = 1; // Purpose of i... int j = 1; // Purpose of j...
Compliant Solution (Initialization)
In this compliant solution, it is readily apparent that both i
and j
are initialized to 1:
int i = 1, j = 1;
Declaring each variable on a separate line is the preferred method. However, multiple variables on one line are acceptable when they are trivial temporary variables such as array indices.
Noncompliant Code Example (Different Types)
In this noncompliant code example, the programmer declares multiple variables, including an array, on the same line. All instances of the type T
have access to methods of the Object
class. However, it is easy to forget that arrays require special treatment when some of these methods are overridden.
public class Example<T> { private T a, b, c[], d; public Example(T in) { a = in; b = in; c = (T[]) new Object[10]; d = in; } }
When an Object
method, such as toString()
, is overridden, a programmer could accidentally provide an implementation for type T
that fails to consider that c
is an array of T
rather than a reference to an object of type T
.
public String toString() { return a.toString() + b.toString() + c.toString() + d.toString(); }
However, the programmer's intent could have been to invoke toString()
on each individual element of the array c
.
// Correct functional implementation public String toString(){ String s = a.toString() + b.toString(); for (int i = 0; i < c.length; i++){ s += c[i].toString(); } s += d.toString(); return s; }
Compliant Solution (Different Types)
This compliant solution places each declaration on its own line and uses the preferred notation for array declaration:
public class Example<T> { private T a; // Purpose of a... private T b; // Purpose of b... private T[] c; // Purpose of c[]... private T d; // Purpose of d... public Example(T in){ a = in; b = in; c = (T[]) new Object[10]; d = in; } }
Applicability
Declaration of multiple variables per line can reduce code readability and lead to programmer confusion.
When more than one variable is declared in a single declaration, ensure that both the type and the initial value of each variable are self-evident.
Declarations of loop indices should be included within a for
statement even when this results in variable declarations that lack a comment about the purpose of the variable:
public class Example { void function() { int mx = 100; // Some max value for (int i = 0; i < mx; ++i ) { /* ... */ } } }
Such declarations are not required to be in a separate line, and the explanatory comment may be omitted.
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Parasoft Jtest | 2024.1 | CERT.DCL52.MVOS | Do not declare multiple variables in one statement |
SonarQube | 9.9 | S1659 |
Bibliography
§6.1, "Number Per Line" | |
[ESA 2005] | Rule 9, Put Single Variable Definitions in Separate Lines |
[JLS 2013] | §4.3.2, "The |
3 Comments
David Svoboda
Fred Long
Done.
Robert Seacord (Manager)
should we relax this recommendation to be more like DCL52-J. Do not declare more than one variable per declaration?