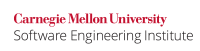
Exceptions that are thrown while logging is in progress can prevent successful logging unless special care is taken. Failure to account for exceptions during the logging process can cause security vulnerabilities, such as allowing an attacker to conceal critical security exceptions by preventing them from being logged. Consequently, programs must ensure that data logging continues to operate correctly even when exceptions are thrown during the logging process.
Noncompliant Code Example
This noncompliant code example writes a critical security exception to the standard error stream:
try { // ... } catch (SecurityException se) { System.err.println(se); // Recover from exception }
Writing such exceptions to the standard error stream is inadequate for logging purposes. First, the standard error stream may be exhausted or closed, preventing recording of subsequent exceptions. Second, the trust level of the standard error stream may be insufficient for recording certain security-critical exceptions or errors without leaking sensitive information. If an I/O error were to occur while writing the security exception, the catch
block would throw an IOException
and the critical security exception would be lost. Finally, an attacker may disguise the exception so that it occurs with several other innocuous exceptions.
Using Console.printf()
, System.out.print*()
, or Throwable.printStackTrace()
to output a security exception also constitutes a violation of this rule.
Compliant Solution
This compliant solution uses java.util.logging.Logger
, the default logging API provided by JDK 1.4 and later. Use of other compliant logging mechanisms, such as log4j, is also permitted.
try { // ... } catch(SecurityException se) { logger.log(Level.SEVERE, se); // Recover from exception }
Typically, only one logger is required for the entire program.
Risk Assessment
Exceptions thrown during data logging can cause loss of data and can conceal security problems.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
ERR02-J | Medium | Likely | High | P6 | L2 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
CodeSonar | 9.0p0 | JAVA.DEBUG.LOG | Debug Warning (Java) |
Parasoft Jtest | 2024.2 | CERT.ERR02.SIO | Avoid calling print methods of 'System.err' or 'System.out' |
SonarQube | 9.9 | S106 | Standard outputs should not be used directly to log anything |
Related Vulnerabilities
HARMONY-5981 describes a vulnerability in the HARMONY implementation of Java. In this implementation, the FileHandler
class can receive log messages, but if one thread closes the associated file, a second thread will throw an exception when it tries to log a message.
10 Comments
David Svoboda
The NCCE/CCE pair essentially mandate that log files should not be opened during a logging operation, but should be opened prior to logging, in order to prevent an IOException from preventing the original error from being logged.
This example occurs in some of the C FIO rules, which warn against re-opening a file stream, but the rule does waranat that this is sometimes necessary. For instance a long-running process may wish not to keep an open file descriptor permenantly, but open the log only when appending a message. This also allows an external daemon to rotate log files.
It may be worthwhile to enhance the CCE to emphasize that we are not advocating against re-opening files (at least not in THIS rule, anyway
Dhruv Mohindra
Amusing Comment! I added this to the CS - "Note that this recommendation does not prevent a program from reopening a closed log file after it realizes that important data must be captured. While an IOException is possible, there is little one can do when writing the data to the log file is itself under question."
Dhruv Mohindra
Any denial of service vulnerability during logging has the same consequences. This item is meant to disallow an attacker from circumventing logging and might need to get a broader name with more examples.
Dhruv Mohindra
A very simple violation:
David Svoboda
The NCCE seems overly complex, and violates ERR04-J. Do not exit abruptly from a finally block, and ERR13-J. Do not throw RuntimeException, and probably other rules. I suspect this rule is a variant of ERR05-J. Do not let checked exceptions escape from a finally block.
There are some regions that must not throw exceptions. I'd guess finalizers are one, and the only reason we have no such rule is because we have a rule against finalizers.
I think we should void this rule; anyone who wishes to preserve it should provide a simpler NCCE that violates no other rules...comments?
Robert Seacord (Manager)
I don't understand what this is an exception to and I think it should simply be deleted:
James Ahlborn
I'm confused by this rule. The examples presented here seem totally unrelated to the rule itself. the rule is addressing how to handle exceptions generated while logging. the examples presented seem to address whether you should use System.err or a logging framework for adequate logging.
Yitzhak Mandelbaum
Agreed -- the text of the rule is broken and needs to be cleaned up. Its on our list.
Henry Wang
The standard error stream or standard output stream may lead to exceptions, which means any use of the standard output is noncompiant.
So is this code snippet a violation of this rule?
David Svoboda
Read the text associated with noncompliant code example. The problem is not that printing to standard output or standard error can produce their own exceptions, there are several potential problems with using either output stream for error handling, such that they might be closed/ignored.
Your code snippet does not violate this rule, but whether it is a good idea or not depends on the role standard output & standard error play in your program.