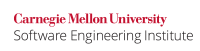
Composition or inheritance may be used to create a new class that both encapsulates an existing class and adds one or more fields. When one class extends another in this way, the concept of equality for the subclass may or may not involve its new fields. That is, when comparing two subclass objects for equality, sometimes their respective fields must also be equal, and other times they need not be equal. Depending on the concept of equality for the subclass, the subclass might override equals()
. Furthermore, this method must follow the general contract for equals()
, as specified by The Java Language Specification (JLS) [JLS 2015].
An object is characterized both by its identity (location in memory) and by its state (actual data). The ==
operator compares only the identities of two objects (to check whether the references refer to the same object); the equals()
method defined in java.lang.Object
can be overridden to compare the state as well. When a class defines an equals()
method, it implies that the method compares state. When the class lacks a customized equals()
method (either locally declared or inherited from a parent class), it uses the default Object.equals()
implementation inherited from Object
. The default Object.equals()
implementation compares only the references and may produce unexpected results.
The equals()
method applies only to objects, not to primitives.
Enumerated types have a fixed set of distinct values that may be compared using ==
rather than the equals()
method. Note that enumerated types provide an equals()
implementation that uses ==
internally; this default cannot be overridden. More generally, subclasses that both inherit an implementation of equals()
from a superclass and lack a requirement for additional functionality need not override the equals()
method.
The general usage contract for equals()
, as specified by the JLS, establishes five requirements:
- It is reflexive: For any reference value
x
,x.equals(x)
must return true. - It is symmetric: For any reference values
x
andy
,x.equals(y)
must return true if and only ify.equals(x)
returns true. - It is transitive: For any reference values
x
,y
, andz
, ifx.equals(y)
returns true andy.equals(z)
returns true, thenx.equals(z)
must return true. - It is consistent: For any reference values
x
andy
, multiple invocations ofx.equals(y)
consistently return true or consistently return false, provided no information used inequals()
comparisons on the object is modified. - For any non-null reference value
x
,x.equals(null)
must return false.
Never violate any of these requirements when overriding the equals()
method.
Noncompliant Code Example (Symmetry)
This noncompliant code example defines a CaseInsensitiveString
class that includes a String
and overrides the equals()
method. The CaseInsensitiveString
class knows about ordinary strings, but the String
class has no knowledge of case-insensitive strings. Consequently, the CaseInsensitiveString.equals()
method should not attempt to interoperate with objects of the String
class.
public final class CaseInsensitiveString { private String s; public CaseInsensitiveString(String s) { if (s == null) { throw new NullPointerException(); } this.s = s; } // This method violates symmetry public boolean equals(Object o) { if (o instanceof CaseInsensitiveString) { return s.equalsIgnoreCase(((CaseInsensitiveString)o).s); } if (o instanceof String) { return s.equalsIgnoreCase((String)o); } return false; } // Comply with MET09-J public int hashCode() {/* ... */} public static void main(String[] args) { CaseInsensitiveString cis = new CaseInsensitiveString("Java"); String s = "java"; System.out.println(cis.equals(s)); // Returns true System.out.println(s.equals(cis)); // Returns false } }
By operating on String
objects, the CaseInsensitiveString.equals()
method violates the second contract requirement (symmetry). Because of the asymmetry, given a String
object s
and a CaseInsensitiveString
object cis
that differ only in case, cis.equals(s))
returns true, while s.equals(cis)
returns false.
Compliant Solution
In this compliant solution, the CaseInsensitiveString.equals()
method is simplified to operate only on instances of the CaseInsensitiveString
class, consequently preserving symmetry:
public final class CaseInsensitiveString { private String s; public CaseInsensitiveString(String s) { if (s == null) { throw new NullPointerException(); } this.s = s; } public boolean equals(Object o) { return o instanceof CaseInsensitiveString && ((CaseInsensitiveString)o).s.equalsIgnoreCase(s); } public int hashCode() {/* ... */} public static void main(String[] args) { CaseInsensitiveString cis = new CaseInsensitiveString("Java"); String s = "java"; System.out.println(cis.equals(s)); // Returns false now System.out.println(s.equals(cis)); // Returns false now } }
Noncompliant Code Example (Transitivity)
This noncompliant code example defines an XCard
class that extends the Card
class.
