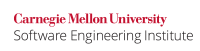
An object that has volatile-qualified type may be modified in ways unknown to the implementation or have other unknown side effects. It is possible to reference a volatile object by using a non-volatile value but the resulting behavior is undefined. According to C99 Section 6.7.3 Type qualifiers Paragraph 5:
If an attempt is made to refer to an object defined with a volatile-qualified type through use of an lvalue with non-volatile-qualified type, the behavior is undefined.
This also applies to objects that behave as if they were defined with qualified types such as an object at a memory-mapped input/output address.
Non-Compliant Code Example
In this example, a volatile object is accessed through
int main(void) { static volatile int **ipp; static int *ip; static volatile int i = 0;; printf("i = %d.\n", i); ipp = &ip; // constraint violation *ipp = &i; // valid if (*ip != 0) { // valid // i had been changed } }
The first assignment is unsafe because it would allow the following valid code to reference the value of the volatile object i
through a non-volatile qualified reference.
Implementation Specific Details
This example compiles without warning on Microsoft Visual C++ .NET (2003) and on MS Visual Studio 2005. Version 3.2.2 of the gcc compiler generates a warning but compiles.
Compliant Solution
In this compliant solution the int * ip
is declared as volatile.
int main(void) { static volatile int **ipp; static volatile int *ip; static volatile int i = 0;; printf("i = %d.\n", i); ipp = &ip; // constraint violation *ipp = &i; // valid if (*ip != 0) { // valid /* i has changed */ }
Priority: P2 Level: L3
Accessing a volatile object through a non-volatile reference results in undefined behavior.
Component |
Value |
---|---|
Severity |
1 (low) |
Likelihood |
1 (unlikely) |
Remediation cost |
2 (medium) |
References
- ISO/IEC 9899-1999 Section 6.7.3 Type qualifiers, Section 6.5.16.1 Simple assignment