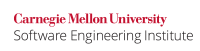
Integer conversions, both implicit and explicit (using a cast), must be guaranteed not to result in lost or misinterpreted data. This is particularly true for integer values that originate from untrusted sources and are used in any of the following ways:
- as an array index
- in any pointer arithmetic
- as a length or size of an object
- as the bound of an array (for example, a loop counter)
- as an argument to a memory allocation function
- in security-critical code
The only integer type conversions that are guaranteed to be safe for all data values and all possible conforming implementations are conversions of an integral value to a wider type of the same signedness. Section 6.3.1.3 of the C Standard [ISO/IEC 9899:2011] says,
When a value with integer type is converted to another integer type other than
_Bool
, if the value can be represented by the new type, it is unchanged.Otherwise, if the new type is unsigned, the value is converted by repeatedly adding or subtracting one more than the maximum value that can be represented in the new type until the value is in the range of the new type.
Otherwise, the new type is signed and the value cannot be represented in it; either the result is implementation-defined or an implementation-defined signal is raised.
Typically, converting an integer to a smaller type results in truncation of the high-order bits.
Noncompliant Code Example (Unsigned to Signed)
Type range errors, including loss of data (truncation) and loss of sign (sign errors), can occur when converting from an unsigned type to a signed type. The following noncompliant code example results in a truncation error on most implementations.
unsigned long int ul = ULONG_MAX; signed char sc; sc = (signed char)ul; /* cast eliminates warning */
Compliant Solution (Unsigned to Signed)
Validate ranges when converting from an unsigned type to a signed type. The following code, for example, can be used when converting from unsigned long int
to a signed char
.
unsigned long int ul = ULONG_MAX; signed char sc; if (ul <= SCHAR_MAX) { sc = (signed char)ul; /* use cast to eliminate warning */ } else { /* handle error condition */ }
Noncompliant Code Example (Signed to Unsigned)
Type range errors, including loss of data (truncation) and loss of sign (sign errors), can occur when converting from a signed type to an unsigned type. The following code results in a loss of sign.
signed int si = INT_MIN; unsigned int ui = (unsigned int)si; /* cast eliminates warning */
Compliant Solution (Signed to Unsigned)
Validate ranges when converting from a signed type to an unsigned type. The following code, for example, can be used when converting from signed int
to unsigned int
.
signed int si = INT_MIN; unsigned int ui; if (si < 0) { /* handle error condition */ } else { ui = (unsigned int)si; /* cast eliminates warning */ }
NOTE: While unsigned types can usually represent all positive values of the corresponding signed type, this relationship is not guaranteed by the C standard.
Noncompliant Code Example (Signed, Loss of Precision)
A loss of data (truncation) can occur when converting from a signed type to a signed type with less precision. The following code can result in truncation.
signed long int sl = LONG_MAX; signed char sc = (signed char)sl; /* cast eliminates warning */
Compliant Solution (Signed, Loss of Precision)
Validate ranges when converting from a signed type to a signed type with less precision. The following code can be used, for example, to convert from a signed long int
to a signed char
.
signed long int sl = LONG_MAX; signed char sc; if ( (sl < SCHAR_MIN) || (sl > SCHAR_MAX) ) { /* handle error condition */ } else { sc = (signed char)sl; /* use cast to eliminate warning */ }
Conversions from signed types with greater precision to signed types with lesser precision require both the upper and lower bounds to be checked.
Noncompliant Code Example (Unsigned, Loss of Precision)
A loss of data (truncation) can occur when converting from an unsigned type to an unsigned type with less precision. The following code results in a truncation error on most implementations.
unsigned long int ul = ULONG_MAX; unsigned char uc = (unsigned char)ul; /* cast eliminates warning */
Compliant Solution (Unsigned, Loss of Precision)
Validate ranges when converting from an unsigned type to an unsigned type with lesser precision. The following code can be used, for example, to convert from an unsigned long int
to an unsigned char
.
unsigned long int ul = ULONG_MAX; unsigned char uc; if (ul > UCHAR_MAX) ) { /* handle error condition */ } else { uc = (unsigned char)ul; /* use cast to eliminate warning */ }
Conversions from unsigned types with greater precision to unsigned types with lesser precision require only the upper bounds to be checked.
