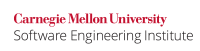
Classes that override the Object.equals()
method must also override the Object.hashCode()
method. The java.lang.Object
class requires that any two objects that compare equal using the equals()
method must produce the same integer result when the hashCode()
method is invoked on the objects [API 2014].
The equals()
method is used to determine logical equivalence between object instances. Consequently, the hashCode()
method must return the same value for all equivalent objects. Failure to follow this contract is a common source of defects.
Noncompliant Code Example
This noncompliant code example associates credit card numbers with strings using a HashMap
and subsequently attempts to retrieve the string value associated with a credit card number. The expected retrieved value is 4111111111111111
; the actual retrieved value is null
.
public final class CreditCard { private final int number; public CreditCard(int number) { this.number = number; } public boolean equals(Object o) { if (o == this) { return true; } if (!(o instanceof CreditCard)) { return false; } CreditCard cc = (CreditCard)o; return cc.number == number; } public static void main(String[] args) { Map<CreditCard, String> m = new HashMap<CreditCard, String>(); m.put(new CreditCard(100), "4111111111111111"); System.out.println(m.get(new CreditCard(100))); } }
The cause of this erroneous behavior is that the CreditCard
class overrides the equals()
method but fails to override the hashCode()
method. Consequently, the default hashCode()
method returns a different value for each object, even though the objects are logically equivalent; these differing values lead to examination of different buckets in the hash table, which prevents the get()
method from finding the intended value.
Note that by specifying the credit card number in main()
, these code examples violate MSC03-J. Never hard code sensitive information for the sake of brevity.
Compliant Solution
This compliant solution overrides the hashCode()
method so that it generates the same value for any two instances that are considered to be equal by the equals()
method. Bloch discusses the recipe to generate such a hash function in detail [Bloch 2008].
public final class CreditCard { private final int number; public CreditCard(int number) { this.number = number; } public boolean equals(Object o) { if (o == this) { return true; } if (!(o instanceof CreditCard)) { return false; } CreditCard cc = (CreditCard)o; return cc.number == number; } public int hashCode() { int result = 17; result = 31 * result + number; return result; } public static void main(String[] args) { Map<CreditCard, String> m = new HashMap<CreditCard, String>(); m.put(new CreditCard(100), "4111111111111111"); System.out.println(m.get(new CreditCard(100))); } }
Risk Assessment
Overriding the equals()
method without overriding the hashCode()
method can lead to unexpected results.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MET09-J | Low | Unlikely | High | P1 | L3 |
Automated Detection
Automated detection of classes that override only one of equals()
and hashcode()
is straightforward. Sound static determination that the implementations of equals()
and hashcode()
are mutually consistent is not feasible in the general case, although heuristic techniques may be useful.
Tool | Version | Checker | Description |
---|---|---|---|
CodeSonar | 8.1p0 | JAVA.IDEF.EQUALSNOHC | Defines equals but not hashCode (Java) |
Parasoft Jtest | 2024.1 | CERT.MET09.OVERRIDE | Override 'Object.hashCode()' when you override 'Object.equals()' and vice versa |
PVS-Studio | 7.31 | V6049 | |
SonarQube | 9.9 | "equals(Object obj)" and "hashCode()" should be overridden in pairs |
Related Guidelines
Bibliography
[API 2014] | |
Item 9, "Always Override |
2 Comments
Masaki Kubo
1. What is "hash bucket"? An entry in a hash table?
2. In the expression:
cast to short seems inappropriate.
David Svoboda
Yes. Tweaked the wording.
Removed the (short). I'd guess it was important a long time ago, but it's useless and confusing now.