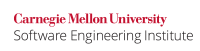
Programming errors can prevent garbage collection of objects that are no longer relevant to program operation. The garbage collector collects only unreachable objects; consequently, the presence of reachable objects that remain unused indicates memory mismanagement. Consumption of all available heap space can cause an OutOfMemoryError
, which usually results in program termination.
Excessive memory leaks can lead to memory exhaustion and denial of service (DoS) and must be avoided (see MSC05-J. Do not exhaust heap space for more information).
Noncompliant Code Example (Off-by-One Programming Error)
The vector
object in the noncompliant code example leaks memory. The condition for removing the vector
element is mistakenly written as n > 0
instead of n >= 0
. Consequently, the method fails to remove one element per invocation and quickly exhausts the available heap space.
public class Leak { static Vector vector = new Vector(); public void useVector(int count) { for (int n = 0; n < count; n++) { vector.add(Integer.toString(n)); } // ... for (int n = count - 1; n > 0; n--) { // Free the memory vector.removeElementAt(n); } } public static void main(String[] args) throws IOException { Leak le = new Leak(); int i = 1; while (true) { System.out.println("Iteration: " + i); le.useVector(1); i++; } } }
Compliant Solution (>=
)
This compliant solution corrects the mistake by changing the loop condition to n >= 0
. It also wraps the cleanup code in a finally
block so that it still executes even if the interim code throws an exception.
public void useVector(int count) { int n = 0; try { for (; n < count; n++) { vector.add(Integer.toString(n)); } // ... } finally { for (n = n - 1; n >= 0; n--) { vector.removeElementAt(n); } } }
Compliant Solution (clear()
)
Prefer the use of standard language semantics where possible. This compliant solution uses the vector.clear()
method, which removes all elements:
public void useVector(int count) { try { for (int n = 0; n < count; n++) { vector.add(Integer.toString(n)); } // ... } finally { vector.clear(); // Clear the vector } }
Noncompliant Code Example (Nonlocal Instance Field)
This noncompliant code example declares and allocates a HashMap
instance field that is used only in the doSomething()
method:
public class Storer { private HashMap<Integer,String> hm = new HashMap<Integer, String>(); private void doSomething() { // hm is used only here and never referenced again hm.put(1, "java"); // ... } }
Programmers may be surprised that the HashMap
persists for the entire lifetime of the Storer
instance.
Compliant Solution (Reduce Scope of Instance Field)
This compliant solution declares the HashMap
as a local variable within the doSomething()
method. The hm
local variable is eliminated after the method returns. When the local variable holds the only reference to the HashMap
, the garbage collector can reclaim its associated storage.
public class Storer { private void doSomething() { HashMap<Integer,String> hm = new HashMap<Integer,String>(); hm.put(1,"java"); // ... } }
Localizing or confining the instance field to a narrower scope simplifies garbage collection; today's generational garbage collectors perform well with short-lived objects.
Noncompliant Code Example (Lapsed Listener)
This noncompliant code example, known as the Lapsed Listener [Goetz 2005a], demonstrates unintentional object retention. The button
continues to hold a reference of the reader
object after completion of the readSomething()
method, even though the reader
object is never used again. Consequently, the garbage collector cannot collect the reader
object. A similar problem occurs with inner classes because they hold an implicit reference to the enclosing class.
public class LapseEvent extends JApplet { JButton button; public void init() { button = new JButton("Click Me"); getContentPane().add(button, BorderLayout.CENTER); Reader reader = new Reader(); button.addActionListener(reader); try { reader.readSomething(); } catch (IOException e) { // Handle exception } } } class Reader implements ActionListener { public void actionPerformed(ActionEvent e) { Toolkit.getDefaultToolkit().beep(); } public void readSomething() throws IOException { // Read from file } }
Noncompliant Code Example (Exception before Remove)
This noncompliant code example attempts to remove the reader
through use of the removeActionListener()
method:
Reader reader = new Reader(); button.addActionListener(reader); try { reader.readSomething(); // Can skip next line of code // Dereferenced, but control flow can change button.removeActionListener(reader); } catch (IOException e) { // Forward to handler }
If an exception is thrown by the readSomething()
method, the removeActionListener()
statement is never executed.
