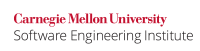
The assert
statement is a convenient mechanism for incorporating diagnostic tests in code. The behavior of the assert
statement depends on the status of a runtime property. When enabled, the assert
statement evaluates its expression argument and throws an AssertionError
if false. When disabled, assert
is a no-op; any side effects resulting from evaluation of the expression in the assertion are lost. Consequently, expressions used with the standard assert
statement must not produce side effects.
Noncompliant Code Example
This noncompliant code attempts to delete all the null names from the list in an assertion. However, the Boolean expression is not evaluated when assertions are disabled.
private ArrayList<String> names; void process(int index) { assert names.remove(null); // Side effect // ... }
Compliant Solution
The possibility of side effects in assertions can be avoided by decoupling the Boolean expression from the assertion:
private ArrayList<String> names; void process(int index) { boolean nullsRemoved = names.remove(null); assert nullsRemoved; // No side effect // ... }
Risk Assessment
Side effects in assertions result in program behavior that depends on whether assertions are enabled or disabled.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
EXP06-J | Low | Unlikely | Low | P3 | L3 |
Automated Detection
Automated detection of assertion operands that contain locally visible side effects is straightforward. Some analyses could require programmer assistance to determine which method invocations lack side effects.
Tool | Version | Checker | Description |
---|---|---|---|
CodeSonar | 9.0p0 | JAVA.STRUCT.SE.ASSERT | Assertion contains side effects |
Parasoft Jtest | 2024.2 | CERT.EXP06.EASE | Expressions used in assertions must not produce side effects |
PVS-Studio | 7.37 | V6055 | |
SonarQube | 9.9 | S3346 | Expressions used in "assert" should not produce side effects |
Related Guidelines
Android Implementation Details
The assert
statement is supported on the Dalvik VM but is ignored under the default configuration. Assertions may be enabled by setting the system property debug.assert
via: adb shell setprop debug.assert 1
or by sending the command-line argument --enable-assert
to the Dalvik VM.
1 Comment
Thomas Hawtin
assert is a statement in Java. As such it would be normal formatting to insert a space between the keyword and opening paraenthesis, like if/while/for. In fact the parenthesis is not part of the syntax and it is usual not to include it. IMO it is misleading to make it look like a method because, as this rule states, it doesn't act like one.
The predominant style in Java (of which I fully approve) is to always check arguments before using them (but after copying mutables). As such, assert is inappropriate for this usage. Good example of using assert are calls to Thread.holdsLock, java.util.concurrent.locks.ReentrantLock.isHeldByCurrentThread and java.awt.EventQueue.isDispatchThread.