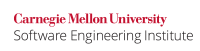
Invocation of System.exit()
terminates the Java Virtual Machine (JVM), consequently terminating all running programs and threads. This can result in denial-of-service (DoS) attacks. For example, a call to System.exit()
that is embedded in Java Server Pages (JSP) code can cause a web server to terminate, preventing further service for users. Programs must prevent both inadvertent and malicious calls to System.exit()
. Additionally, programs should perform necessary cleanup actions when forcibly terminated (for example, by using the Windows Task Manager, POSIX kill
command, or other mechanisms).
Noncompliant Code Example
This noncompliant code example uses System.exit()
to forcefully shut down the JVM and terminate the running process. The program lacks a security manager; consequently, it lacks the capability to check whether the caller is permitted to invoke System.exit()
.
public class InterceptExit { public static void main(String[] args) { // ... System.exit(1); // Abrupt exit System.out.println("This never executes"); } }
Compliant Solution
This compliant solution installs a custom security manager PasswordSecurityManager
that overrides the checkExit()
method defined in the SecurityManager
class. This override is required to enable invocation of cleanup code before allowing the exit. The default checkExit()
method in the SecurityManager
class lacks this facility.
class PasswordSecurityManager extends SecurityManager { private boolean isExitAllowedFlag; public PasswordSecurityManager(){ super(); isExitAllowedFlag = false; } public boolean isExitAllowed(){ return isExitAllowedFlag; } @Override public void checkExit(int status) { if (!isExitAllowed()) { throw new SecurityException(); } super.checkExit(status); } public void setExitAllowed(boolean f) { isExitAllowedFlag = f; } } public class InterceptExit { public static void main(String[] args) { PasswordSecurityManager secManager = new PasswordSecurityManager(); System.setSecurityManager(secManager); try { // ... System.exit(1); // Abrupt exit call } catch (Throwable x) { if (x instanceof SecurityException) { System.out.println("Intercepted System.exit()"); // Log exception } else { // Forward to exception handler } } // ... secManager.setExitAllowed(true); // Permit exit // System.exit() will work subsequently // ... } }
This implementation uses an internal flag to track whether the exit is permitted. The method setExitAllowed()
sets this flag. The checkExit()
method throws a SecurityException
when the flag is unset (that is, false). Because this flag is not initially set, normal exception processing bypasses the initial call to System.exit()
. The program catches the SecurityException
and performs mandatory cleanup operations, including logging the exception. The System.exit()
method is enabled only after cleanup is complete.
Exceptions
ERR09-J-EX0: It is permissible for a command-line utility to call System.exit()
, for example, when the required number of arguments are not input [Bloch 2008], [ESA 2005].
Risk Assessment
Allowing unauthorized calls to System.exit()
may lead to denial of service.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
ERR09-J | Low | Unlikely | Medium | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
CodeSonar | 8.1p0 | JAVA.DEBUG.CALL | Debug Call (Java) |
Coverity | 7.5 | DC.CODING_STYLE | Implemented |
Parasoft Jtest | 2024.1 | CERT.ERR09.JVM CERT.ERR09.EXIT | Do not stop the JVM in a web component Do not call methods which terminates Java Virtual Machine |
SonarQube | 9.9 | S1147 | Exit methods should not be called |
Related Guidelines
Android Implementation Details
On Android, System.exit()
should not be used because it will terminate the virtual machine abruptly, ignoring the activity life cycle, which may prevent proper garbage collection.
Bibliography
[API 2014] | Method |
Section 9.5, "The Finalize Method" | |
[ESA 2005] | Rule 78, Restrict the use of the |
Section 7.4, "JVM Shutdown" | |
Chapter 16, "Intercepting a Call to |
8 Comments
Dhruv Mohindra
Some discussion here about this one.
David Svoboda MGR
I suspect there are more cases where invoking System.exit() is permissible:
* To prevent an info-disclosure or code-execution attack. That is, if the system suspects it is being attacked and might have repercussions worse thandenial of service, it can shut down.
* When the program detects a bug in itself (assertion failures)
* when the input data fed to the program is invalid (this is a generalization of EX1)
Others?
David Svoboda
While interesting, I don't think the code examples support the rule.
The first NCCE/CS pair seems to say "prevent untrusted code from exiting". (Which is a surprise since I expected "don't call System.exit()"). The problem arises when a library needs to exit for various reasons (see my previous comment). In these cases aborting becomes a less dire consequence then letting execution proceed and possibly getting pwned.
The second NCCE/CS pair seems to say "handle signals from outside gracefully". Not sure about Java, but WG14 (C) decided that the only thing a signal handler can do safely is set a flag (indicating the signal arrived) and return. IOW I'm not sure there's anything useful you can do inside the hookShutdown() method.
Right now I'm inclined to void this rule, although if either example can prevail they can save the rule (or a rule promiting the CS).
Robert Seacord (Manager)
According to the API (http://download.oracle.com/javase/6/docs/api/java/lang/System.html#exit%28int%29)
The call
System.exit
is effectively equivalent to the call:Perhaps the title of this rule should be "Only trusted code must be allowed to terminate the JVM" and we should add this point that there are other ways to do it.
David Svoboda
Wordsmithed the title
Thomas Hawtin
WebStart allows the JVM to be terminated by untrusted code.
David Svoboda
Is this by design? Or considered a bug? I presume JWS employs a security manager...does the manager not prevent a call to System.exit()?
If this ability is considered a good thing, perhaps we should expand ERRO9-EX0 to include JWS apps.
Thomas Hawtin
Java WebStart (jaws) uses a process per application. Calling System.exit ensures that the application really quits, without random non-daemon threads keeping it alive. This is specified in the JNLP spec.
I believe there exist JNLP implementations the share a process between applications. These should still allow System.exit, but presumably try to shutdown the application differently - stopping application threads, for instance.