public class Card { private final int number; public Card(int number) { this.number = number; } public boolean equals(Object o) { if (!(o instanceof Card)) { return false; } Card c = (Card)o; return c.number == number; } public int hashCode() {/* ... */} } class XCard extends Card { private String type; public XCard(int number, String type) { super(number); this.type = type; } public boolean equals(Object o) { if (!(o instanceof Card)) { return false; } // Normal Card, do not compare type if (!(o instanceof XCard)) { return o.equals(this); } // It is an XCard, compare type as well XCard xc = (XCard)o; return super.equals(o) && xc.type == type; } public int hashCode() {/* ... */} public static void main(String[] args) { XCard p1 = new XCard(1, "type1"); Card p2 = new Card(1); XCard p3 = new XCard(1, "type2"); System.out.println(p1.equals(p2)); // Returns true System.out.println(p2.equals(p3)); // Returns true System.out.println(p1.equals(p3)); // Returns false // violating transitivity } }
In the noncompliant code example, p1
and p2
compare equal and p2
and p3
compare equal, but p1
and p3
compare unequal, violating the transitivity requirement. The problem is that the Card
class has no knowledge of the XCard
class and consequently cannot determine that p2
and p3
have different values for the field type
.
Compliant Solution (Delegation)
Unfortunately, in this case it is impossible to extend the Card
class by adding a value or field in the subclass while preserving the Java equals()
contract. This problem is not specific to the Card class but applies to any class hierarchy that can consider equal instances of distinct subclasses of some superclass. For such cases, use composition rather than inheritance to achieve the desired effect [Bloch 2008], [Liskov 1994], [Cline, C++ Super-FAQ]. It is fundamentally impossible to have a class that both allows arbitrary subclass extensions and permits an equals()
method that is reflexive, symmetric, and transitive, as is required by Object.equals()
. In the interests of consistency and security, we forgo arbitrary subclass extensions, and assume that {{Card.equals()}} may impose certain restrictions on its subclasses.
This compliant solution adopts this approach by adding a private card
field to the XCard
class and providing a public viewCard()
method.
class XCard { private String type; private Card card; // Composition public XCard(int number, String type) { card = new Card(number); this.type = type; } public Card viewCard() { return card; } public boolean equals(Object o) { if (!(o instanceof XCard)) { return false; } XCard cp = (XCard)o; return cp.card.equals(card) && cp.type.equals(type); } public int hashCode() {/* ... */} public static void main(String[] args) { XCard p1 = new XCard(1, "type1"); Card p2 = new Card(1); XCard p3 = new XCard(1, "type2"); XCard p4 = new XCard(1, "type1"); System.out.println(p1.equals(p2)); // Prints false System.out.println(p2.equals(p3)); // Prints false System.out.println(p1.equals(p3)); // Prints false System.out.println(p1.equals(p4)); // Prints true } }
Compliant Solution (Class Comparison)
If the Card.equals()
method could unilaterally assume that two objects with distinct classes were not equal, it could be used in an inheritance hierarchy while preserving transitivity:
public class Card { private final int number; public Card(int number) { this.number = number; } public boolean equals(Object o) { if (!(o.getClass() == this.getClass())) { return false; } Card c = (Card)o; return c.number == number; } public int hashCode() {/* ... */} }
Noncompliant Code Example (Consistency)
A uniform resource locator (URL) specifies both the location of a resource and a method to access it. According to the Java API documentation for Class URL
[API 2014]:
Two URL objects are equal if they have the same protocol, reference equivalent hosts, have the same port number on the host, and the same file and fragment of the file.
Two hosts are considered equivalent if both host names can be resolved into the same IP addresses; else if either host name can't be resolved, the host names must be equal without regard to case; or both host names equal to null.
The defined behavior for the equals()
method is known to be inconsistent with virtual hosting in HTTP.
Virtual hosting allows a web server to host multiple websites on the same computer, sometimes sharing the same IP address. Unfortunately, this technique was unanticipated when the URL
class was designed. Consequently, when two completely different URLs resolve to the same IP address, the URL
class considers them to be equal.
Another risk associated with the equals()
method for URL
objects is that the logic it uses when connected to the Internet differs from that used when disconnected. When connected to the Internet, the equals()
method follows the steps described in the Java API; when disconnected, it performs a string compare on the two URLs. Consequently, the URL.equals()
method violates the consistency requirement for equals()
.
