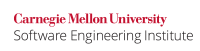
Certain combinations of permissions can produce significant capability increases and should not be granted. Other permissions should be granted only to special code.
AllPermission
The permission java.security.AllPermission
grants all possible permissions to code. This facility was included to reduce the burden of managing a multitude of permissions during routine testing as well as when a body of code is completely trusted. Code is typically granted AllPermission
via the security policy file; it is also possible to programmatically associate AllPermission
with a ProtectionDomain
. This permission is dangerous in production environments. Never grant AllPermission
to untrusted code.
ReflectPermission
, suppressAccessChecks
Granting ReflectPermission
on the target suppressAccessChecks
suppresses all standard Java language access checks when the permitted class attempts to operate on package-private, protected, or private members of another class. Consequently, the permitted class can obtain permissions to examine any field or invoke any method belonging to an arbitrary class [Reflect 2006]. As a result, ReflectPermission
must never be granted with target suppressAccessChecks
.
According to the technical note Permissions in the Java SE 6 Development Kit [Permissions 2008], Section ReflectPermission, target suppressAccessChecks
:
Warning: Extreme caution should be taken before granting this permission to code, for it provides the ability to access fields and invoke methods in a class. This includes not only public, but protected and private fields and methods as well.
RuntimePermission
, createClassLoader
The permission java.lang.RuntimePermission
applied to target createClassLoader
grants code the permission to create a ClassLoader
object. This permission is extremely dangerous because malicious code can create its own custom class loader and load classes by assigning them arbitrary permissions. A custom class loader can define a class (or ProtectionDomain
) with permissions that override any restrictions specified in the systemwide security policy file.
Permissions in the Java SE 6 Development Kit [Permissions 2008] states:
This is an extremely dangerous permission to grant. Malicious applications that can instantiate their own class loaders could then load their own rogue classes into the system. These newly loaded classes could be placed into any protection domain by the class loader, thereby automatically granting the classes the permissions for that domain.
Noncompliant Code Example (Security Policy File)
This noncompliant example grants AllPermission
to the klib
library:
// Grant the klib library AllPermission grant codebase "file:${klib.home}/j2se/home/klib.jar" { permission java.security.AllPermission; };
The permission itself is specified in the security policy file used by the security manager. Program code can obtain a permission object by subclassing the java.security.Permission
class or any of its subclasses (BasicPermission
, for example). The code can use the resulting object to grant AllPermission
to a ProtectionDomain
.
Compliant Solution
This compliant solution shows a policy file that can be used to enforce fine-grained permissions:
grant codeBase "file:${klib.home}/j2se/home/klib.jar", signedBy "Admin" { permission java.io.FilePermission "/tmp/*", "read"; permission java.io.SocketPermission "*", "connect"; };
To check whether the caller has the requisite permissions, standard Java APIs use code such as the following:
// Security manager check FilePermission perm = new java.io.FilePermission("/tmp/JavaFile", "read"); AccessController.checkPermission(perm); // ...
Always assign appropriate permissions to code. Define custom permissions when the granularity of the standard permissions is insufficient.
Noncompliant Code Example (PermissionCollection
)
This noncompliant code example shows an overridden getPermissions()
method, defined in a custom class loader. It grants java.lang.ReflectPermission
with target suppressAccessChecks
to any class that it loads.
protected PermissionCollection getPermissions(CodeSource cs) { PermissionCollection pc = super.getPermissions(cs); pc.add(new ReflectPermission("suppressAccessChecks")); // Permission to create a class loader // Other permissions return pc; }
Compliant Solution
This compliant solution does not grant java.lang.ReflectPermission
with target suppressAccessChecks
to any class that it loads:
protected PermissionCollection getPermissions(CodeSource cs) { PermissionCollection pc = super.getPermissions(cs); // Other permissions return pc; }
Exceptions
ENV03-J-EX0: It may be necessary to grant AllPermission
to trusted library code so that callbacks work as expected. For example, it is common practice, and acceptable, to grant AllPermission
to the optional Java packages (extension libraries):
// Standard extensions extend the core platform and are granted all permissions by default grant codeBase "file:$java.ext.dirs/*" { permission java.security.AllPermission; };
Risk Assessment
Granting AllPermission
to untrusted code allows it to perform privileged operations.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
ENV03-J | High | Likely | Low | P27 | L1 |
Automated Detection
Static detection of potential uses of dangerous permissions is a trivial search. Automated determination of the correctness of such uses is not feasible.
