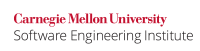
While it used to be common practice to use integers and pointers interchangeably in C (although it was not considered good style), the C99 standard has mandated that pointer to integer and integer to pointer conversions are implementation defined. By definition of the standard, the only value which can be considered interchangeable between pointers and integers is the constant 0. This means that less the exception of 0, mixing integers and pointers may have undesired consequences depending on the implementation which is used.
Universal Integer/Pointer Storage
Non-Compliant Code
In this non-compliant code, the pointer ptr
is used in an arithmetic operation that is eventually casted as an integer, as stated above, the actual result for both this assignment and following assignment to ptr2
are implementation defined.
unsigned int myint = 0; unsigned int *ptr = &myint; ... unsigned int number = ptr + 1; unsigned int *ptr2 = ptr;
Compliant Solution
A union can be used to give raw memory access to both an integer and a pointer and will take up as much size as its largest element which will mitigate a loss of data.
union intpoint { unsigned int *pointer; unsigned int number; } intpoint; ... intpoint mydata = 0xcfcfcfcf; ... unsigned int num = mydata.number + 1; unsigned int *ptr = mydata.pointer;
Accessing Memory-Mapped Addresses*
Note: This is a bad idea. The following is a description of how to more properly execute a bad idea.
Oftentimes in low level kernel or graphics code you will need to access memory at a specific location. Thus, you are going to have to make a literal integer to pointer to conversion.
Non-Compliant Code Example
In this non-compliant code, a pointer is set directly to an integer constant, where it is unknown whether the result will be as intended.
unsigned int *ptr = 0xcfcfcfcf;
Because integers are no longer pointers this could have drastic consequences.
Compliant Solution
Adding in an explicit cast may help the compiler format and store the value as expected into the pointer.
unsigned int *ptr = (unsigned int *) 0xcfcfcfcf;
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT14-A |
2 |
2(unlikely) |
3(medium) |
P2 |
L3 |
References
[[ISO/IEC 9899-1999:TC2]] Section 6.3.2.3, "Pointers"