Exceptions
INT31-EX1: The C standard defines minimum ranges for standard integer types. For example, the minimum range for an object of type unsigned short int
is 0 to 65,535, while the minimum range for int
is −32,767 to +32,767. This means that it is not always possible to represent all possible values of an unsigned short int
as an int
. However, on the IA-32 architecture, for example, the actual integer range is from −2,147,483,648 to +2,147,483,647, meaning that it is quite possible to represent all the values of an unsigned short int
as an int
for this architecture. As a result, it is not necessary to provide a test for this conversion on IA-32. It is not possible to make assumptions about conversions without knowing the precision of the underlying types. If these tests are not provided, assumptions concerning precision must be clearly documented, as the resulting code cannot be safely ported to a system where these assumptions are invalid. A good way to document these assumptions is to use static assertions. (See DCL03-C. Use a static assertion to test the value of a constant expression.)
Risk Assessment
Integer truncation errors can lead to buffer overflows and the execution of arbitrary code by an attacker.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
INT31-C | high | probable | high | P6 | L2 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Compass/ROSE | Can detect violations of this rule. However, false warnings may be raised if | ||
2017.07 | NEGATIVE_RETURNS | Can find array accesses, loop bounds, and other expressions that may contain dangerous implied integer conversions that would result in unexpected behavior. | |
2017.07 | REVERSE_NEGATIVE | Can find instances where a negativity check occurs after the negative value has been used for something else. | |
2017.07 | MISRA_CAST | Can find the instances where an integer expression is implicitly converted to a narrower integer type, or implicitly converting the signedness of an integer value or implicitly converting the type of a complex expression. | |
Fortify SCA | 5.0 | Can detect violations of this rule with CERT C Rule Pack. | |
2024.1 | PRECISION.LOSS | ||
9.7.1 | 93 S | Fully implemented. | |
PRQA QA-C | Unable to render {include} The included page could not be found. | 2850 (C) | Partially implemented |
* Coverity Prevent cannot discover all violations of this rule, so further verification is necessary.
Related Vulnerabilities
CVE-2009-1376 results from a violation of this rule. In version 2.5.5 of Pidgin, an unsigned integer (offset
) is set to the value of a 64-bit unsigned integer, which can lead to truncation [xorl 2009]. An attacker can execute arbitrary code by carefully choosing this value and causing a buffer overflow.
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
CERT C++ Secure Coding Standard | INT31-CPP. Ensure that integer conversions do not result in lost or misinterpreted data |
CERT Oracle Secure Coding Standard for Java | NUM12-J. Ensure conversions of numeric types to narrower types do not result in lost or misinterpreted data |
ISO/IEC TR 24772 | Numeric conversion errors [FLC] |
MISRA-C | Rule 10.1 |
MITRE CWE | CWE-192, Integer coercion error CWE-197, Numeric truncation error CWE-681, Incorrect conversion between numeric types |
Bibliography
[Dowd 2006] | Chapter 6, "C Language Issues" ("Type Conversions," pp. 223–270) |
[ISO/IEC 9899:2011] | Section 6.3.1.3, "Signed and Unsigned Integers" |
[Seacord 2005a] | Chapter 5, "Integers" |
[Viega 2005] | Section 5.2.9, "Truncation Error" Section 5.2.10, "Sign Extension Error" Section 5.2.11, "Signed to Unsigned Conversion Error" Section 5.2.12, "Unsigned to Signed Conversion Error" |
[Warren 2002] | Chapter 2, "Basics" |
[xorl 2009] | "CVE-2009-1376: Pidgin MSN SLP Integer Truncation" |