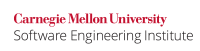
Different alignments are possible for different types of objects. If the type checking system is overridden by an explicit cast, or the pointer is cast into and out of void *
, the alignment of an object may be changed. As a result, if a pointer to one object is converted to a pointer to a different object, the objects must have the same alignment.
Non-Compliant Code Example
C99 allows a pointer may be cast into and out of void *
. As a result, it is possible to silently convert from one pointer type to another without the compiler diagnosing the problem by storing or casting a pointer to void *
and then storing or casting it to the final type. In this non-compliant code example, the type checking system is circumvented due to the caveats of void
pointers.
char *loop_ptr; int *int_ptr; int *loop_function(void *v_pointer){ return v_pointer; } int_ptr = loop_function(loop_ptr);
This example compiles without warning. However, v_pointer
may be aligned on a one byte boundary. Once it is cast to an int
, some architectures will require that the object is aligned on a four byte boundary. If int_ptr
is later dereferenced, the program may termination abnormally.
Compliant Solution
Because the input parameter directly influences the return value, and loopFunction()
returns an int *
, the formal parameter v_pointer
is redeclared to only accept int *
.
int *loop_ptr; int *int_ptr; int *loopFunction(int *v_pointer) { return v_pointer; } int_ptr = loopFunction(loop_ptr);
Implementation Details
The following list shows common alignments for Microsoft, Borland, and GNU compilers for the IA-32 architecture.
Type |
Alignment |
---|---|
|
1 byte aligned |
|
2 byte aligned |
|
4 byte aligned |
|
4 byte aligned |
|
8 byte on Windows, 4 byte on Linux |
Risk Assessment
Accessing a pointer or an object that is no longer on the correct access boundary can cause a program to crash, give wrong information, or may cause slow pointer accesses (if the architecture does not care about alignment).
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP36-C |
1 (low) |
2 (probable) |
2 (medium) |
P4 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Bryant 03]]
[[ISO/IEC 9899-1999]] Section 6.2.5, "Types"
EXP35-C. Do not access or modify the result of a function call after a subsequent sequence point 03. Expressions (EXP) EXP37-C. Call functions with the arguments intended by the API