Consider an application that allows an organization's employees to access an external mail service via http://mailwebsite.com
. The application is designed to deny access to other websites by behaving as a makeshift firewall. However, a crafty or malicious user could nevertheless access an illegitimate website http://illegitimatewebsite.com
if it were hosted on the same computer as the legitimate website and consequently shared the same IP address. Even worse, if the legitimate website were hosted on a server in a commercial pool of servers, an attacker could register multiple websites in the pool (for phishing purposes) until one was registered on the same computer as the legitimate website, consequently defeating the firewall.
public class Filter { public static void main(String[] args) throws MalformedURLException { final URL allowed = new URL("http://mailwebsite.com"); if (!allowed.equals(new URL(args[0]))) { throw new SecurityException("Access Denied"); } // Else proceed } }
Compliant Solution (Strings)
This compliant solution compares the string representations of two URLs, thereby avoiding the pitfalls of URL.equals()
:
public class Filter { public static void main(String[] args) throws MalformedURLException { final URL allowed = new URL("http://mailwebsite.com"); if (!allowed.toString().equals(new URL(args[0]).toString())) { throw new SecurityException("Access Denied"); } // Else proceed } }
This solution still has problems. Two URLs with different string representation can still refer to the same resource. However, the solution fails safely in this case because the equals()
contract is preserved, and the system will never allow a malicious URL to be accepted by mistake.
Compliant Solution (URI.equals()
)
A Uniform Resource Identifier (URI) contains a string of characters used to identify a resource; this is a more general concept than an URL. The java.net.URI
class provides string-based equals()
and hashCode()
methods that satisfy the general contracts for Object.equals()
and Object.hashCode()
; they do not invoke hostname resolution and are unaffected by network connectivity. URI
also provides methods for normalization and canonicalization that URL
lacks. Finally, the URL.toURI()
and URI.toURL()
methods provide easy conversion between the two classes. Programs should use URIs instead of URLs whenever possible. According to the Java API Class URI
documentation [API 2014]:
A
URI
may be either absolute or relative. AURI
string is parsed according to the generic syntax without regard to the scheme, if any, that it specifies. No lookup of the host, if any, is performed, and no scheme-dependent stream handler is constructed.
This compliant solution uses a URI
object instead of a URL
. The filter appropriately blocks the website when presented with any string other than http://mailwebsite.com
because the comparison fails.
public class Filter { public static void main(String[] args) throws MalformedURLException, URISyntaxException { final URI allowed = new URI("http://mailwebsite.com"); if (!allowed.equals(new URI(args[0]))) { throw new SecurityException("Access Denied"); } // Else proceed } }
Additionally, the URI
class performs normalization (removing extraneous path segments such as "..
") and relativization of paths [API 2014], [Darwin 2004].
Noncompliant Code Example (java.security.Key
)
The method java.lang.Object.equals()
by default is unable to compare composite objects such as cryptographic keys. Most Key
classes lack an equals()
implementation that would override Object
's default implementation. In such cases, the components of the composite object must be compared individually to ensure correctness.
This noncompliant code example compares two keys using the equals()
method. The comparison may return false even when the key instances represent the same logical key.
private static boolean keysEqual(Key key1, Key key2) { if (key1.equals(key2)) { return true; } return false; }
Compliant Solution (java.security.Key
)
This compliant solution uses the equals()
method as a first test and then compares the encoded version of the keys to facilitate provider-independent behavior. For example, this code can determine whether a RSAPrivateKey
and RSAPrivateCrtKey
represent equivalent private keys [Sun 2006].
private static boolean keysEqual(Key key1, Key key2) { if (key1.equals(key2)) { return true; } if (Arrays.equals(key1.getEncoded(), key2.getEncoded())) { return true; } // More code for different types of keys here. // For example, the following code can check if // an RSAPrivateKey and an RSAPrivateCrtKey are equal: if ((key1 instanceof RSAPrivateKey) && (key2 instanceof RSAPrivateKey)) { if ((((RSAKey)key1).getModulus().equals( ((RSAKey)key2).getModulus())) && (((RSAPrivateKey) key1).getPrivateExponent().equals( ((RSAPrivateKey) key2).getPrivateExponent()))) { return true; } } return false; }
Exceptions
MET08-J-EX0: Requirements of this rule may be violated provided that the incompatible types are never compared. There are classes in the Java platform libraries (and elsewhere) that extend an instantiable class by adding a value component. For example, java.sql.Timestamp
extends java.util.Date
and adds a nanoseconds field. The equals()
implementation for Timestamp
violates symmetry and can cause erratic behavior when Timestamp
and Date
objects are used in the same collection or are otherwise intermixed [Bloch 2008].
