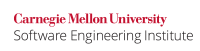
Formatted input functions such as scanf()
, fscanf()
, vscanf()
, and vfscanf()
can be used to read string data from stdin
or (in the cases of fscanf()
and vfscanf()
) other input stream. These functions work fine for valid integer values but lack robust error handling for invalid values.
Instead of these functions, try inputing the value as a string and then converting it to an integer valoue using strtol()
or a related function INT00-A.
Non-Compliant Example
This non-compliant example uses the scanf()
function to read a string from stdin
and covert it to an integer value. The scanf()
and fscanf()
functions have undefined behavior if the value of the result of this operation cannot be represented as an integer.
int si; scanf("%d", &si);
Compliant Solution
The following compliant example uses the fgets()
to input a string and strtol()
to convert the string to an integer value. Error checking is provided to make sure that the value is a valid integer in the range of int
.
char buff [25]; char *end_ptr; long sl; int si; fgets(buff, sizeof buff, stdin); errno = 0; sl = strtol(buff, &end_ptr, 0); if (ERANGE == errno) { puts("number out of range\n"); } else if (sl > INT_MAX) { printf("%ld too large!\n", sl); } else if (sl < INT_MIN) { printf("%ld too small!\n", sl); } else if (end_ptr == buff) { puts("not valid numeric input\n"); } else { si = (int)sl; }
If you are attempting to convert a string to a smaller interger type (int
, short
, or signed char
), then you only need test the result against the limits for that type. The tests do nothing if the smaller type happens to have the same size and representation on a particular compiler.
References
- Jack Klein. Bullet Proof Integer Input Using strtol(). http://home.att.net/~jackklein/c/code/strtol.html
- ISO/IEC 9899-1999 Section 7.20.1.4 The strtol, strtoll, strtoul, and strtoull functions; Section 7.19.6 Formatted input/output functions