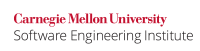
Threads that invoke Object.wait()
expect to wake up and resume execution when their condition predicate becomes true. To be compliant with THI03-J. Always invoke wait() and await() methods inside a loop, waiting threads must test their condition predicates upon receiving notifications and must resume waiting if the predicates are false.
The notify()
and notifyAll()
methods of package java.lang.Object
are used to wake up a waiting thread or threads, respectively. These methods must be invoked from a thread that holds the same object lock as the waiting thread(s); these methods throw an IllegalMonitorStateException
when invoked from any other thread. The notifyAll()
method wakes up all threads waiting on an object lock and allows threads whose condition predicate is true to resume execution. Furthermore, if all the threads whose condition predicate evaluates to true previously held a specific lock before going into the wait state, only one of them will reacquire the lock upon being notified. Presumably, the other threads will resume waiting. The notify()
method wakes up only one thread, with no guarantee regarding which specific thread is notified. The chosen thread is permitted to resume waiting if its condition predicate is unsatisfied; this often defeats the purpose of the notification.
Consequently, invoking the notify()
method is permitted only when all of the following conditions are met:
- All waiting threads have identical condition predicates.
- All threads perform the same set of operations after waking up. That is, any one thread can be selected to wake up and resume for a single invocation of
notify()
. - Only one thread is required to wake upon the notification.
These conditions are satisfied by threads that are identical and provide a stateless service or utility.
The java.util.concurrent.locks
utilities provide the Condition.signal()
and Condition.signalAll()
methods to awaken threads that are blocked on a Condition.await()
call. Condition
objects are required when using java.util.concurrent.locks.Lock
objects. Although Lock
objects allow the use of Object.wait()
, Object.notify()
, and Object.notifyAll()
methods, their use is prohibited by LCK03-J. Do not synchronize on the intrinsic locks of high-level concurrency objects. Code that synchronizes using a Lock
object uses one or more Condition
objects associated with the Lock
object rather than using its own intrinsic lock. These objects interact directly with the locking policy enforced by the Lock
object. Consequently, the await()
, signal()
, and signalAll()
methods are used in place of the wait()
, notify()
, and notifyAll()
methods.
The signal()
method must not be used unless all of these conditions are met:
- The
Condition
object is identical for each waiting thread. - All threads must perform the same set of operations after waking up, which means that any one thread can be selected to wake up and resume for a single invocation of
signal()
. - Only one thread is required to wake upon receiving the signal.
or all of these conditions are met:
- Each thread uses a unique
Condition
object. - Each
Condition
object is associated with the sameLock
object.
When used securely, the signal()
method has better performance than signalAll()
.
When notify()
or signal()
is used to waken a waiting thread, and the thread is not prepared to resume execution, it often resumes waiting. Consequently, no thread wakens, which may cause the system to hang.
Noncompliant Code Example (notify()
)
This noncompliant code example shows a complex, multistep process being undertaken by several threads. Each thread executes the step identified by the time
field. Each thread waits for the time
field to indicate that it is time to perform the corresponding thread's step. After performing the step, each thread first increments time
and then notifies the thread that is responsible for the next step.
public final class ProcessStep implements Runnable { private static final Object lock = new Object(); private static int time = 0; private final int step; // Do Perform operations when field time // reaches this value public ProcessStep(int step) { this.step = step; } @Override public void run() { try { synchronized (lock) { while (time != step) { lock.wait(); } // Perform operations time++; lock.notify(); } } catch (InterruptedException ie) { Thread.currentThread().interrupt(); // Reset interrupted status } } public static void main(String[] args) { for (int i = 4; i >= 0; i--) { new Thread(new ProcessStep(i)).start(); } } }
This noncompliant code example violates the liveness property. Each thread has a different condition predicate because each requires step
to have a different value before proceeding. The Object.notify()
method wakes only one thread at a time. Unless it happens to wake the thread that is required to perform the next step, the program will deadlock.
Compliant Solution (notifyAll()
)
In this compliant solution, each thread completes its step and then calls notifyAll()
to notify the waiting threads. The thread that is ready can then perform its task while all the threads whose condition predicates are false (loop condition expression is true) promptly resume waiting.