Compliant Solution (finally
Block)
This compliant solution uses a finally
block to ensure that the reader
object's reference is removed:
Reader reader = new Reader(); button.addActionListener(reader); try { reader.readSomething(); } catch (IOException e) { // Handle exception } finally { button.removeActionListener(reader); // Always executed }
Noncompliant Code Example (Member Object Leaks)
This noncompliant code example implements a stack data structure [Bloch 2008] that continues to hold references to elements after they have been popped off the stack:
public class Stack { private Object[] elements; private int size = 0; public Stack(int initialCapacity) { this.elements = new Object[initialCapacity]; } public void push(Object e) { ensureCapacity(); elements[size++] = e; } public Object pop() { // This method causes memory leaks if (size == 0) { throw new EmptyStackException(); } return elements[--size]; } /* * Ensure space for at least one more element, roughly * doubling the capacity each time the array needs to grow. */ private void ensureCapacity() { if (elements.length == size) { Object[] oldElements = elements; elements = new Object[2 * elements.length + 1]; System.arraycopy(oldElements, 0, elements, 0, size); } } }
The object references are retained on the stack even after the element is popped. Such obsolete references cause objects to remain live; consequently, the objects cannot be garbage-collected.
Compliant Solution (null
)
This compliant solution assigns null
to all obsolete references:
public Object pop() { if (size == 0) { throw new EmptyStackException(); // Ensures object consistency } Object result = elements[--size]; elements[size] = null; // Eliminate obsolete reference return result; }
The garbage collector can then include individual objects formerly referenced from the stack in its list of objects to free.
Although these examples appear trivial and do not represent significant problems in production code, obsolete references remain a concern when dealing with data structures such as hash tables containing many large records. It is prudent to assign null
to array-like custom data structures; doing so with individual objects references or local variables is unnecessary because the garbage collector handles these cases automatically [Commes 2007].
Noncompliant Code Example (Strong References)
A common variation of the obsolete object fallacy is the unintentional retention of objects in collections such as maps. In this noncompliant code example, a server maintains temporary metadata about all committed secure connections:
class HashMetaData { private Map<SSLSocket, InetAddress> m = Collections.synchronizedMap( new HashMap<SSLSocket, InetAddress>()); public void storeTempConnection(SSLSocket sock, InetAddress ip) { m.put(sock, ip); } public void removeTempConnection(SSLSocket sock) { m.remove(sock); } }
It is possible to close a socket without removing it from this map. Consequently, this map may contain dead sockets until removeTempConnection()
is invoked on them. In the absence of notification logic, it is impossible to determine when to call removeTempConnection()
. Moreover, nullifying original objects or referents (Socket
connections) is unwieldy.
Compliant Solution (Weak References)
This compliant solution uses weak references to allow timely garbage collection:
// ... private Map<SSLSocket, InetAddress> m = Collections.synchronizedMap( new WeakHashMap<SSLSocket, InetAddress>()); // ...
Strong references prevent the garbage collector from reclaiming objects that are stored inside container objects, such as in a Map
. According to the Java API [API 2014], weak reference objects "do not prevent their referents from being made finalizable, finalized, and then reclaimed."
Keys held in WeakHashMap
objects are referenced through weak references. Objects become eligible for garbage collection when they lack strong references. Consequently, use of weak references allows the code to refer to the referent without delaying garbage collection of the referent. This approach is suitable only when the lifetime of the object is required to be the same as the lifetime of the key.
Simply facilitating garbage collection of unneeded objects through use of weak references is insufficient. Programs must also prune the recording data structure so that additional live entries can be accommodated. The implementation of WeakHashMap
in Java 7 includes a reference queue to efficiently remove entries that correspond to a null pointer value [https://github.com/openjdk-mirror/jdk7u-jdk/blob/master/src/share/classes/java/util/WeakHashMap.java].
Compliant Solution (Soft References)
Use of soft references is also permitted. Soft references guarantee that the referent will be reclaimed before an OutOfMemoryError
occurs and also that the referent will remain live until memory begins to run out.
Reference queues provide notifications when a referent is garbage-collected. When the referent is garbage-collected, the HashMap
continues to strongly reference both the SoftReference
object and the corresponding map value (for each entry in the HashMap
).
