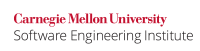
Threads and tasks that block on operations involving network or file I/O must provide callers with an explicit termination mechanism to prevent denial-of-service (DoS) vulnerabilities.
Noncompliant Code Example (Blocking I/O, Volatile Flag)
This noncompliant code example uses a volatile done
flag to indicate it whether is safe to shut down the thread, as suggested in THI05-J. Do not use Thread.stop() to terminate threads. However, when the thread is blocked on network I/O as a consequence of invoking the readLine()
method, it cannot respond to the newly set flag until the network I/O is complete. Consequently, thread termination may be indefinitely delayed.
// Thread-safe class public final class SocketReader implements Runnable { private final Socket socket; private final BufferedReader in; private volatile boolean done = false; private final Object lock = new Object(); public SocketReader(String host, int port) throws IOException { this.socket = new Socket(host, port); this.in = new BufferedReader( new InputStreamReader(this.socket.getInputStream()) ); } // Only one thread can use the socket at a particular time @Override public void run() { try { synchronized (lock) { readData(); } } catch (IOException ie) { // Forward to handler } } public void readData() throws IOException { String string; while (!done && (string = in.readLine()) != null) { // Blocks until end of stream (null) } } public void shutdown() { done = true; } public static void main(String[] args) throws IOException, InterruptedException { SocketReader reader = new SocketReader("somehost", 25); Thread thread = new Thread(reader); thread.start(); Thread.sleep(1000); reader.shutdown(); // Shut down the thread } }
Noncompliant Code Example (Blocking I/O, Interruptible)
This noncompliant code example is similar to the preceding example but uses thread interruption to shut down the thread. Network I/O on a java.net.Socket
is unresponsive to thread interruption.
// Thread-safe class public final class SocketReader implements Runnable { // Other methods... public void readData() throws IOException { String string; while (!Thread.interrupted() && (string = in.readLine()) != null) { // Blocks until end of stream (null) } } public static void main(String[] args) throws IOException, InterruptedException { SocketReader reader = new SocketReader("somehost", 25); Thread thread = new Thread(reader); thread.start(); Thread.sleep(1000); thread.interrupt(); // Interrupt the thread } }
Compliant Solution (Close Socket Connection)
This compliant solution terminates the blocking network I/O by closing the socket in the shutdown()
method. The readLine()
method throws a SocketException
when the socket is closed, consequently allowing the thread to proceed. Note that it is impossible to keep the connection alive while simultaneously halting the thread both cleanly and immediately.
public final class SocketReader implements Runnable { // Other methods... public void readData() throws IOException { String string; try { while ((string = in.readLine()) != null) { // Blocks until end of stream (null) } } finally { shutdown(); } } public void shutdown() throws IOException { socket.close(); } public static void main(String[] args) throws IOException, InterruptedException { SocketReader reader = new SocketReader("somehost", 25); Thread thread = new Thread(reader); thread.start(); Thread.sleep(1000); reader.shutdown(); } }
After the shutdown()
method is called from main()
, the finally
block in readData()
executes and calls shutdown()
again, closing the socket for a second time. However, when the socket has already been closed, this second call does nothing.
When performing asynchronous I/O, a java.nio.channels.Selector
can be unblocked by invoking either its close()
or its wakeup()
method.
When additional operations must be performed after emerging from the blocked state, use a boolean
flag to indicate pending termination. When supplementing the code with such a flag, the shutdown()
method should also set the flag to false so that the thread can cleanly exit from the while
loop.
Compliant Solution (Interruptible Channel)
This compliant solution uses an interruptible channel, java.nio.channels.SocketChannel
, instead of a Socket
connection. If the thread performing the network I/O is interrupted using the Thread.interrupt()
method while it is reading the data, the thread receives a ClosedByInterruptException
, and the channel is closed immediately. The thread's interrupted status is also set.
public final class SocketReader implements Runnable { private final SocketChannel sc; private final Object lock = new Object(); public SocketReader(String host, int port) throws IOException { sc = SocketChannel.open(new InetSocketAddress(host, port)); } @Override public void run() { ByteBuffer buf = ByteBuffer.allocate(1024); try { synchronized (lock) { while (!Thread.interrupted()) { sc.read(buf); // ... } } } catch (IOException ie) { // Forward to handler } } public static void main(String[] args) throws IOException, InterruptedException { SocketReader reader = new SocketReader("somehost", 25); Thread thread = new Thread(reader); thread.start(); Thread.sleep(1000); thread.interrupt(); } }
This technique interrupts the current thread. However, it stops the thread only because the code polls the thread's interrupted status with the Thread.interrupted()
method and terminates the thread when it is interrupted. Using a SocketChannel
ensures that the condition in the while
loop is tested as soon as an interruption is received, even though the read is normally a blocking operation. Similarly, invoking the interrupt()
method of a thread blocked on a java.nio.channels.Selector
also causes that thread to awaken.