Risk Assessment
Violating the general contract when overriding the equals()
method can lead to unexpected results.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MET08-J | Low | Unlikely | Medium | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
CodeSonar | 8.1p0 | JAVA.COMPARE.CTO.ASSYM | Asymmetric compareTo (Java) |
Parasoft Jtest | 2023.1 | CERT.MET08.EQREFL | Make sure implementation of Object.equals(Object) is reflexive |
SonarQube | 9.9 | S2162 | "equals" methods should be symmetric and work for subclasses |
Related Guidelines
Bibliography
[API 2014] | Class URI Class URL (method equals() ) |
Item 8, "Obey the General Contract When Overriding | |
[Cline, C++ Super-FAQ] | |
Section 9.2, "Overriding the | |
Chapter 3, "Classes, Strings, and Arrays," section "The Object Class (Equality)" | |
[Liskov 1994] | Liskov, B. H.; Wing, J. M. (November 1994). A behavioral notion of subtyping. ACM Trans. Program. Lang. Syst.16 (6). pp. 1811–1841. doi:10.1145/197320.197383. An updated version appeared as CMU technical report: Liskov, Barbara; Wing, Jeannette (July 1999). "Behavioral Subtyping Using Invariants and Constraints" (PS). |
[Sun 2006] | Determining If Two Keys Are Equal (JCA Reference Guide) |
"More Joy of Sets" |
23 Comments
David Svoboda
Doesn't this rule also apply to all other common methods, like Object.hashCode()?
Dhruv Mohindra
This rule can be described as a fine grained rule: particular to equals(). For the hashcode contract we have 3 points to consider according to the spec. The first and the last are usually not violated (they can be discussed in the hashcode rec though) and for the middle one we have MET13-J. Ensure that hashCode() is overridden when equals() is overridden. In short, this rule doesn't apply to other methods. Other methods that are susceptible to abuse should use separate rules IMHO, instead of having a lengthy general guideline on 'follow the contract of methods while overriding'.
Philip Miller
I am with Dhruv on the specific set of 5 axioms that must hold for equals. The first 3 axioms are the mathematical definition of an equivalence class and therefore do not apply to the general case of relational operators of which hashCode() is one. However it would be easy to rewrite MET12-J. to state a broader rule that in overriding methods the programmer should write code that faithfully preserves the general contract. If that is what we believe then we should do it. If not I recommend that we state it is sometimes OK to violate the general contract in the commentary of MET12-J.
Masaki Kubo
The following sentence doesn't make sense with the given condition and the following code example:
David Svoboda
I've embellished the sentence...it pertains to websites hosted by companies like Alexa who rent web space to anyone with $.
Masaki Kubo
Thanks David! Its much clearer now.
Yozo TODA
I think the title of this rule "Ensure objects that are equated are equatable" is too abstract.
I want to re-phrase it to more intuitive one (mainly because I'm working for japanese translation of the standard (-: ).
Here are some candidates, with my understanding on the rule.
Which (or other?) describes better the intention of the rule?
equals()
contract when introducing or extending classesequals()
when introducing or extending classes (similar to MET10-J)David Svoboda
I agree that the old title was kinda abstract. Hopefully the new title is better.
Yozo TODA
thanks!
James Ahlborn
I don't understand this sentence (re. transitivity):
"Unfortunately, in this case it is impossible to extend an instantiable class (as opposed to an
abstract
class) by adding a value or field in the subclass while preserving theequals()
contract."It is perfectly possible to extend instantiable classes and preserve the equals() contract. the most straightforward way to do it is to use "getClass() == o.getClass()" to compare classes instead of instanceof. in fact, this is often a better/safer design choice as it reduces the chances of accidentally creating subclass hierarchies with broken equals() implementations. More details here and here.
David Svoboda
James:
The reason for that statement is that you can program your subclass's equal() method to do whatever you want (including just comparing classes), but you can't change your superclass's equal() method. In our example, we can make Xcard.equals() do anything, but we can't change Card.equals(), and thus we can't prevent someone passing an XCard to Card.equals(). Again, if XCard doesn't have a type field, or we don't care about its type field wrt equality, we're OK. But if we care about the type field in equality, we can't preserve transivity. It's easier to forego inheritence (Xcard is no longer a subclass of Card) than it is to saccrifice transitivity.
James Ahlborn
I certainly understand the problem with the code in question. However, regarding the sentence i quoted, use of the phrase "an instantiable class" (as opposed to saying "the Card class") seems to imply that this is a general problem with all instantiable base classes, when in reality it is only a problem for the example in question (the example Card class). in the majority of cases, developers probably have access to most, if not all, of the classes in the hierarchy, and if they follow the best practice in the equals() method implementation (using getClass() instead of instanceof), then they can easily maintain a hierarchy of classes where the the various classes are individually instantiable and the equals contract is maintained at all levels.