When the garbage collector clears the reference to an object, it adds the corresponding SoftReference
object to the reference queue. The SoftReference
object remains in the reference queue until some operation is performed on the queue (such as a poll()
or remove()
). After such an operation, the SoftReference
object in the hash map is also garbage-collected:
class HashMetaData { private Map<SoftReference<SSLSocket>, InetAddress> m = Collections.synchronizedMap( new HashMap<SoftReference<SSLSocket>, InetAddress>()); ReferenceQueue queue = new ReferenceQueue(); public void storeTempConnection(SSLSocket sock, InetAddress ip) { SoftReference<SSLSocket> sr; while ((sr = (SoftReference) queue.poll()) != null) { // Removes the WeakReference object and the value (not the referent) m.remove(sr); } sr = new SoftReference<SSLSocket>(sock, queue); m.put(sr, ip); } // removeTempConnection() deleted, no longer necessary }
Note that the two-argument constructor of SoftReference
takes a Queue
argument and must be used to perform direct queue processing. Dead entries should be pruned prior to insertion.
Weak references are garbage-collected more aggressively than soft references. Consequently, weak references should be preferred in applications in which efficient memory usage is critical, and soft references should be preferred in applications that rely heavily on caching.
Risk Assessment
Memory leaks in Java applications may be exploited in a DoS attack.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MSC04-J | Low | Unlikely | High | P1 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Parasoft Jtest | 2024.2 | CERT.MSC04.LEAKS | Ensure resources are deallocated |
Related Guidelines
ISO/IEC TR 24772:2010 | Memory Leak [XYL] |
CWE-401, Improper Release of Memory before Removing Last Reference ("Memory Leak") |
Bibliography
[API 2014] | |
Item 6, "Eliminate Obsolete Object References" | |
"Memory Leak Avoidance" | |
"Lapsed Listeners" | |
14 Comments
Lothar Kimmeringer
Another compliant solution for removing elements from a vector avoiding the use of counters at all is
Dhruv Mohindra
True, this makes it clearer/less error prone. It has been added as a CS now. Thanks!
Thomas Hawtin
The standard way to remove a range from a list is:
[
|http://java.sun.com/javase/6/docs/api/java/util/List.html#subList(int, int)]
Dhruv Mohindra
Thanks. I added another CS with this one and
vector.clear()
. I will also be adding more NCCE/CS pairs soon to cover more insidious memory leaks.James Ahlborn
The
removeTempConnection()
method in "CS (Reference Queue)" won't actually work since the keys are instances of WeakReference, not instances of SSLSocket. and you can't fix it by just wrapping a WeakReference around the key to remove since WeakReference uses the default Objectequals()
andhashCode()
method implementations. in order to implement that correctly, you need to use a custom subclass of WeakReference which stashes the hashCode of the original object and returns that forhashCode()
as well as an implementation of theequals()
method which tests for reference equality on the WeakReference instances or reference equality on the referents.James Ahlborn
The "CS (Soft References)" is kind of a meaningless example. you would never use a SoftReference as a key because the whole point of a SoftReference is to hold on to something as long as possible (assuming no memory pressure) which is no longer strongly referenced in the hopes that you might want to use it again. however, in the example, since the SoftReference is the key, you could never actually retrieve the referent (since you presumably no longer have a strong reference to it) and therefore never actually retrieve anything from the cache. (granted you could iterate through the map and return the keys, but that kind of defeats the point of a map). SoftReferences are pretty much always used for values.
A Bishop
The first two 'compliant' solutions can still leak memory in exception scenarios. The try / finally (or try with resources) pattern should be used to clean up the vector.
David Svoboda
Wrapped both cleanup code snippets in finally blocks.
Jérôme GUY
Vector is now deprecated (since a long time
)
Even if the logic of the sample is good, we may change the sample using Collection (as abstraction for example).
David Svoboda
java.util.Vector
is not officially deprecated by Sun/Oracle, and I would not criticize code that uses it. It also provides built-in synchronization, which ArrayList does not.Jérôme GUY
OK, you are right !
Yozo TODA
some japanese reader is giving us a comment that
Compliant Solutions (Reference Queue and Soft Reference) have
storeTempConnection
method like thiswhich re-assign
wr
before using the value from the first assignment. something strange...This code is introduced on revision 40.
I suppose the code should be like this?
David Svoboda
Agreed, I modified both code examples as you suggest. Thanks.
Yozo TODA
thanks, David!
I added missing re-assignments. (rev.134)