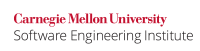
The default SecurityManager
checks whether the caller of a particular method has sufficient permissions to proceed with an action. An action is defined in Java's security architecture as a level of access and requires certain permissions before it can be performed. For Example, the actions for java.io.FilePermission
are read, write, execute, and delete [API 2013]. The "Permission Descriptions and Risks" guide [Oracle 2011d] enumerates the default permissions and the risks associated with granting these permissions to Java code.
Sometimes, stronger restrictions than those provided by the default security manager are necessary. Failure to provide custom permissions when no corresponding default permissions exist can lead to privilege escalation vulnerabilities that enable untrusted callers to execute restricted operations or actions.
This guideline addresses the problem of excess privileges. See SEC50-J. Avoid granting excess privileges for another approach to solving this problem.
Noncompliant Code Example
This noncompliant code example contains a privileged block that is used to perform two sensitive operations: loading a library and setting the default exception handler.
class LoadLibrary { private void loadLibrary() { AccessController.doPrivileged(new PrivilegedAction() { public Object run() { // Privileged code System.loadLibrary("myLib.so"); // Perform some sensitive operation like setting the default exception handler MyExceptionReporter.setExceptionReporter(reporter); return null; } }); } }
When used, the default security manager forbids the loading of the library unless the RuntimePermission
loadLibrary.myLib
is granted in the policy file. However, the security manager does not automatically guard a caller from performing the second sensitive operation of setting the default exception handler because the permission for this operation is nondefault and, consequently, unavailable. This security weakness can be exploited, for example, by programming and installing an exception handler that reveals information that a legitimate handler would filter out.
Compliant Solution
This compliant solution defines a custom permission ExceptionReporterPermission
with target exc.reporter
to prohibit illegitimate callers from setting the default exception handler. This can be achieved by subclassing BasicPermission
, which allows binary-style permissions (either allow or disallow). The compliant solution then uses a security manager to check whether the caller has the requisite permission to set the handler. The code throws a SecurityException
if the check fails. The custom permission class ExceptionReporterPermission
is also defined with the two required constructors.
class LoadLibrary { private void loadLibrary() { AccessController.doPrivileged(new PrivilegedAction() { public Object run() { // Privileged code System.loadLibrary("myLib.so"); // Perform some sensitive operation like setting the default exception handler MyExceptionReporter.setExceptionReporter(reporter); return null; } }); } } final class MyExceptionReporter extends ExceptionReporter { public void setExceptionReporter(ExceptionReporter reporter) { SecurityManager sm = System.getSecurityManager(); if(sm != null) { sm.checkPermission(new ExceptionReporterPermission("exc.reporter")); } // Proceed to set the exception reporter } // ... Other methods of MyExceptionReporter } final class ExceptionReporterPermission extends BasicPermission { public ExceptionReporterPermission(String permName) { super(permName); } // Even though the actions parameter is ignored, this constructor has to be defined public ExceptionReporterPermission(String permName, String actions) { super(permName, actions); } }
The policy file needs to grant two permissions: ExceptionReporterPermission exc.reporter
and RuntimePermission loadlibrary.myLib
. The following policy file assumes that the preceding sources reside in the c:\package
directory on a Windows-based system.
grant codeBase "file:/c:/package" { // For *nix, file:${user.home}/package/ permission ExceptionReporterPermission "exc.reporter"; permission java.lang.RuntimePermission "loadLibrary.myLib"; };
By default, permissions cannot be defined to support actions using BasicPermission
, but the actions can be freely implemented in the subclass ExceptionReporterPermission
if required. BasicPermission
is abstract
even though it contains no abstract methods; it defines all the methods that it extends from the Permission
class. The custom-defined subclass of the BasicPermission
class must define two constructors to call the most appropriate (one- or two-argument) superclass constructor (because the superclass lacks a default constructor). The two-argument constructor also accepts an action even though a basic permission does not use it. This behavior is required for constructing permission objects from the policy file. Note that the custom-defined subclass of the BasicPermission
class is declared to be final
.
Applicability
Running Java code without defining custom permissions where default permissions are inapplicable can leave an application open to privilege escalation vulnerabilities.
Bibliography
[API 2013] | Class FilePermission Class SecurityManager |
[Oaks 2001] | Chapter 5, "The Access Controller," "Permissions" |
[Oracle 2011d] | Permissions in the Java™ SE 6 Development Kit (JDK) |
[Oracle 2013c] | Permissions in Java SE 7 Development Kit (JDK) |
[Policy 2010] | "Permission Descriptions and Risks" |
5 Comments
David Svoboda
This is a decent rule, although it looks to me like a duplicate of SEC50-JG. Avoid granting excess privileges. Why don't we merge them together?
This line bothers me:
This is not the way you load up Java's AWT package. Replace "awt" with "somelib.so".
Fred Long
I think that this is rather different from SEC50-JG. SEC50-JG talks about setting an access control context using existing permissions. This guideline talks about defining custom permissions in situations where existing permissions are not adequate. I think that they should be kept separate.
I agree with your two bullet points, though.
David Svoboda
I'll fix the bullet points Monday.
It looks to me that this rule and SEC50-JG address the same problem...not giving enough restrictions to an untrusted program. They provide different solutions (using preexisting permissions vs granting new custom permissions). So I could still make an argument for merging them.
At the very least, they should cross-reference each other.
David Svoboda
bullet points addressed.
David Svoboda
I still have a nagging feeling this rule should be merged with SEC50-JG. They both have similar NCCEs, with two different compliant solutions.