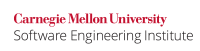
Files on multiuser systems are generally owned by a particular user. The owner of the file can specify which other users on the system should be allowed to access the contents of these files.
These file systems use a privileges and permissions model to protect file access. When a file is created, the file access permissions dictate who may access or operate on the file. When a program creates a file with insufficiently restrictive access permissions, an attacker may read or modify the file before the program can modify the permissions. Consequently, files must be created with access permissions that prevent unauthorized file access.
Noncompliant Code Example
The constructors for FileOutputStream
and FileWriter
do not allow the programmer to explicitly specify file access permissions. In this noncompliant code example, the access permissions of any file created are implementation-defined and may not prevent unauthorized access:
Writer out = new FileWriter("file");
Compliant Solution (Java 1.6 and Earlier)
Java 1.6 and earlier lack a mechanism for specifying default permissions upon file creation. Consequently, the problem must be avoided or solved using some mechanism external to Java, such as by using native code and the Java Native Interface (JNI).
Compliant Solution (POSIX)
The I/O facility java.nio
provides classes for managing file access permissions. Additionally, many of the methods and constructors that create files accept an argument allowing the program to specify the initial file permissions.
The Files.newByteChannel()
method allows a file to be created with specific permissions. This method is platform-independent, but the actual permissions are platform-specific. This compliant solution defines sufficiently restrictive permissions for POSIX platforms:
Path file = new File("file").toPath(); // Throw exception rather than overwrite existing file Set<OpenOption> options = new HashSet<OpenOption>(); options.add(StandardOpenOption.CREATE_NEW); options.add(StandardOpenOption.APPEND); // File permissions should be such that only user may read/write file Set<PosixFilePermission> perms = PosixFilePermissions.fromString("rw-------"); FileAttribute<Set<PosixFilePermission>> attr = PosixFilePermissions.asFileAttribute(perms); try (SeekableByteChannel sbc = Files.newByteChannel(file, options, attr)) { // Write data };
Exceptions
FIO01-J-EX0: When a file is created inside a directory that is both secure and unreadable by untrusted users, that file may be created with the default access permissions. This could be the case if, for example, the entire file system is trusted or is accessible only to trusted users (see FIO00-J. Do not operate on files in shared directories for the definition of a secure directory).
FIO01-J-EX1: Files that do not contain privileged information need not be created with specific access permissions.
Risk Assessment
If files are created without appropriate permissions, an attacker may read or write to the files, possibly resulting in compromised system integrity and information disclosure.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
FIO01-J | Medium | Probable | High | P4 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
CodeSonar | 9.0p0 | JAVA.IO.PERM.ACCESS | Accessing file in permissive mode |
Parasoft Jtest | 2024.2 | CERT.FIO01.ASNF | Avoid implicit file creation when a String is passed as an argument |
PVS-Studio | 7.37 | V5318 |
Related Guidelines
VOID FIO06-CPP. Create files with appropriate access permissions | |
ISO/IEC TR 24772:2010 | Missing or Inconsistent Access Control [XZN] |
CWE-279, Incorrect Execution-Assigned Permissions |
Android Implementation Details
Creating files with weak permissions may allow malicious applications to access the files.
Bibliography
[API 2014] | |
[CVE] | |
Chapter 9, "UNIX 1: Privileges and Files" | |
[OpenBSD] | |
"The | |
Section 2.7, "Restricting Access Permissions for New Files on UNIX" |
12 Comments
David Svoboda
Java provides no Windows analogue of PosixFilePermissions. So on Windows, on an untrusted filesystem, you can't create files with sufficiently secure permissions, even on Java 1.7.
Robert Seacord (Manager)
AclFileAttributeView â“ Supports reading or updating a file's Access Control Lists (ACL). The NFSv4 ACL model is supported. Any ACL model, such as the Windows ACL model, that has a well-defined mapping to the NFSv4 model might also be supported.
http://download.java.net/jdk7/docs/api/java/nio/file/attribute/AclFileAttributeView.html
David Svoboda
I have (for now) removed the Java 1.7, Windows CS.
The Java 1.7 release notes point to the Java Tutorial wrt documentation of new features, especially NIO.
The tutorial has a code sample regarding how to create a file with specific UNIX permissions, but nothing about creating a file with permissions for Windows. (There is, in fact, a code sample on how to create DOS files with specific attributes, but nothing related to permissions.) And Google provides no other info on tweaking 'file permissions' in Windows. (it actually links to FIO03-J first!)
FTM my WinXP VM has no setting as to how to change permissions in a file. I'd guess that Windows XP simply does not provide a permissions capability, at least not to non-developers.
What you are suggesting may be programmable, but certainly does not seem to be intended by Java/Oracle.
David Svoboda
I took another stab at creating a Windows file with a correct ACL. The code I've got so far is this:
But I can't find any mechanism to construct a FileAttribute from an AclEntry. In fact, the only way to construct a FileAttribute seems to be from PosixFilePermissions
Which seems to indicate that you can't create a file with specified permissions on any platform except POSIX. The APIs here seem to let you get/set the permissions of a file on any platform after it has been created. But that defeats the purpose of this rule.
Yozo TODA
how about revising Risk Assessment with the following ?
David Svoboda
Done
vijaya kedar
How can the file permissions be changed in Java 1.5?
David Svoboda
Java 1.6 and earlier lack a mechanism for specifying default permissions upon file creation. Consequently, the problem must be avoided or solved using some mechanism external to Java, such as by using native code and the Java Native Interface (JNI).
Pankaj
File tempFile = new File(getFile(fileName,tmpDir));
How can 'Incorrect Permission Assignment for Critical Resource' be solved using Java 8 in this scenario
David Svoboda
The same way is is solved in Java 7...see the POSIX compliant solution for details.
Yariv Tal
Last discussion on creating a file with correct permissions on Windows was way back in 2011 (see above comments) with the conclusion that the Java API does not support this even after 1.7.
Is there any information on how to achieve this today?
Is it possible to create a file with appropriate permissions in modern Java?
If so - a sample compliant solution should be added.
David Svoboda
A quick perusal of the Java 17 docs leads me to think there is still no secure way to create a file with minimal file permission in Windows. The API docs and the Java Secure Coding Guidelines are both silent on the matter.