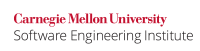
Security checks based on untrusted sources can be bypassed. Any untrusted object or argument must be defensively copied before a security check is performed. The copy operation must be a deep copy; the implementation of the clone()
method may produce a shallow copy, which can still be compromised. In addition, the implementation of the clone()
method can be provided by the attacker (see OBJ06-J. Defensively copy mutable inputs and mutable internal components for more information).
Noncompliant Code Example
This noncompliant code example describes a security vulnerability from the Java 1.5 java.io
package. In this release, java.io.File
is nonfinal, allowing an attacker to supply an untrusted argument constructed by extending the legitimate File
class. In this manner, the getPath()
method can be overridden so that the security check passes the first time it is called but the value changes the second time to refer to a sensitive file such as /etc/passwd
. This is an example of a time-of-check, time-of-use (TOCTOU) vulnerability.
public RandomAccessFile openFile(final java.io.File f) { askUserPermission(f.getPath()); // ... return (RandomAccessFile)AccessController.doPrivileged(new PrivilegedAction <Object>() { public Object run() { return new RandomAccessFile(f, f.getPath()); } }); }
The attacker could extend java.io.File
as follows:
public class BadFile extends java.io.File { private int count; public String getPath() { return (++count == 1) ? "/tmp/foo" : "/etc/passwd"; } }
Compliant Solution (Final)
This vulnerability can be mitigated by declaring java.io.File
final.
Compliant Solution (Copy)
This compliant solution ensures that the java.io.File
object can be trusted despite not being final. The solution creates a new File
object using the standard constructor. This technique ensures that any methods invoked on the File
object are the standard library methods and not overriding methods that have been provided by the attacker.
public RandomAccessFile openFile(java.io.File f) { final java.io.File copy = new java.io.File(f.getPath()); askUserPermission(copy.getPath()); // ... return (RandomAccessFile)AccessController.doPrivileged(new PrivilegedAction <Object>() { public Object run() { return new RandomAccessFile(copy, copy.getPath()); } }); }
Note that using the clone()
method instead of the openFile()
method would copy the attacker's class, which is not desirable (see OBJ06-J. Defensively copy mutable inputs and mutable internal components).
Risk Assessment
Basing security checks on untrusted sources can result in the check being bypassed.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
SEC02-J | High | Probable | Medium | P12 | L1 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Coverity | 7.5 | UNSAFE_REFLECTION | Implemented |
Parasoft Jtest | 2023.1 | BD.SECURITY.TDRFL | Protect against Reflection injection |
Related Guidelines
Authentication Logic Error [XZO] | |
CWE-302, Authentication Bypass by Assumed-Immutable Data |
Android Implementation Details
The code examples using the java.security
package are not applicable to Android, but the principle of the rule is applicable to Android apps.