Noncompliant Code Example (Database Connection)
This noncompliant code example shows a thread-safe DBConnector
class that creates one JDBC connection per thread. Each connection belongs to one thread and is not shared by other threads. This is a common use case because JDBC connections are intended to be single-threaded.
public final class DBConnector implements Runnable { private final String query; DBConnector(String query) { this.query = query; } @Override public void run() { Connection connection; try { // Username and password are hard coded for brevity connection = DriverManager.getConnection( "jdbc:driver:name", "username", "password" ); Statement stmt = connection.createStatement(); ResultSet rs = stmt.executeQuery(query); // ... } catch (SQLException e) { // Forward to handler } // ... } public static void main(String[] args) throws InterruptedException { DBConnector connector = new DBConnector("suitable query"); Thread thread = new Thread(connector); thread.start(); Thread.sleep(5000); thread.interrupt(); } }
Database connections, like sockets, lack inherent interruptibility. Consequently, this design fails to support the client's attempts to cancel a task by closing the resource when the corresponding thread is blocked on a long-running query, such as a join.
Compliant Solution (Statement.cancel()
)
This compliant solution uses a ThreadLocal
wrapper around the connection so that a thread calling the initialValue()
method obtains a unique connection instance. This approach allows provision of a cancelStatement()
so that other threads or clients can interrupt a long-running query when required. The cancelStatement()
method invokes the Statement.cancel()
method.
public final class DBConnector implements Runnable { private final String query; private volatile Statement stmt; DBConnector(String query) { this.query = query; } private static final ThreadLocal<Connection> connectionHolder = new ThreadLocal<Connection>() { Connection connection = null; @Override public Connection initialValue() { try { // ... connection = DriverManager.getConnection( "jdbc:driver:name", "username", "password" ); } catch (SQLException e) { // Forward to handler } return connection; } }; public Connection getConnection() { return connectionHolder.get(); } public boolean cancelStatement() { // Allows client to cancel statement Statement tmpStmt = stmt; if (tmpStmt != null) { try { tmpStmt.cancel(); return true; } catch (SQLException e) { // Forward to handler } } return false; } @Override public void run() { try { if (getConnection() != null) { stmt = getConnection().createStatement(); } if (stmt == null || (stmt.getConnection() != getConnection())) { throw new IllegalStateException(); } ResultSet rs = stmt.executeQuery(query); // ... } catch (SQLException e) { // Forward to handler } // ... } public static void main(String[] args) throws InterruptedException { DBConnector connector = new DBConnector("suitable query"); Thread thread = new Thread(connector); thread.start(); Thread.sleep(5000); connector.cancelStatement(); } }
The Statement.cancel()
method cancels the query, provided the database management system (DBMS) and driver both support cancellation. It is impossible to cancel the query if either the DBMS or the driver fail to support cancellation.
According to the Java API, Interface Statement
documentation [API 2014]
By default, only one
ResultSet
object perStatement
object can be open at the same time. As a result, if the reading of oneResultSet
object is interleaved with the reading of another, each must have been generated by differentStatement
objects.
This compliant solution ensures that only one ResultSet
is associated with the Statement
belonging to an instance, and, consequently, only one thread can access the query results.
Risk Assessment
Failure to provide facilities for thread termination can cause nonresponsiveness and DoS.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
THI04-J | Low | Probable | Medium | P4 | L3 |
Bibliography
[API 2014] | |
Section 24.3, "Stopping a Thread" | |
Chapter 7, "Cancellation and Shutdown" | |
Section 2.4, "Two Approaches to Stopping a Thread" | |
Java Thread Primitive Deprecation | |
[JPL 2006] | Section 14.12.1, "Don't Stop" |
3 Comments
Dhruv Mohindra
Could also have poison pills as a CS though it might be less favorable than existing CSs.
Robert Seacord
Comment from Dhruv about "Database connections, like sockets, lack inherent interruptibility."
Typically code uses a caching mechanism with proper timeouts to get around such delays, sometimes also called as âimpatient requestorâ pattern. It might be too late to discuss this exception unless someone wants to bungee jump.
James Ahlborn
When I initially looked at the last CS (Statement.cancel), I noticed that the DBConnector was not well designed as it did heavy lifting in the constructor (generally a bad idea). Then I realized that on top of that, it was a broken example because the ThreadLocal Connection was established in the main thread, not the child thread as intended. Also, the asynchronous cancelStatement() method is vulnerable to TOCTOU if the use of stmt ever changes in the class. I'll clean up the example.