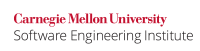
Do not access or modify the result of a function call after a subsequent sequence point. According to C99 Section 6.5.2.2, "Function calls":
If an attempt is made to modify the result of a function call or to access it after the next sequence point, the behavior is undefined.
Non-Compliant Code Example
In C, the lifetime of a return value ends at the next sequence point.
#include <stdio.h> struct X { char a[6]; }; struct X addressee() { struct X result = { "world" }; return result; } int main(void) { printf("Hello, %s!\n", addressee().a); return 0; }
Because there is a sequence point after the call to addressee()
and before the call to printf()
, this program has
undefined behavior.
Implementation Details
This code compiles cleanly and runs without error under Microsoft Visual C++ Version 8.0. On gcc version 4.1, the program compiles with a warning when the -Wall
switch is used and execution on Linux results in a segmentation fault.
Compliant Solution
This compliant solution does not have undefined behavior because the reference to the returned value is evaluated before the subsequent sequence point.
#include <stdio.h> struct X { char a[6]; }; struct X addressee() { struct X result = { "world" }; return result; } int main(void) { printf("Hello, %c!\n", addressee().a[rand() % 5]); return 0; }
Risk Assessment
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP34-C |
1 (low) |
1 (low) |
3 (medium) |
P3 |
L3 |
Examples of vulnerabilities resulting from the violation of this rule can be found on the CERT website.
References
[[ISO/IEC 9899-1999]] Section 6.5.2.2, "Function calls"