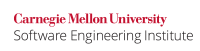
An infinite loop with an empty body consumes CPU cycles but does nothing. Optimizing compilers and just-in-time systems (JITs) are permitted to (perhaps unexpectedly) remove such a loop. Consequently, programs must not include infinite loops with empty bodies.
Noncompliant Code Example
This noncompliant code example implements an idle task that continuously executes a loop without executing any instructions within the loop. An optimizing compiler or JIT could remove the while
loop in this example.
public int nop() { while (true) {} }
Compliant Solution (Thread.sleep()
)
This compliant solution avoids use of a meaningless infinite loop by invoking Thread.sleep()
within the while
loop. The loop body contains semantically meaningful operations and consequently cannot be optimized away.
public final int DURATION=10000; // In milliseconds public void nop() throws InterruptedException { while (true) { // Useful operations Thread.sleep(DURATION); } }
Compliant Solution (yield()
)
This compliant solution invokes Thread.yield()
, which causes the thread running this method to consistently defer to other threads:
public void nop() { while (true) { Thread.yield(); } }
Risk Assessment
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MSC01-J | Low | Unlikely | Medium | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Parasoft Jtest | 2024.2 | CERT.MSC01.EB | Avoid control statements with empty bodies |
SonarQube | 3.10 | S2189 |
Bibliography
[API 2014] |
5 Comments
Unknown User (eazebu)
Should this rule also encompass infinite recursion?
Robert Seacord
This is sort of a confusing rule, and mostly about how to write infinite loops, not how to avoid them. Note that the corresponding C rule was voided. That might be a good direction for this rule as well.
A separate question is if we need rules about infinite loops and infinite recursion.
A Bishop
I agree. while(true) is more the danger sign than the empty loop body. Although I'm also surprised the JIT can remove the whole while loop.
David Svoboda
This rule exists because:
IOW empty infinite loops are a special case in Java due to the idiosyncracies of JIT compilers.
I would guess the overall purpose of this rule is to deal with developers who use an inf. loop to (temporarly) suspend execution. Perhaps the rule should be re-titled to instruct how to suspend execution.
Julian Ospald
This rule is covered in SonarQube java plugin too as S2189.