David Svoboda
I've modified the rule, including a compliant solution that uses class comparison like you suggest. I will still assert that preserving transitivity is impossible if your base class contains an equals method that must assume objects of different subclasses might still be equal. But I think that's getting too complicated to add directly to the rule.
Yitzhak Mandelbaum
David, I don't think that James would argue with your assertion. And, I think that the contrast between the two approaches is an important point, so I've added it explicitly to your amended text.
James Ahlborn
I basically agree with both of these last 2 comments. Technically, it is still possible to preserve transitivity and allow subclass equality, but the solution is too complex to include here, rarely needed, and generally superfluous to the intent of this book.
Nelson Christos
Compliant Solution (Class Comparison). In that section we are using getClass() instead of instanceof. This has the effect of equating objects if they have the same implementation class. This would break the Liskov Substitution principle. Would like to know how the getClass method is a compliant solution where infact the delegation one should be right approach
David Svoboda
Nelson:
Interesting point. It deserves further discussion in the Compliant Solution (CS), once we figure out what to add :)
AFAICT the Liskov substitution principle is fundamentally incompatible with preserving transitivity of equals(), and the code example and preceding noncompliant code illustrates this point very well. While a good idea in theory, the Liskov substitution principle does wind up creating problems in OOP, see the Marshall Cline's C++ FAQs for more analysis:
https://isocpp.org/wiki/faq/proper-inheritance
Still, I would agree with you that the Delegation compliant solution is preferrable, because it permits having subclasses of Card with differing values, but they still respect Card.equals() and comply with this rule. That is impossible under Class Comparison CS.
BTW what is the best reference for the Liskov substitution principle? If we mention it in the rule, we should cite a reference, and I suspect this is the best one:
Nelson Christos
The one cited by you would be the best one doi:10.1145/197320.197383.
As for the getClass() implementation, it is prone to issues. Consider there is a subclass ColoredCard which has an attribute color. Now from an equality perspective it would be logical to consider a Card equals based on the number and not the color.
Lets consider an example of a deck of cards. which contain 52 cards (all Card instances).
Set<Card> cards = Set.of(new Card(1),new Card(2).....);
If the client searches the collection to check if a Card with number "1" is present like below, it would result false.
cards.contains(new ColoredCard(1,red)) // returns false as equals only considers same class implementations
However if the same was executed with the instanceof operator, it would have worked fine provided delegation was used maintaining the Liskov rule.
David Svoboda
Nelson:
That was my point. If you want to comply with Liskov, any subclass of Card must respect Card.equals(). If Card.equals() compares only numbers, then you cannot have a ColoredCard.equals() that also mandates that cards are equal only if their colors match. You can have a ColoredCard that merely relies on Card.equals(). This is a reasonable design decision.
Another legit design decision is to have ColoredCard.equals() check for colors (as well as numbers), but segregate ColoredCards from Cards so the transitivity problem never surfaces.
That said, having Card.equals() declare two cards to be distinct if they are distinct types violates Liskov, Which can be a legit design decision. But it prevents a Liskov-compatible class hierarchy.
Nelson Christos
Ok so we both are making the same point
. Hope we can have some change made to the CS for maintaining the Liskov principle.
David Svoboda
I have added some text to the Delegation CS, explaining that this is a general OOD paradox (not specific to Java). While we could add much more, this is a big rabbit hole, and further study should be relegated to references. So I have added references to the Liskov paper, as well as the C++ FAQ.
Sven Biedermann
This rule is broken in SONAR for all Set-Implementations. Here from Set.java:
Here, for instance, the AbstractSet-Implementation:
return false;
if (c.size() != size())
return false;
As a consquence, willing to comply to this rule makes it impossible to construct class hierarchies with different implementations (e.g. for distinct performance-tradeoffs), which are logical equivalent.
So, the SONAR rule implementation is far too strict and should be revised!
David Svoboda
First of all, your documentation and implementation all come from the Java's own Set and AbstractSet classes:
https://docs.oracle.com/javase/7/docs/api/java/util/Set.html#equals(java.lang.Object)
Second, java.util.Set and AbstractSet still fully comply with this rule, as their equals() methods are reflexive, symmetric, transitive, and consistent. They do this by imposing a definition of equals() that you probably cannot override in classes that implement Set<>