Only the run()
method from the noncompliant code example is modified, as follows:
public final class ProcessStep implements Runnable { private static final Object lock = new Object(); private static int time = 0; private final int step; // Perform operations when field time // reaches this value public ProcessStep(int step) { this.step = step; } @Override public void run() { try { synchronized (lock) { while (time != step) { lock.wait(); } // Perform operations time++; lock.notifyAll(); // Use notifyAll() instead of notify() } } catch (InterruptedException ie) { Thread.currentThread().interrupt(); // Reset interrupted status } } }
Noncompliant Code Example (Condition
Interface)
This noncompliant code example is similar to the noncompliant code example for notify()
but uses the Condition
interface for waiting and notification:
public class ProcessStep implements Runnable { private static final Lock lock = new ReentrantLock(); private static final Condition condition = lock.newCondition(); private static int time = 0; private final int step; // Perform operations when field time // reaches this value public ProcessStep(int step) { this.step = step; } @Override public void run() { lock.lock(); try { while (time != step) { condition.await(); } // Perform operations time++; condition.signal(); } catch (InterruptedException ie) { Thread.currentThread().interrupt(); // Reset interrupted status } finally { lock.unlock(); } } public static void main(String[] args) { for (int i = 4; i >= 0; i--) { new Thread(new ProcessStep(i)).start(); } } }
As with Object.notify()
, the signal()
method may awaken an arbitrary thread.
Compliant Solution (signalAll()
)
This compliant solution uses the signalAll()
method to notify all waiting threads. Before await()
returns, the current thread reacquires the lock associated with this condition. When the thread returns, it is guaranteed to hold this lock [API 2014]. The thread that is ready can perform its task while all the threads whose condition predicates are false resume waiting.
Only the run()
method from the noncompliant code example is modified, as follows:
public class ProcessStep implements Runnable { private static final Lock lock = new ReentrantLock(); private static final Condition condition = lock.newCondition(); private static int time = 0; private final int step; // Perform operations when field time // reaches this value public ProcessStep(int step) { this.step = step; } @Override public void run() { lock.lock(); try { while (time != step) { condition.await(); } // Perform operations time++; condition.signalAll(); } catch (InterruptedException ie) { Thread.currentThread().interrupt(); // Reset interrupted status } finally { lock.unlock(); } } }
Compliant Solution (Unique Condition per Thread)
This compliant solution assigns each thread its own condition. All the Condition
objects are accessible to all the threads:
// Declare class as final because its constructor throws an exception public final class ProcessStep implements Runnable { private static final Lock lock = new ReentrantLock(); private static int time = 0; private final int step; // Perform operations when field time // reaches this value private static final int MAX_STEPS = 5; private static final Condition[] conditions = new Condition[MAX_STEPS]; public ProcessStep(int step) { if (step <= MAX_STEPS) { this.step = step; conditions[step] = lock.newCondition(); } else { throw new IllegalArgumentException("Too many threads"); } } @Override public void run() { lock.lock(); try { while (time != step) { conditions[step].await(); } // Perform operations time++; if (step + 1 < conditions.length) { conditions[step + 1].signal(); } } catch (InterruptedException ie) { Thread.currentThread().interrupt(); // Reset interrupted status } finally { lock.unlock(); } } public static void main(String[] args) { for (int i = MAX_STEPS - 1; i >= 0; i--) { ProcessStep ps = new ProcessStep(i); new Thread(ps).start(); } } }
Even though the signal()
method is used, only the thread whose condition predicate corresponds to the unique Condition
variable will awaken.
This compliant solution is safe only when untrusted code cannot create a thread with an instance of this class.
Risk Assessment
Notifying a single thread rather than all waiting threads can violate the liveness property of the system.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
THI02-J | Low | Unlikely | Medium | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Parasoft Jtest | 2024.1 | CERT.THI02.ANF | Do not use 'notify()'; use 'notifyAll()' instead so that all waiting threads will be notified |
SonarQube | 9.9 | S2446 | "notifyAll" should be used |
17 Comments
Dhruv Mohindra
TODO: Investigate, performance loss vs. security trade-off.
Fred Long
See Bloch 08, Item 69: Prefer concurrency utilities to wait and notify for a possible alternative compliant solution, or possibly another rule/recommendation.
Dhruv Mohindra
I think I am going to rename this to something like "Notify all waiting threads instead of a single thread". Then I can also include
signal()
andsignalAll()
fromjava.util.concurrent.locks
InterfaceCondition
.David Svoboda
Dhruv Mohindra
I think we should get rid of the value "6" in the statement - new Condition[6]; of the last CS.
