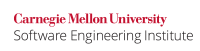
The final
keyword can be used to specify constant values (that is, values that cannot change during program execution). However, constants that can change over the lifetime of a program should not be declared public final. The Java Language Specification (JLS) [JLS 2013] allows implementations to insert the value of any public final field inline in any compilation unit that reads the field. Consequently, if the declaring class is edited so that the new version gives a different value for the field, compilation units that read the public final field could still see the old value until they are recompiled. This problem may occur, for example, when a third-party library is updated to the latest version but the referencing code is not recompiled.
A related error can arise when a programmer declares a static final
reference to a mutable object (see OBJ50-J. Never confuse the immutability of a reference with that of the referenced object for additional information).
Noncompliant Code Example
In this noncompliant code example, class Foo
in Foo.java
declares a field whose value represents the version of the software:
class Foo { public static final int VERSION = 1; // ... }
The field is subsequently accessed by class Bar
from a separate compilation unit (Bar.java
):
class Bar { public static void main(String[] args) { System.out.println("You are using version " + Foo.VERSION); } }
When compiled and run, the software correctly prints
You are using version 1
But if a developer were to change the value of VERSION
to 2 by modifying Foo.java
and subsequently recompile Foo.java
while failing to recompile Bar.java
, the software would incorrectly print
You are using version 1
Although recompiling Bar.java
solves this problem, a better solution is available.
Compliant Solution
According to §13.4.9, "final
Fields and Constants," of the JLS [JLS 2013],
Other than for true mathematical constants, we recommend that source code make very sparing use of class variables that are declared
static
andfinal
. If the read-only nature offinal
is required, a better choice is to declare aprivate static
variable and a suitable accessor method to get its value.
In this compliant solution, the version field in Foo.java
is declared private static and accessed by the getVersion()
method:
class Foo { private static int version = 1; public static final int getVersion() { return version; } // ... }
The Bar
class in Bar.java
is modified to invoke the getVersion()
accessor method to retrieve the version
field from Foo.java
:
class Bar { public static void main(String[] args) { System.out.println( "You are using version " + Foo.getVersion()); } }
In this solution, the private version value cannot be copied into the Bar
class when it is compiled, consequently preventing the bug. Note that this transformation imposes little or no performance penalty because most just-in-time (JIT) code generators can inline the getVersion()
method at runtime.
Applicability
Declaring a value that changes over the lifetime of the software as final may lead to unexpected results.
According to §9.3, "Field (Constant) Declarations," of the JLS [JLS 2013], "Every field declaration in the body of an interface is implicitly public
, static
, and final
. It is permitted to redundantly specify any or all of these modifiers for such fields." Therefore, this guideline does not apply to fields defined in interfaces. Clearly, if the value of a field in an interface changes, every class that implements or uses the interface must be recompiled (see MSC53-J. Carefully design interfaces before releasing them for more information).
Constants declared using the enum
type are permitted to violate this guideline.
Constants whose value never changes throughout the entire lifetime of the software may be declared as final. For instance, the JLS recommends that mathematical constants be declared final.
Bibliography
[JLS 2013] | §4.12.4, " |
6 Comments
Robert Seacord
my brain is getting stuck on the phrase "constants whose value might change". Don't we mean variables?
Robert Seacord
There is some cognitive dissidence between this quote:
we recommend that source code make very sparing use of class variables that are declared
static
andfinal
.and the fact that the compliant solution has class variables that are declared
static
andfinal
.Maybe the quote should be in the NCE section? And then we can explain why we did it anyway?
Fred Long
Hopefully, I've addressed both these comments.
The CS was supposed to follow the advice in the quote and not declare the variable
final
!Yitzhak Mandelbaum
I have some real concerns about this rule. It is basically recommending a hack to work around a (bizarre) shortcoming of the java compiler/linker. We are recommending that programmers take values which are semantically constant – that is, values which are constant with the respect to the current instance of the program (that is, the normal definition of constant) – and refrain from marking them constant because the java compiler can't tell when its linking against outdated bytecode? Or, wrap them in an accessor method which adds clutter and potential for bugs via typos.
Do we have any compelling argument (evidence?) that the consequences of this guideline will be net positive? There is clearly potential for negative impact in that a programmer is forced to choose between "good" code (by writing extra methods) or easy code. I can easily envision programmers dropping final to pass conformance tests rather than go through the hassle and clutter of the recommended solution.
Also, does the JLS guarantee that compilers not inline trivial functions like getVersion? If JITs are free to do so, why wouldn't compilers be?
Is there no alternative to this approach? Can build tools be used to detect the outdated bytecode?
Dhruv Mohindra
So I'm not convinced with this advice at the moment.
James Ahlborn
First of all, i assume that it's merely oversight that Foo.version is not final in the CS (it can be final and the solution will still work just fine).
Second, this is definitely a feasible scenario (compiling against different byte code than that which is used at runtime). this happens frequently when you are compiling against an API which is backed but some separate framework implementation. often, the API has a major version, e.g. 2.0, but the implementation that you actually run with is something like 2.2 (common examples being JAXB and JAX-WS). in this case, the constant inlining could definitely be a problem.