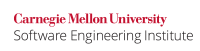
Integer arithmetic used to calculate a value for assignment to a floating point variable may lead to loss of information. This can be avoided by converting one of the integers in the expression to a floating type.
Non-Compliant Code Example
In this non-compliant code, the floating point variables d
, e
and f
are not initialized correctly because the operations take place before the values are converted to floating point values and hence the results are truncated to the nearest decimal point or may overflow. Consequently, the division and multiplication operations take place on integers then get converted to floating point.
short a = 533; int b = 6789; long c = 466438237; float d = a / 7; /* d is 76.0 */ double e = b / 30; /* e is 226.0 */ double f = c * 789; /* f may be negative due to overflow */
Compliant Solution 1
In this compliant solution, the decimal error in initialization is eliminated by ensuring that at least one of the operands to the division operation is floating point.
short a = 533; int b = 6789; long c = 466438237; float d = a / 7.0f; /* d is 76.14286 */ double e = b / 30.; /* e is 226.3 */ double f = (double)c * 789; /* f is 360*/
Compliant Solution 2
In this compliant code, the decimal error in initialization is eliminated by first storing the integer in the floating point variable and then performing the arithmetic operation. This ensures that at least one of the operands is a floating point number and consequently the operation is performed on floating point numbers.
short a = 533; int b = 6789; long c = 466438237; float d = a; double e = b; double f = c; d /= 7; /* d is 76.14286 */ e /= 30; /* e is 226.3 */ f *= 789; /* f is 591176.47275 */
Risk Assessment
It may be desirable for the operation to take place as integers before the conversion (obviating the need for a trunc()
call, for example). In such cases, it should be clearly documented to avoid future maintainers misunderstanding the intent of the code.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FLP33-C |
1 (low) |
2 (probable) |
3 (low) |
P6 |
L2 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Hatton 95]] Section 2.7.3, "Floating-point misbehavior"
[[ISO/IEC 9899-1999]] Section 5.2.4.2.2, "Characteristics of floating types <float.h>"
05. Floating Point (FLP) FLP34-C. Ensure that demoted floating point values are within range