David Svoboda
Feel free to rewrite the code as you see fit. 6 is just the minimum value needed for the code to work.
Robert Seacord
I removed the sentence from the NCE for the Condition Interface:
The
condition
field allows each thread to wait on a different condition predicate.Because I didn't see in this example that there was more than one condition predicate.
This is obviously true in the "Unique Condition Per Thread" but this is clearly stated there.
I'm also removing the status field because I think this needs further review.
Dhruv Mohindra
"An IllegalMonitorStateException is thrown if the current thread is not the owner of this object's monitor." . I think the problem is more like - the wrong lock object will receive the notification.
Dhruv Mohindra
Doesn't appear that the use-case described in the first NCE can be implemented cleanly with the j.u.c utilities (except for using
Condition
which is already shown). Suggestions anyone? The idea is to recommend j.u.c over wait/notifyAll as a compliant solution. Otherwise, I am leaving this as is.Jonathan Paulson
Just a note: [Rogue 2000] Rule 98: Consider using
notify()
instead ofnotifyAll()
directly contradicts this guideline. Their arguments are based on performance.David Svoboda
notify() is clearly faster than notifyAll(), and I believe this rule does address the (few) cases when notify() is safe to use.
Simon Roberts
Perhaps I'm misreading this (in which case, I'd beg an edit to help clarify). However, I'm deeply concerned by one aspect of what I think I'm reading.
This requirement seems to be that signal() should only be used in place of signalAll() in one of two scenarios. If I understand the text correctly then I can paraphrase these as: first, _all_ threads are waiting for the same reason, second, every thread has it's own condition variable.
This appears to omit what I believe is the proper use of the condition mechanism. As follows (hopefully succinct, but not failing to identify the key points--clearly contradictory goals!).
--------
Every "reason for waiting" has its own condition variable. Every thread that await()s a particular condition variable must be equally capable of immediately and properly resolving the reason that condition was signaled.
--------
Notice that rather than giving every _thread_ its own condition (which seems to create rather onerous dependencies), the focus here is on "what the thread(s) do(es)". Any thread that can resolve the condition can validly await that condition, any thread that might fail to resolve the condition must not await on it.
My major concern with the use of the xxxAll() methods is that, as has been noted, from an architectural / scalability perspective, using either notifyAll, or signalAll forces _every_ waiting thread to go through the cycle of waiting-for-notification->waiting-for-lock->running and in most cases all but one of them will go right back to waiting. This is an enormous overhead in highly concurrent systems and can cause a huge loss of throughput due to the slew of non-productive wakeup-investigate-gobacktosleep operations.
Now, it's possible that there's a specifically _security_ reason (as opposed to a more general "concurrency correctness" reason) for the suggestion you gave. However, if that's the case, the reason for it is not clear from the text.
David Svoboda
The reason for this rule is that if you signal a single thread waiting on a condition, and that thread is not able to resolve the condition, then your design is flawed. That is, your thread must now somehow signal the right thread, which is not obvious if there are multiple threads waiting. This is a security problem simply because oftentimes when the wrong thread is signelled, it goes right back to waiting, resulting in deadlock (or permanent waiting). You might consider this a 'concurrency correctness' reason.
This should be apparent from the noncompliant code examples, and is stated in the Risk Assessment section. But I'll agree that the intro text should make this clear but doesn't. So I've added the following paragraph to the intro:
Gianni Belli
A "static Lock" is very dangerous. It can severely destry a banking application for example.
Can you please check that declaration. This was a very dangerous piece of code. I have take a picture and saved this code. This securecoding at cert seems very dangerous
David Svoboda
I think you are referring to the static lock in all of the code examples, right?
It is correct that the lock is static, as there should be only one such lock for all threads, and instances of the ProcessStep object. You might also consider the lock as protecting the increment of the time variable.
Gianni Belli
Hi! Why this example at oracle.com https://docs.oracle.com/javase/tutorial/essential/concurrency/newlocks.html
does not use a static Lock?
Also the first compliant example here LCK00-J. Use private final lock objects to synchronize classes that may interact with untrusted code
does not use a static Lock. Why?
David Svoboda
Because they are each protecting a field that is not static. See LCK06-J. Do not use an instance lock to protect shared static data for more information.