Tool | Version | Checker | Description |
---|---|---|---|
CodeSonar | 9.0p0 | JAVA.IO.PERM | Permissive File Mode (Java) |
Related Vulnerabilities
CVE-2007-5342 describes a vulnerability in Apache Tomcat 5.5.9 through 5.5.25 and 6.0.0 through 6.0.15. The security policy used in the JULI logging component failed to restrict certain permissions for web applications. An attacker could modify the log level, directory, or prefix attributes in the org.apache.juli.FileHandler
handler, permitting them to modify logging configuration options and overwrite arbitrary files.
Related Guidelines
Android Implementation Details
The java.security
package exists on Android for compatibility purposes only, and it should not be used. Android uses another permission mechanism for security purposes.
Bibliography
[API 2014] | Class |
Section 2.5, "Reflection" | |
Section " | |
6 Comments
Thomas Hawtin
Note that, due to extensive use of callbacks, library code needs permissions at least as liberal as application code it is used with. This relationship is transitive, so that libraries used by other libraries have the same relationship. If you don't know what permissions the application code will have, then unfortunately you need to go with all permissions for libraries.
Fred Long
I have changed the title, made some edits, and added an exception to the rule.
I hope that addresses your concerns.
Carl Antuar
I'm currently working on a custom SecurityManager implementation that could provide a solution to this issue. It will introduce the idea of 'guest pass' permissions, that allow a class to be present on the call stack iff there is also a class on the stack that holds the real permission.
So, instead of granting AllPermission to the library, you could grant it GuestPass(AllPermission). Then, if the application code has permission to perform an action, the guest pass for the library will be honored, and the action will succeed; but if the library tries to take an action without the application code present (which might happen due to, eg, crafted input from a remote attacker), then the guest pass by itself will not be enough and the action will fail.
This is particularly relevant for frameworks that appear on the stack before application code, such as Struts and Spring - or the application server itself, for that matter.
Code is early stages yet, but is on GitHub under ThrawnCA/security-manager.git
Chris Sammut
Hi,
I am a student at the university of Malta and I am working on my thesis which is a Firefox extension. I am using Liveconnect to connect java and javascript and need to give my jar file all permissions. How can I do this?
I am using Netbeans and wish to set it to no security. can I do this in the Netbeans IDE?
Thanks
Chris
Dhruv Mohindra
Chris, we only focus on Core Java SE 6 in this standard so we usually don't address specifics (I guess Sun is working on the js/Java bridge now; it's within J2SE). I pulled out the documentation for Liveconnect (link here) and as you probably note, section 2.8 says that a default sandboxing security model applies here.
This thread has more information on what you are trying to achieve with Firefox. The last reply from 'kbr' in particular. That said, I am not recommending you grant AllPermission to external code as this guideline explains. In any case, it is better to create a policy file and grant specific permissions when the sandboxing rules are too tight.
Dhruv Mohindra
This question needs some more justice, though I don't want to provide javascript code (examples and discussion of which can be found at this SUN forums thread, reply 27 is a summary). I have two observations:
1. At first thought, it seems granting AllPermission to a FFox extension may be ok. However, this means that a user who does not trust your extension cannot use it. If a user does not want the extension to read the disk, for example, and thus defines a custom policy which your extension does not satisfy - bad luck.
2. A more serious issue is of trusted code running together with untrusted code. If one includes javascript from untrusted sources, you can imagine the result if the malicious source of javascript misuses the permissions. More so, if the javascript in the Ffox extension itself sets the policy permissions (writing secure javascript is beyond this standard's scope). Consider one example. From past experience coding Ffox extensions, I recall that you need to have global identifiers with unique names as they are shared between different Ffox extensions. An untrusted extension may be able to misuse the trusted jar file, more so if there are problems with the javascript code.
The description at XqueryUseMe ffox extension description known to be the latest compatible extension makes me even more suspicious:
If there is doubt about what can be trusted and untrusted Ffox extensions, a simple way to find out is to read this recent article.
In summary, even if AllPermission applies to a trusted codebase for an extension, it may be possible to write insecure javascript that interacts with other malicious extensions. Thus, IMO it is better to grant AllPermission only for testing or for library code.